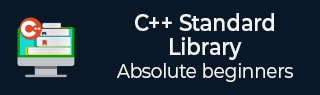
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ String::substr() 函数
C++ 的std::string::substr()函数用于从给定的字符串对象获取子字符串。它接受两个参数:子字符串的起始位置和子字符串的长度。此函数返回一个新的字符串对象,其中包含原始字符串的指定部分。
如果长度参数放置错误,则默认为从起始位置提取到字符串末尾的子字符串。
语法
以下是 std::string::substr() 函数的语法。
string substr (size_t pos = 0, size_t len = npos) const;
参数
- pos - 指示要复制的第一个字符的位置。
- len - 指示子字符串中包含的字符数。
返回值
此函数返回一个字符串对象。
示例 1
以下是使用 C++ 中的 std::substr() 函数创建从索引 0 开始并以索引 10 结束的子字符串的基本程序。
#include <iostream> #include <string> using namespace std; int main() { string str = " Tutorialspoint "; string sub = str.substr(0, 10); cout << sub << endl; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Tutorials
示例 2
在此示例中,substr 仅使用起始位置调用,因此它会从该位置创建到字符串末尾的子字符串。
#include <iostream> #include <string> using namespace std; int main() { string str = " Tutorialspoint "; string sub = str.substr(10); cout << sub << endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出。
point
示例 3
以下是另一个创建子字符串位置大于字符串长度导致 out_of_range 异常的示例。
#include <iostream> #include <string> using namespace std; int main() { string str = " Tutorialspoint "; try { string sub = str.substr(50); } catch (const std::out_of_range & e) { cout << "Exception: " << e.what() << endl; } return 0; }
输出
以下是上述代码的输出。
Exception: basic_string::substr: __pos (which is 50) > this->size() (which is 16)
示例 4
在此示例中,我们使用等于字符串长度的起始位置调用子字符串,它返回一个空字符串。
#include <iostream> #include <string> using namespace std; int main() { string str = "Tutorialspoint"; string sub = str.substr(str.length()); cout << "Empty string = '" << sub << "'" << endl; return 0; }
输出
以下是上述代码的输出。
Empty string = ''
string.htm
广告