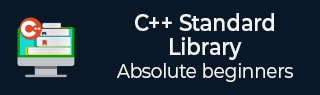
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::compare() 函数
C++ 的std::string::compare()函数用于比较两个字符串。它提供了一种按字典顺序比较字符串对象内容与另一个字符串或子字符串的方法。它返回一个整数,如下所示:
0:字符串相等
<0:调用字符串小于参数字符串。
>0:调用字符串大于参数字符串。
此函数可用于比较整个字符串或指定范围的字符。
语法
以下是 std::string::compare() 函数的语法。
int compare (const string& str) const noexcept; or int compare (size_t pos, size_t len, const string& str) const; int compare (size_t pos, size_t len, const string& str, size_t subpos, size_t sublen) const; or int compare (const char* s) const; int compare (size_t pos, size_t len, const char* s) const; or int compare (size_t pos, size_t len, const char* s, size_t n) const;
参数
- str − 指示另一个字符串对象。
- len − 指示比较字符串的长度。
- pos − 指示对应字符串中第一个字符的位置。
- subpos, sublen − 与上面的 pos 和 len 相同。
- n − 指示要比较的字符数。
- s − 指示指向字符数组的指针。
返回值
它返回一个带符号整数,指示字符串之间的关系。
异常
如果抛出异常,则字符串不会发生任何更改。
示例 1
以下是一个使用 C++ 演示 string::compare 的基本示例。
#include <iostream> #include <string> using namespace std; int main() { string X1 = "apple"; string X2 = "banana"; int result = X1.compare(X2); if (result == 0) { cout << " Both are equal " << endl; } else if (result < 0) { cout << X1 << " is less than " << X2 << endl; } else if (result > 0) { cout << X1 << " is greater than " << X2 << endl; } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
apple is less than banana
示例 2
在这个例子中,我们比较一个子字符串与另一个字符串。所以它将输出结果打印为相等。
#include <iostream> #include <string> using namespace std; int main() { string X1 = "Tutorialspoint"; string X2 = "point"; int result = X1.compare(9, 5, X2); if (result == 0) { cout << " Both are equal " << endl; } else if (result < 0) { cout << X1.substr(6, 5) << " is less than " << X2 << endl; } else if (result > 0) { cout << X1.substr(6, 5) << " is greater than " << X2 << endl; } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出。
Both string are equal.
示例 3
以下是用 string::compare() 函数比较两个字符串子字符串的另一个示例。
#include <iostream> #include <string> using namespace std; int main() { string X1 = "hello world"; string X2 = "goodbye world"; int result = X1.compare(6, 5, X2, 8, 5); if (result == 0) { cout << " Both are equal " << endl; } else if (result < 0) { cout << X1.substr(6, 5) << " is less than " << X2.substr(8, 5) << endl; } else { cout << X1.substr(6, 5) << " is greater than " << X2.substr(8, 5) << endl; } return 0; }
输出
以下是上述代码的输出。
The substrings are equal.
示例 4
在这个例子中,我们将一个字符串与空字符串进行比较。
#include <iostream> #include <string> using namespace std; int main() { string X1 = "hello"; string X2 = ""; int result = X1.compare(X2); if (result == 0) { cout << " Both are equal " << endl; } else if (result < 0) { cout << X1 << " is lesser than " << X2 << endl; } else { cout << X1 << " is greater than " << X2 << endl; } return 0; }
输出
以下是上述代码的输出。
hello is greater than
string.htm
广告