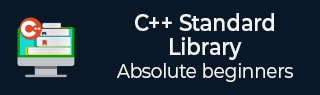
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Stack::operator>= 函数
C++ 函数std::stack::operator>=用于比较两个栈。它检查一个栈中的元素是否大于或等于另一个栈中的元素。如果栈的大小不同,此函数可能会返回未定义的结果。如果第一个栈的元素大于或等于第二个栈的对应元素,则返回true;否则返回false。
语法
以下是std::stack::operator>=函数的语法:
bool operator>=(const stack <Type, Container>& left, const stack <Type, Container>& right);
参数
- left - 类型为stack的对象。
- right - 类型为stack的对象。
返回值
如果第一个栈大于或等于第二个栈,则返回true;否则返回false。
示例1
让我们来看下面的例子,我们将比较两个栈x和y的大小。
#include <iostream> #include <stack> int main() { std::stack<int> x, y; x.push(11); x.push(22); x.push(33); y.push(44); y.push(55); if (x.size() >= y.size()) { std::cout << "True"; } else { std::cout << "False"; } return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
True
示例2
考虑下面的例子,我们将检查栈的大小是否大于或等于指定的值。
#include <iostream> #include <stack> int main(){ std::stack<int> x; x.push(11); x.push(22); int value = 4; if (x.size() >= value) { std::cout << "True, Stack size is greaterthan or equal to" <<value<<"."; } else { std::cout << "False, Stack size is Not greaterthan or equal to " <<value<<"."; } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
False, Stack size is Not greaterthan or equal to 4.
示例3
在下面的例子中,我们将观察std::stack::operator>=函数的用法。
#include <iostream> #include <stack> using namespace std; int main(void){ stack<int> s1; stack<int> s2; for (int i = 0; i < 5; ++i) { s1.push(i + 1); s2.push(i + 1); } s1.push(6); if (s1 >= s2) cout << "Stack s1 is greater than or equal to s2." << endl; s2.push(7); if (!(s1 >= s2)) cout << "Stack s1 is not greater than or equal to s2." << endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Stack s1 is greater than or equal to s2. Stack s1 is not greater than or equal to s2.
广告