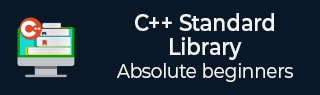
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Tuple::tuple_cat() 函数
C++ 的std::tuple::tuple_cat()函数用于将多个元组连接成单个元组。它接受任意数量的元组作为参数,并返回一个包含所有输入元组元素的新元组。此函数允许元组的组合,有助于合并或追加元组等操作。
语法
以下是 std::tuple::tuple_cat() 函数的语法。
tuple_cat (Tuples&&... tpls);
参数
- tpls − 它是一个元组对象的列表,这些元组可以是不同类型的。
返回值
此函数返回一个适当类型的元组对象以容纳参数。
示例
在下面的示例中,我们将组合两个不同类型的元组。
#include <iostream> #include <tuple> int main() { std::tuple<int, double> x(1, 0.04); std::tuple<char> y('A'); auto a = std::tuple_cat(x, y); std::cout << std::get<0>(a) << "," << std::get<1>(a)<< "," << std::get<2>(a) <<std::endl; return 0; }
输出
如果运行上述代码,它将生成以下输出:
1,0.04,A
示例
考虑另一种情况,我们将多个元组组合成单个元组。
#include <iostream> #include <tuple> int main() { std::tuple<double> x(0.01); std::tuple<std::string> y("Welcome"); std::tuple<bool, int> z(false, 1); auto a = std::tuple_cat(x, y, z); std::cout << std::get<0>(a) << ", " << std::get<1>(a) << ", " << std::get<2>(a) << ", " << std::get<3>(a) <<std::endl; return 0; }
输出
上述代码的输出如下:
0.01, Welcome, 0, 1
示例
让我们来看下面的示例,我们将尝试组合空元组并观察输出。
#include <iostream> #include <tuple> int main() { std::tuple<> x; std::tuple<> y; auto a = std::tuple_cat(x, y); std::cout << "Size of the combined tuple: " << std::tuple_size<decltype(a)>::value << std::endl; return 0; }
输出
上述代码的输出如下:
Size of the combined tuple: 0
示例
以下示例将结合使用 make_tuple() 函数和 tuple_cat() 函数。
#include <iostream> #include <tuple> int main() { auto x = std::make_tuple(1, 0.01); auto y = std::make_tuple("TutorialsPoint", 'R'); auto a = std::tuple_cat(x, y); std::cout << std::get<0>(a) << ", " << std::get<1>(a) << ", " << std::get<2>(a) << ", " << std::get<3>(a) << std::endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
1, 0.01, TutorialsPoint, R
广告