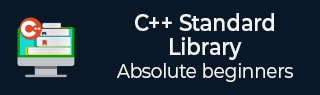
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::find_first_of() 函数
C++ 的std::string::find_first_of 用于在字符串中查找指定字符集中任何字符的第一次出现。它搜索在给定字符串或字符集中找到的任何字符的第一个实例,并返回该字符的位置。如果未找到任何字符,则返回 std::string::npos。
语法
以下是 std::string::find_first_of() 函数的语法。
size_t find_first_of (const string& str, size_t pos = 0) const noexcept; or size_t find_first_of (const char* s, size_t pos = 0) const; or size_t find_first_of (const char* s, size_t pos, size_t n) const; or size_t find_first_of (char c, size_t pos = 0) const noexcept;
参数
- str − 指示要搜索的字符的另一个字符串。
- pos − 指示要考虑在搜索中使用的字符串中第一个字符的位置。
- s − 指示指向字符数组的指针。
- n − 指示要搜索的字符值的个数。
- c − 指示要搜索的单个字符。
返回值
此函数返回搜索到的字符的位置。
示例 1
在下面的示例中,我们将考虑 find_first_of() 函数的基本用法。
#include <iostream> #include <string> #include <cstddef> int main() { std::string str("It replaces the vowels in this sentence by asterisks."); std::size_t found = str.find_first_of("aeiou"); while (found != std::string::npos) { str[found] = '*'; found = str.find_first_of("aeiou", found + 1); } std::cout << str << '\n'; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
It r*pl*c*s th* v*w*ls *n th*s s*nt*nc* by *st*r*sks.
示例 2
在下面的代码中,我们初始化了字符串 x,并使用 find_first_of() 函数查找字符串 = 'morning' 的第一次出现位置。
#include<iostream> #include<string> using namespace std; int main() { string x = "Good morning everyone!"; cout << "String contains = " << x << endl; cout << "String character = " << x.find_first_of("morning"); return 0; }
输出
如果我们运行上述代码,它将生成以下输出。
String contains = Good morning everyone! String character = 1
示例 3
在程序中,我们初始化了字符串 x 并将位置设置为 2。因此,它从第二个位置开始搜索 'morning' 的第一次出现。因此,在这里我们可以看到指定了开始搜索的位置。
#include<iostream> #include<string> using namespace std; int main() { string x = "Good morning everyone!"; cout << "String contains : " << x << '\n'; cout << "searching from second position and finding the first occurrence of the 'morning' = " << x.find_first_of("morning", 2); return 0; }
输出
以下是上述代码的输出。
String contains : Good morning everyone! searching from second position and finding the first occurrence of the 'morning' = 2
示例 4
在下面的示例中,我们初始化了字符串 x,并使用 find_first_of() 函数查找单个字符第一次出现的位置。
#include<iostream> #include<string> using namespace std; int main() { string x = "Tutorialspoint Company!"; cout << "String = " << x << '\n'; cout << "First occurrence position of 'u' character = " << x.find_first_of('u'); return 0; }
输出
以下是上述代码的输出。
String = Tutorialspoint Company! First occurrence position of 'u' character = 1
string.htm
广告