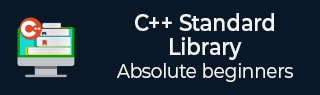
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Stack::swap() 函数
C++ 函数std::stack::swap()交换两个栈的所有元素。为了使用此函数,两个栈的数据类型必须相同,但大小可以不同(如有必要可以修改)。
swap() 函数是用于处理 C++ 中栈数据结构的简单而高效的工具。它可以用于反转栈中元素的顺序、清除栈的内容以及对栈中的元素进行排序。
语法
以下是 std::stack::swap() 函数的语法:
void swap (stack& x) noexcept;
参数
x - 另一个相同类型的栈对象。
返回值
此方法没有返回值。
示例 1
以下示例演示了使用不同大小的 std::stack::swap() 函数。
首先,我们创建了两个栈 's1' 和 's2'。然后,我们使用 push() 函数将值 '1 - 6' 插入 's1',并将值 '101 - 103' 插入 's2'。之后,我们使用 swap() 函数交换 's1' 和 's2' 的元素。最后,我们使用 top() 和 pop() 函数检索并移除两个栈中的元素。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s1; stack<int> s2; for (int i = 0; i < 5; ++i) s1.push(i + 1); for (int i = 0; i < 3; ++i) s2.push(100 + i); s1.swap(s2); cout << "Contents of stack s1 after swap operation" << endl; while (!s1.empty()) { cout << s1.top() << endl; s1.pop(); } cout << endl; cout << "Contents of stack s2 after swap operation" << endl; while (!s2.empty()) { cout << s2.top() << endl; s2.pop(); } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Contents of stack s1 after swap operation 102 101 100 Contents of stack s2 after swap operation 5 4 3 2 1
示例 2
现在我们尝试交换大小相同的两个栈 'stack1' 和 'stack2':
#include <iostream> #include <stack> int main() { std::stack<int> stack1; std::stack<int> stack2; stack1.push(1); stack1.push(2); stack1.push(3); stack2.push(4); stack2.push(5); stack2.push(6); // Swap the two stacks stack1.swap(stack2); std::cout << "Stack 1 after swap:" << std::endl; while (!stack1.empty()) { std::cout << stack1.top() << std::endl; stack1.pop(); } std::cout << "Stack 2 after swap:" << std::endl; while (!stack2.empty()) { std::cout << stack2.top() << std::endl; stack2.pop(); } return 0; }
输出
上述代码的输出如下:
Stack 1 after swap: 6 5 4 Stack 2 after swap: 3 2 1
示例 3
在这里,我们使用 char 数据类型上的 swap() 函数来交换两个栈 's1' 和 's2' 的元素:
#include <iostream> #include <stack> using namespace std; int main(void) { stack<char> s1; stack<char> s2; s1.push('c'); s1.push('a'); s1.push('r'); s2.push('b'); s2.push('i'); s2.push('k'); s2.push('e'); s1.swap(s2); cout << "Contents of stack s1 after swap operation" << endl; while (!s1.empty()) { cout << s1.top() << endl; s1.pop(); } cout << endl; cout << "Contents of stack s2 after swap operation" << endl; while (!s2.empty()) { cout << s2.top() << endl; s2.pop(); } return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Contents of stack s1 after swap operation e k i b Contents of stack s2 after swap operation r a c
示例 4
现在,我们尝试将包含 2 个元素的栈 'stack1' 与空栈 'stack2' 交换:
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> stack1; stack<int> stack2; stack1.push(1); stack1.push(2); stack1.swap(stack2); cout << "Contents of stack1 after swap operation:" << endl; while (!stack1.empty()) { cout << stack1.top() << endl; stack1.pop(); } cout << endl; cout << "Contents of stack2 after swap operation:" << endl; while (!stack2.empty()) { cout << stack2.top() << endl; stack2.pop(); } return 0; }
输出
以下是上述代码的输出:
Contents of stack1 after swap operation: Contents of stack2 after swap operation: 2 1
广告