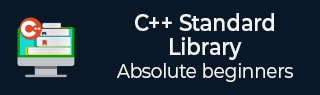
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_map::emplace() 函数
C++ 函数unordered_map::emplace()用于向容器插入新元素,并使容器大小增加一。只有当键不存在于容器中时,插入才会发生,这使得键是唯一的。
如果多次放置相同的键,地图只存储第一个元素,因为地图是一个不存储多个相同键值的容器。
语法
以下是 unordered_map::emplace() 函数的语法:
pair<iterator, bool> emplace( Args&&... args);
参数
args − 表示要转发到元素构造函数的参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
返回一个pair,包含一个bool值(指示是否发生了插入)和一个指向新插入元素的迭代器。
示例 1
让我们看看 unordered_map::emplace() 函数的基本用法。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<char, int> um; um.emplace('a', 1); um.emplace('b', 2); um.emplace('c', 3); um.emplace('d', 4); um.emplace('e', 5); cout << "Unordered map contains following elements" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Unordered map contains following elements e = 5 d = 4 c = 3 b = 2 a = 1
示例 2
在下面的示例中,我们创建了一个 unordered_map,然后我们将使用 emplace() 函数添加另外两个键值对。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<string, int> um = {{"Aman", 490},{"Vivek", 485},{"Akash", 500},{"Sonam", 450}}; cout << "Unordered map contains following elements before" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; cout<<"after use of the emplace function \n"; um.emplace("Sarika", 440); um.emplace("Satya", 460); cout << "Unordered map contains following elements" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Unordered map contains following elements before Sonam = 450 Akash = 500 Vivek = 485 Aman = 490 after use of the emplace function Unordered map contains following elements Sarika = 440 Satya = 460 Sonam = 450 Akash = 500 Vivek = 485 Aman = 490
示例 3
考虑下面的示例,我们尝试使用 emplace() 函数更改现有键的值,如下所示:
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<string, int> um = {{"Aman", 490},{"Vivek", 485},{"Akash", 500},{"Sonam", 450}}; cout << "Unordered map contains following elements before usages of emplace" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; um.emplace("Aman", 440); um.emplace("Sonam", 460); cout << "Unordered map contains same elements after the usages of emplace() function" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
以下是上述代码的输出:
Unordered map contains following elements before Sonam = 450 Akash = 500 Vivek = 485 Aman = 490 Unordered map contains same elements after the usages of emplace() function Sonam = 450 Akash = 500 Vivek = 485 Aman = 490
广告