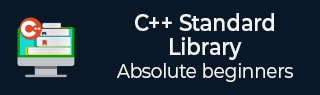
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::emplace_hint() 函数
C++ 的unordered_multimap::emplace_hint()函数用于使用提示或位置在unordered_multimap中插入新元素。该位置仅作为提示;它不决定插入的位置,并且通过插入新元素将容器大小增加一。此函数类似于emplace()函数。
语法
以下是unordered_map::emplace_hint()函数的语法。
iterator emplace_hint ( const_iterator position, Args&&... args );
参数
- position − 插入元素的位置提示。
- args − 它指定转发到构造新元素的参数。
返回值
此函数返回指向新插入元素的迭代器。
示例 1
在下面的示例中,让我们看看emplace_hint()函数的使用。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm = { {'b', 2}, {'c', 3}, {'d', 4}, }; umm.emplace_hint(umm.begin(), 'b', 2); umm.emplace_hint(umm.end(), 'e', 3); cout << "Unordered multimap contains following elements" << endl; for (auto it = umm.begin(); it != umm.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Unordered multimap contains following elements e = 3 b = 2 b = 2 c = 3 d = 4
示例 2
考虑下面的示例,我们将在此示例中在容器的起始位置和结束位置添加键值对。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<string, int> umm = {{"Aman", 490},{"Vivek", 485},{"Akash", 500},{"Sonam", 450}}; cout << "multimap contains following elements before" << endl; for (auto it = umm.begin(); it != umm.end(); ++it) cout << it->first << " = " << it->second << endl; cout<<"after use of the emplace_hint() function \n"; umm.emplace_hint(umm.begin(), "Vivek", 440); umm.emplace_hint(umm.end(), "Aman", 460); umm.emplace_hint(umm.end(), "Akash", 460); cout << "multimap contains following elements" << endl; for (auto it = umm.begin(); it != umm.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
以下是上述代码的输出:
multimap contains following elements before Akash = 500 Vivek = 485 Sonam = 450 Aman = 490 after use of the emplace_hint() function multimap contains following elements Aman = 460 Aman = 490 Sonam = 450 Vivek = 440 Vivek = 485 Akash = 460 Akash = 500
示例 3
让我们看下面的例子,我们将使用emplace_hint()并将键值对存储在随机顺序中。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_multimap<int, string> Ummap; auto it = Ummap.emplace_hint(Ummap.begin(), 1, "Jun"); it = Ummap.emplace_hint(it, 1, "January"); it = Ummap.emplace_hint(it, 2, "February"); it = Ummap.emplace_hint(it, 3, "March"); Ummap.emplace_hint(it, 3, "April"); Ummap.emplace_hint(it, 2, "May"); cout << "The unordered_multimap contains following element : \n"; cout << "KEY\tELEMENT"<<endl; for (auto itr = Ummap.begin(); itr != Ummap.end(); itr++) cout << itr->first << "\t"<< itr->second << endl; return 0; }
输出
上述代码的输出如下:
The unordered_multimap contains following element : KEY ELEMENT 3 March 3 April 2 May 2 February 1 Jun 1 January
广告