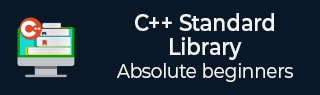
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_set::empty() 函数
C++ 的std::unordered_set::empty() 函数用于返回一个布尔值,指示 unordered_set 容器是否为空。
如果 unordered_set 为空,则 begin() 等于 end(),并且 empty() 函数返回 true;否则,它返回 false。
语法
以下是 std::unordered_set::empty 的语法。
bool empty() const noexcept;
参数
此函数不接受任何参数。
返回值
如果容器大小为 0,则此函数返回 true,否则返回 false。
示例 1
考虑以下示例,我们将演示 unordered_set::empty() 函数的使用。
#include <iostream> #include <string> #include <unordered_set> int main () { std::unordered_set<std::string> first = {"sairam","krishna","mammahe"}; std::unordered_set<std::string> second; std::cout << "first " << (first.empty() ? "is empty" : "is not empty" ) << std::endl; std::cout << "second " << (second.empty() ? "is empty" : "is not empty" ) << std::endl; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
first is not empty second is empty
示例 2
让我们看一下下面的示例,我们将使用 empty() 函数检查 unordered_set 是否为空。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<string> uSet; if(uSet.empty()==1){ cout<<boolalpha; cout<<"Is unordered_set empty? "<<uSet.empty()<<endl; } else{ cout<<"unordered_set contains following elements: "<<endl; for(auto it: uSet) cout<<it<<endl; } return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Is unordered_set empty? true
示例 3
在以下示例中,我们将使用 empty() 函数检查 unordered_set 是否为空,如果它包含元素,则显示 unordered_set 的内容。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<int> uSet{10, 20, 30, 40, 50}; if(uSet.empty()==1){ cout<<boolalpha; cout<<"Is unordered_set empty? "<<uSet.empty()<<endl; } else{ cout<<"unordered_set contains following elements: "<<endl; for(auto it: uSet) cout<<it<<endl; } return 0; }
输出
以下是上述代码的输出:
unordered_set contains following elements: 50 40 30 20 10
示例 4
以下是 empty() 函数用法的另一个示例,并在布尔值中显示结果。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<string> uSet; cout<<boolalpha; cout<<"boolean value before the insertion of elements: "<<endl; cout<<"Is unordered_set empty? "<<uSet.empty()<<endl; uSet.insert("tutorix"); uSet.insert("tutorialspoint"); cout<<"boolean value after the insertion of elements: "<<endl; cout<<"Is unordered_set empty? "<<uSet.empty()<<endl; return 0; }
输出
上述代码的输出如下:
boolean value before the insertion of elements: Is unordered_set empty? true boolean value after the insertion of elements: Is unordered_set empty? false
广告