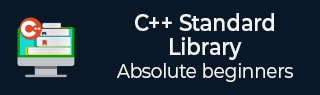
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Stack::top() 函数
C++ 函数std::stack::top()返回栈顶元素。在栈中,栈顶元素是最后插入或最近插入的元素,因为栈是后进先出 (LIFO) 容器。我们需要执行弹出操作才能连续从栈中获取栈顶元素。
top() 函数不接受任何参数。此外,重要的是要确保不能在空栈上调用 top() 函数,如果尝试这样做,它将抛出异常。
语法
以下是 std::stack::top() 函数的语法:
stack_name.top()
参数
此函数不接受任何参数。
返回值
返回栈顶元素。
示例 1
以下示例显示了 std::stack::top() 函数的用法。在这里,我们创建一个空的整数栈,并使用 emplace() 函数向其中插入五个元素。然后,我们使用 top() 和 pop() 函数分别以 LIFO 顺序检索和移除元素,直到栈变空。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s; for (int i = 0; i < 5; ++i) s.emplace(i + 1); while (!s.empty()) { cout << s.top() << endl; s.pop(); } return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
5 4 3 2 1
示例 2
在下面的示例中,我们创建一个栈 's' 并向栈中插入三个整数值。然后,我们使用 top() 函数检索栈顶元素:
#include <iostream> #include <stack> using namespace std; int main(){ stack<int> s; s.push(7); s.push(32); s.push(95); cout << "The top element in s1 is:" << endl; cout << s.top(); return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
The top element in s1 is: 95
示例 3
在这里,我们创建一个整数栈 's' 并向栈中插入两个值 (3 和 5)。然后,我们使用 top() 函数将栈顶元素修改为 '7'。
#include <iostream> #include <stack> using namespace std; int main(){ stack<int> s; s.push(3); s.push(5); s.top() = 7; cout << "Top element of the stack: " << s.top() << endl; return 0; }
输出
以下是上述代码的输出:
Top element of the stack: 7
示例 4
现在,我们创建一个名为 s1 的空字符数据类型栈,并按顺序向栈中插入字符 't'、'r'、'u'、'c' 和 'k'。然后,我们使用 top() 函数检索栈顶元素:
#include <iostream> #include <stack> using namespace std; int main(void) { stack <char> s1; s1.push('t'); s1.push('r'); s1.push('u'); s1.push('c'); s1.push('k'); cout << "The top element in s1 is:" << endl; cout << s1.top(); return 0; }
输出
上述代码的输出如下:
The top element in s1 is: k
广告