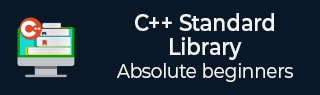
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Stack::empty() 函数
C++ 的std::stack::empty()函数检查堆栈是否为空(没有元素)。大小为零的堆栈被认为是空堆栈。
此函数不接受任何参数,它是一个const成员函数。“const”关键字表示该函数不修改堆栈。如果堆栈为空,则返回布尔值true,否则返回false。
当堆栈为空时,尝试从中弹出元素将导致错误,因为没有元素可删除。因此,空堆栈通常用作涉及推送元素的操作的起点。
语法
以下是std::stack::empty()函数的语法:
bool stack_name.empty() const;
参数
此方法不接受任何参数。
返回值
如果堆栈为空,则返回 true,否则返回 false。
示例 1
以下示例显示了 std::stack::empty() 函数的用法。首先,我们尝试创建一个没有元素的堆栈s,并使用 empty() 函数验证它。然后,我们将元素 '1' 插入/压入堆栈,并使用 empty() 函数确定此堆栈是否为空。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s; if (s.empty()){ cout << "Stack is empty." << endl;} else { cout << "Stack is not empty." << endl;} s.emplace(1); if (!s.empty()){ cout << "Stack is not empty." << endl;} else { cout << "Stack is empty." << endl;} return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Stack is empty. Stack is not empty.
示例 2
在这里,我们尝试按顺序将从 1 到 6 的数字插入堆栈。然后,我们使用 pop() 函数逐个删除元素,并计算元素的乘积,直到堆栈为空。
#include <iostream> #include <stack> using namespace std; int main() { stack<int> s; // initializing the variable Product with the value 1 int Product = 1; for (int i=1;i<=6;i++) s.push(i); while (!s.empty()){ // multiplying the topmost element of the stack with Product Product = Product * s.top(); s.pop(); } cout << "The Product of elements is: " << Product << '\n'; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
The Product of elements is: 720
示例 3
在以下示例中,我们创建一个空堆栈s,然后将值 '10'、'20'、'30'、'3' 和 '0' 压入其中。然后,我们使用 empty() 函数检查堆栈是否为空,并根据结果打印结果。
#include <iostream> #include <stack> using namespace std; int main() { stack<int> s; s.push(10); s.push(20); s.push(30); s.push(3); s.push(0); cout<<"stack s is empty: \n"<<s.empty()<<"\n"; //empty stack stack<int> s1; cout<<"stack s1 is empty: \n"<<s1.empty(); }
输出
以下是上述代码的输出:
stack s is empty: 0 stack s1 is empty: 1
广告