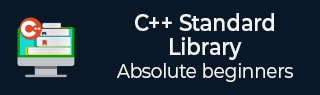
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::stoi() 函数
C++ 的std::string::stoi()函数用于将字符串转换为整数 (int)。此函数将字符串的初始字符分析为整数,并在遇到第一个非数字字符时停止。如果转换成功,则返回整数的值,否则抛出 invalid_argument 异常。
语法
以下是 std::string::stoi() 函数的语法。
int stoi (const string& str, size_t* idx = 0, int base = 10); int stoi (const wstring& str, size_t* idx = 0, int base = 10);
参数
此函数有两个参数,如下所述。
- str − 表示包含整数表示形式的字符串对象。
- idx − 表示指向 size_t 类型对象指针,函数将其值设置为 str 中数值之后下一个字符的位置。
- base − 表示数值基数,用于确定有效字符及其解释。
返回值
它返回已解析字符串的整数值。
示例 1
以下是使用 C++ 演示 string::stoi 的基本转换的基本示例。
#include <iostream> #include <string> using namespace std; int main() { string s = "42"; int num = stoi(s); cout << num << endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出。
42
示例 2
在此示例中,我们正在处理十六进制和二进制字符串以进行整数转换。
#include <iostream> #include <string> using namespace std; int main() { string hexStr = "2A"; string binStr = "101010"; int numHex = stoi(hexStr, nullptr, 16); int numBin = stoi(binStr, nullptr, 2); cout << numHex << ", " << numBin << endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出。
42, 42
示例 3
在以下示例中,我们正在从混合字符串中提取数字。
#include <iostream> #include <string> using namespace std; int main() { string mixedStr = "Year2024"; int year = stoi(mixedStr.substr(4)); cout << year << endl; return 0; }
输出
以下是以上代码的输出。
2024
示例 4
以下是一个演示 string:stoi() 函数的另一个示例。此处传递了字符串,但该字符串不包含任何有效数字,即数值。因此,它引发了 invalid_argument 异常。
#include <iostream> #include <string> using namespace std; int main() { string invalidStr = "Tutorialspoint"; try { int num = stoi(invalidStr); } catch (const std::invalid_argument & e) { cout << "Invalid input: " << e.what() << endl; } return 0; }
输出
以下是以上代码的输出。
Invalid input: stoi
string.htm
广告