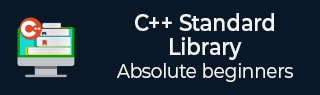
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Tuple::tie() 函数
C++ 的std::tuple::tie()函数用于帮助将多个变量绑定到元组的元素。本质上,它允许将元组值直接解包到指定的变量中。它主要用于您希望将元组的元素分解成单独的变量以进行进一步操作的场景。
tie() 函数可以与其他操作(如比较和排序)结合使用。
语法
以下是 std::tuple::tie() 函数的语法。
tie( Types&... args ) noexcept;
参数
- args - 它包含构造的元组应包含的元素列表。
示例
让我们看下面的例子,我们将使用 tie() 和 make_tuple() 交换两个变量的值。
#include <iostream> #include <tuple> using namespace std; int main() { int x = 11, y = 111; tie(x, y) = make_tuple(y, x); cout << "x: " << x << ", y: " << y << endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
x: 111, y: 11
示例
考虑另一种情况,我们将使用 tie() 函数将元组数据解包到单独的变量中。
#include <iostream> #include <tuple> using namespace std; int main() { tuple<float, string> data = make_tuple(0.01, "Welcome"); float x; string y; tie(x,y) = data; cout << "x: " << x << ", y: " << y <<endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
x: 0.01, y: Welcome
示例
在下面的示例中,元组数据的第二个元素仅解包到变量中,而其他元素被忽略。
#include <iostream> #include <tuple> using namespace std; int main() { tuple<int, string> data = make_tuple(42, "TutorialsPoint"); string x; tie(ignore, x) = data; cout << "x: " << x << endl; return 0; }
输出
以下是上述代码的输出:
x: TutorialsPoint
广告