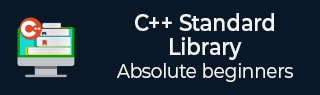
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::find_last_of() 函数
C++ 的 std::string::find_last_of() 函数用于在字符串中查找指定字符集中任何字符的最后一次出现位置。它从字符串的结尾向开头搜索,返回找到的与字符集中任何字符匹配的最后一个字符的索引。如果找不到字符集中的任何字符,则返回 std::string::npos。
语法
以下是 std::string::find_last_of() 函数的语法。
size_t find_last_of (const string& str, size_t pos = npos) const noexcept; or size_t find_last_of (const char* s, size_t pos = npos) const; or size_t find_last_of (const char* s, size_t pos, size_t n) const; or size_t find_last_of (char c, size_t pos = npos) const noexcept;
参数
- str − 表示另一个字符串对象。
- pos − 表示要在搜索中考虑的字符串中最后一个字符的位置。
- s − 表示指向字符数组的指针。
- n − 表示要搜索的字符数量。
- c − 表示要搜索的单个字符。
返回值
此函数返回最后一个字符的位置。
示例 1
以下是一个使用 C++ 查找 std::string::find_last_of 的示例。
#include<iostream> using namespace std; int main() { string str = "Tutorialspoint"; cout << "String:" << str << '\n'; cout << "last occurances = " << str.find_last_of("poi"); return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
String:Tutorialspoint last occurances = 11
示例 2
以下是在指定位置和长度的字符串中查找任何字符最后一次出现的另一个示例。
#include <iostream> #include <string> using namespace std; int main() { string str = "Tutorialspoint"; string chars = "al"; size_t pos = str.find_last_of(chars, 10); if (pos != string::npos) { cout << "Last occurrence is at index " << pos << endl; } else { cout << "not found in string" << endl; } return 0; }
输出
如果我们运行上述代码,它将生成以下输出。
Last occurrence is at index = 7
示例 3
在这个例子中,我们正在赋值一个空字符串和一个字符串字符。因此,因为它是一个空字符串,所以它不能搜索或查找其参数中的最后一个字符。
#include <iostream> #include <string> using namespace std; int main() { string str = ""; string chars = "al"; size_t pos = str.find_last_of(chars); if (pos != string::npos) { cout << "Last occurrence '" << chars << "' is at index " << pos << endl; } else { cout << " Empty String " << endl; } return 0; }
输出
以下是上述代码的输出。
Empty String
示例 4
在这个程序中,我们初始化了一个字符串并分配了一个不在字符串中的字符。
#include <iostream> #include <string> using namespace std; int main() { string str = "hello world"; string chars = "xyz"; size_t pos = str.find_last_of(chars); if (pos != string::npos) { cout << "Last occurrence '" << chars << "' is at index " << pos << endl; } else { cout << "Character not found in string (unmatched character)" << endl; } return 0; }
输出
以下是上述代码的输出。
Character not found in string (unmatched character)
string.htm
广告