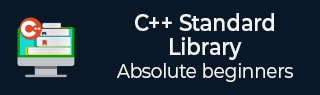
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Tuple::operator=() 函数
C++ 的std::tuple::operator=() 函数用于将一个元组的值赋给另一个相同类型的元组。它允许高效地复制元组内容,确保每个元素都分配到目标元组中的对应元素。
通过使用此函数,我们可以轻松地管理和操作元组。
语法
以下是 std::tuple::operator=() 函数的语法。
tuple& operator= (const tuple& tpl);tuple& operator= (tuple&& tpl) noexcept;
参数
- tpl - 它表示另一个具有相同数量元素的元组对象
返回值
此函数不返回任何值。
示例
让我们看下面的示例,我们将把一个元组的内容赋值给另一个元组。
#include <iostream> #include <tuple> int main() { std::tuple<std::string, double> x("Welcome", 0.01); std::tuple<std::string, double> y; y = x; std::cout << std::get<0>(y) << " " << std::get<1>(y) << " " <<std::endl; return 0; }
输出
让我们编译并运行以上程序,这将产生以下结果:
Welcome 0.01
示例
考虑另一种情况,我们将把一个元组的内容赋值给另一个数据类型不同的元组。
#include <iostream> #include <tuple> int main() { std::tuple<int, std::string> x(1, "TutorialsPoint"); std::tuple<double, const char*> y; std::get<0>(y) = static_cast<double>(std::get<0>(x)); std::get<1>(y) = std::get<1>(x).c_str(); std::cout << std::get<0>(y) << " " << std::get<1>(y) << std::endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
1 TutorialsPoint
示例
在下面的示例中,我们将使用 operator=() 函数将元组赋值给自己,并观察输出。
#include <iostream> #include <tuple> int main() { std::tuple<std::string, double> x("TP", 1.12); x = x; std::cout << std::get<0>(x) << " " << std::get<1>(x) << " " << std::endl; return 0; }
输出
以下是以上代码的输出:
TP 1.12
示例
以下是一个示例,我们将使用 operator=() 函数以及 tie。
#include <iostream> #include <tuple> int main() { int x, y, z; std::tuple<int, double, char> a(12, 0.01, 'c'); std::tie(x, y, z) = a; std::cout << "x: " << x << ", y: " << y << ", z: " << z << std::endl; return 0; }
输出
以上代码的输出如下:
x: 12, y: 0, z: 99
广告