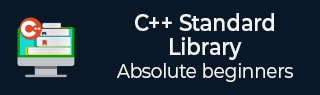
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Stack::operator!= 函数
C++ 函数std::stack::operator!=是一个二元运算符,用于测试两个栈是否不相等。
此函数不接受任何参数,并且是一个const成员函数。“const”关键字表示该函数不会修改栈。它是operator==函数的补集(相反)运算符。如果两个栈的元素和大小不相等,则返回布尔值true,否则返回false。
二元运算符采用两个操作数来执行特定的运算,例如加法、减法、乘法、除法或比较。它由符号或关键字表示,例如+、-、*、/和!=。
语法
以下是std::stack::operator!=函数的语法:
bool stack1 != stack2
参数
- stack1 - 第一个栈。
- stack2 - 第二个栈。
返回值
如果两个栈不相等,则返回true,否则返回false。
示例 1
以下示例演示了通过比较包含相同元素且大小相同的两个栈来使用std::stack::operator!=函数。
首先,我们创建两个栈's1'和's2',并将元素'1-5'插入到两个栈中。然后我们使用operator!=函数比较它们。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s1; stack<int> s2; for (int i = 0; i < 5; ++i) { s1.push(i + 1); s2.push(i + 1); } if (s1 != s2){ cout << "Both stacks are not equal." << endl; }else { cout << "Both stacks are equal." << endl; } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Both stacks are equal.
示例 2
在这里,我们比较两个包含不同元素但大小相同的非空栈。
最初,我们创建两个栈's1'和's2',并将元素'1'、'2'和'3'插入到's1'中,并将'3'、'2'和'1'插入到's2'中。然后我们使用operator!=函数比较它们。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s1; stack<int> s2; s1.push(1); s1.push(2); s1.push(3); s2.push(3); s2.push(2); s2.push(1); if (s1 != s2) { cout << "Both stacks are not equal." << endl; }else { cout << "Both stacks are equal." << endl; } }
输出
如果我们运行上面的代码,它将生成以下输出:
Both stacks are not equal.
示例 3
现在,我们比较两个包含不同元素且大小不同的非空栈。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s1; stack<int> s2; s1.push(1); s1.push(2); s2.push(1); if (s1 != s2) { cout << "Both stacks are not equal." << endl; }else { cout << "Both stacks are equal." << endl; } }
输出
以下是上述代码的输出:
Both stacks are not equal.
示例 4
在下面的示例中,我们尝试使用operator!=函数比较两个空栈's1'和's2':
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s1; stack<int> s2; if (s1 != s2) { cout << "Both stacks are not equal." << endl; }else { cout << "Both stacks are equal." << endl; } }
输出
上述代码的输出如下:
Both stacks are equal.
广告