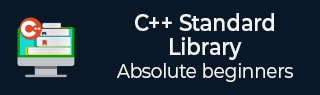
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::stoull() 函数
C++ 的std::string::stoull() 函数用于将字符串转换为无符号长长整型。它分析字符串以提取数值并忽略任何空格。它还支持可选参数以指定转换的基数,例如十进制、十六进制或八进制。如果由于无效输入导致转换失败,则会抛出 invalid_argument 异常。
语法
以下是 std::string::stoull() 函数的语法。
unsigned long long stoull (const string& str, size_t* idx = 0, int base = 10); unsigned long long stoull (const wstring& str, size_t* idx = 0, int base = 10);
参数
- str - 表示包含整数表示形式的字符串对象。
- idx - 表示指向 size_t 类型对象的指针,该函数将该指针的值设置为 str 中数值后下一个字符的位置。
- base - 表示确定有效字符及其解释的数字基数。
返回值
它将字符串作为值返回到无符号长长整型。
示例 1
以下是用 C++ 使用 std::stoull() 函数将字符串转换为无符号长长整型的基本示例。
#include <iostream> #include <string> using namespace std; int main() { string number = "12345678901234567890"; unsigned long long ull = stoull(number); cout << "The number = " << ull << endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出。
The number = 12345678901234567890
示例 2
以下是一个指定数字基数的示例,用于将十六进制字符串转换为其以 16 为基数的十进制等效值。
#include <iostream> #include <string> using namespace std; int main() { string hexNumber = "1A2B3C4D5E6F"; unsigned long long ull = stoull(hexNumber, nullptr, 16); cout << "The hexadecimal number " << hexNumber << " is " << ull << " in decimal." << endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出。
The hexadecimal number 1A2B3C4D5E6F is 28772997619311 in decimal.
示例 3
在此程序中,我们从包含文本和数字的字符串中提取第一个数字,并演示如何使用 idx 参数查找已解析数字后字符的位置。
#include <iostream> #include <string> using namespace std; int main() { string mixedString = "42ABC!@"; size_t idx = 0; while (idx < mixedString.size() && !isdigit(mixedString[idx])) { ++idx; } if (idx < mixedString.size()) { unsigned long long ull = stoull(mixedString.substr(idx), & idx, 10); cout << "Extracted number = " << ull << endl; idx += mixedString.find_first_of("0123456789"); cout << "Next character in string after number = " << mixedString[idx] << endl; } return 0; }
输出
以下是以上代码的输出。
Extracted number = 42 Next character in string after number = A
示例 4
在此程序中,我们转换一个不表示数字的字符串,捕获并处理可能抛出的潜在异常。
#include <iostream> #include <string> using namespace std; int main() { string number = "not a number"; try { unsigned long long ull = stoull(number); cout << "The number is: " << ull << std::endl; } catch (const invalid_argument & ia) { cerr << "Invalid argument = " << ia.what() << endl; } return 0; }
输出
以下是以上代码的输出。
Invalid argument: stoull
string.htm
广告