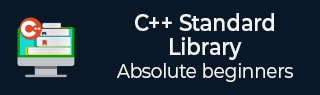
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Stack::pop() 函数
C++ 函数std::stack::pop() 从堆栈顶部移除一个元素,有效地将其大小减一。
pop() 函数不接受任何参数并返回 void。此外,重要的是要确保不能在空堆栈上调用 pop() 函数,如果尝试这样做,它将抛出异常。
pop() 函数作为 LIFO(后进先出)顺序中的删除操作。这意味着最后插入堆栈的元素是第一个被移除的元素,而第一个插入堆栈的元素是最后一个被移除的元素。
pop() 函数的复杂度为 O(1),这意味着无论堆栈的大小如何,它的执行时间都是恒定的。
语法
以下是 std::stack::pop() 函数的语法:
void stack_name.pop();
参数
此函数不接受任何参数。
返回值
此函数没有返回值。
示例 1
以下示例显示了 std::stack::pop() 函数的使用方法。这里我们创建一个空的整数堆栈,并使用 emplace() 函数向其中插入五个元素。然后,我们使用 top() 和 pop() 函数分别以 LIFO 顺序检索和移除元素,直到堆栈变空。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s; for (int i = 0; i < 5; ++i) s.emplace(i + 1); while (!s.empty()) { cout << s.top() << endl; s.pop(); } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
5 4 3 2 1
示例 2
在这里,我们尝试按 1 到 6 的数字顺序将元素插入堆栈。然后,我们使用 pop() 函数逐一移除元素并计算元素的乘积,直到堆栈变空。最后,我们使用 size() 函数在 while 循环的每次迭代中检索堆栈的大小,并在堆栈变空后打印乘积。
#include <iostream> #include <stack> using namespace std; int main(){ stack<int> stck; int Product = 1; stck.push(1); stck.push(2); stck.push(3); stck.push(4); stck.push(5); stck.push(6); while (!stck.empty()){ Product = Product * stck.top(); cout<<"\nsize of stack is: "<<stck.size(); stck.pop(); } cout<<"\nThe product of the elements is: "<<Product; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
size of stack is: 6 size of stack is: 5 size of stack is: 4 size of stack is: 3 size of stack is: 2 size of stack is: 1 The product of the elements is: 720
示例 3
现在,我们使用名称 s1 创建一个空的字符数据类型的堆栈,并按顺序将字符 't'、'r'、'u'、'c' 和 'k' 插入堆栈。然后,我们分别使用 top() 和 pop() 函数从堆栈中检索和移除元素,直到堆栈变空。
#include <iostream> #include <stack> using namespace std; int main(void) { stack <char> s1; s1.push('t'); s1.push('r'); s1.push('u'); s1.push('c'); s1.push('k'); cout << "Contents of stack s1" << endl; while (!s1.empty()) { cout << s1.top() << endl; s1.pop(); } return 0; }
输出
上述代码的输出如下:
Contents of stack s1 k c u r t
示例 4
在下面的示例中,我们创建一个浮点数数据类型的堆栈,然后向其中插入 6 个元素。然后我们移除前 3 个元素,然后检索并移除剩余元素,直到堆栈变空。
#include <iostream> #include <stack> using namespace std; int main() { stack<float> s; s.push(87.45f); s.push(43.76f); s.push(365.43f); s.push(32.67f); s.push(79.6f); s.push(645.76f); s.pop(); s.pop(); s.pop(); while (!s.empty()) { cout << s.top() << endl; s.pop(); } return 0; }
输出
以下是上述代码的输出:
365.43 43.76 87.45
广告