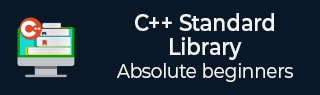
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::find_last_not_of() 函数
C++ 的std::string::find_last_not_of()函数用于查找字符串中最后一个不匹配指定集合中任何字符的字符的位置。它从结尾向开头搜索字符串,并返回最后一个不匹配提供的集合中任何字符的字符的位置。
如果字符串中的所有字符都是集合的一部分,则它返回std::string::npos。
语法
以下是std::string::find_last_not_of()函数的语法。
size_t find_last_not_of (const string& str, size_t pos = npos) const noexcept; or size_t find_last_not_of (const char* s, size_t pos = npos) const; or size_t find_last_not_of (const char* s, size_t pos, size_t n) const; or size_t find_last_not_of (char c, size_t pos = npos) const noexcept;
参数
- str − 指示另一个字符串对象。
- pos − 指示要考虑搜索的字符串中最后一个字符的位置。
- s − 指示指向字符数组的指针。
- n − 指示要搜索的字符数量。
- c − 指示要搜索的单个字符。
返回值
此函数返回最后一个不匹配字符的位置。
示例 1
以下是一个使用 C++ 中的 std::string::find_last_not_of 查找不在另一个字符串中的最后一个字符的示例。
#include <iostream> #include <string> using namespace std; int main() { string str = " Tutorialspoint10years@ "; size_t found = str.find_last_not_of("abcdefghijklmnopqrstuvwxyz "); if (found != string::npos) { cout << "The last non-alphabetic character is " << str[found]; cout << " at index " << found << '\n'; } return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
The last non-alphabetic character is @ at index 22
示例 2
以下是如何在字符串中查找最后一个非数字的另一个示例。
#include <iostream> #include <string> using namespace std; int main() { string str = "12345abcXYZ6789"; size_t pos = str.find_last_not_of("0123456789"); if (pos != string::npos) { cout << " '" << str[pos] << "' is at index " << pos << endl; } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出。
'Z' is at index 10
示例 3
在这个程序中,我们跳过字符串开头的空格。
#include <iostream> #include <string> using namespace std; int main() { string str = " Tutorialspoint10years@"; size_t pos = str.find_last_not_of(" \t\n"); if (pos != string::npos) { cout << "last non-whitespace character is '" << str[pos] << "' at index " << pos << endl; } return 0; }
输出
以下是上述代码的输出。
Last non-whitespace character is '@' at index 25
示例 4
在这个程序中,我们初始化了两个字符串以查找不在另一个字符串中的最后一个字符。
#include <iostream> #include <string> using namespace std; int main() { string str1 = "Tutorialspoint10years@"; string str2 = "10years@"; size_t pos = str1.find_last_not_of(str2); if (pos != string::npos) { cout << "last character in '" << str1 << "' not in '" << str2 << "' is '" << str1[pos] << "' at index " << pos << endl; } return 0; }
输出
以下是上述代码的输出。
last character in 'Tutorialspoint10years@' not in '10years@' is 't' at index 13
string.htm
广告