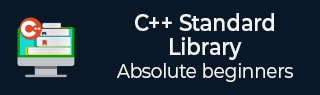
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::stof() 函数
C++ 的std::string::stof()函数用于将字符串转换为浮点数。此函数分析字符串,从字符串开头提取浮点值。如果转换成功,则返回浮点值;否则,将抛出invalid_argument异常。
语法
以下是 std::string::stof() 函数的语法。
float stof (const string& str, size_t* idx = 0); float stof (const wstring& str, size_t* idx = 0);
参数
此函数有两个参数,如下所述。
- str − 表示包含浮点数表示形式的字符串对象。
- idx − 指向 size_t 类型对象的指针,函数将其值设置为 str 中数值之后下一个字符的位置。
返回值
此函数返回与作为参数传递的字符串内容对应的浮点值。
示例 1
以下是用 C++ 使用 std::stof() 函数将字符串转换为浮点数的基本示例。
#include <string> #include <iostream> using namespace std; int main() { string temperature = " 36.6"; float bodyTemp = stof(temperature); cout << "Body temperature is " << bodyTemp << " degree Celsius" << endl; return 0; }
输出
如果运行上述代码,它将生成以下输出。
Body temperature is 36.6 degree Celsius
示例 2
以下示例传递包含有效浮点数的子字符串,跳过初始文本。
#include <string> #include <iostream> using namespace std; int main() { string mixedContent = "Height: 180cm"; size_t pos; float height = stof(mixedContent.substr(7), & pos); cout << "Height = " << height << " cm " << endl; return 0; }
输出
如果运行上述代码,它将生成以下输出。
Height = 180 cm
示例 3
在这个例子中,我们使用 C++ 中的 string::stof 转换单个字符串中的多个数字。
#include <string> #include <iostream> #include <vector> using namespace std; int main() { string data = "3.14 1.618 2.718"; vector < float > numbers; size_t pos = 0, prev_pos = 0; while ((pos = data.find(' ', prev_pos)) != string::npos) { numbers.push_back(std::stof(data.substr(prev_pos, pos - prev_pos), nullptr)); prev_pos = pos + 1; } numbers.push_back(stof(data.substr(prev_pos), nullptr)); for (float num: numbers) { cout << num << std::endl; } return 0; }
输出
以下是上述代码的输出。
3.14 1.618 2.718
示例 4
在这个例子中,我们给出了无效的输入,所以它会抛出 invalid_argument 异常,因为该字符串无法转换为浮点数。
#include <string> #include <iostream> using namespace std; int main() { string invalidNumber = "abc123"; try { float value = stof(invalidNumber); cout << "Value is: " << value << std::endl; } catch (const invalid_argument & e) { cout << "Invalid argument: " << e.what() << endl; } return 0; }
输出
以下是上述代码的输出。
Invalid argument: stof
string.htm
广告