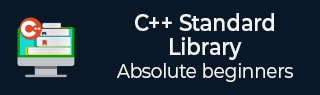
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_set::operators=() 函数
C++ 的std::unordered_set::operator=() 函数用于替换 unordered_set 容器的内容,或将一个 unordered_set 复制或移动到另一个 unordered_set 容器。
赋值运算符仅针对相同类型的对象定义;如果尝试对不同类型使用赋值运算符,则会导致编译错误。
此函数有 3 个多态变体:使用复制赋值运算符、移动赋值运算符和初始化列表(您可以在下面找到所有变体的语法)。
语法
以下是 std::operators=() 函数的语法。
unordered_set& operator= ( const unordered_set& ust ); or unordered_set& operator= ( unordered_set&& ust ); or unordered_set& operator= ( intitializer_list<value_type> il );
参数
- ust - 表示相同类型的 unordered_set 对象。
- il - 表示一个初始化列表对象。
返回值
此函数返回 (*this) unordered_set 容器。
示例 1
在以下示例中,我们将使用 unordered_set::operator=() 函数将当前 unordered_set 的元素复制到另一个 unordered_set 中。
#include <unordered_set> #include <iterator> #include <iostream> using namespace std; int main() { unordered_set<int> uSet, myUset; uSet = {1, 2, 3 ,4, 5}; //assigning the current unordered_set into another unordrer_set myUset = uSet; cout<<"Elements of myUset: "; for(auto it: myUset){ cout<<it<<" "; } cout<<endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Elements of myUset: 5 4 3 2 1
示例 2
考虑以下示例,我们将使用 std::unordered_map::operator=() 函数的移动版本。
#include <unordered_set> #include <iterator> #include <iostream> using namespace std; int main() { unordered_set<int> uSet, myUset; uSet = {1, 2, 3 ,4, 5}; //assigning the current unordered_set into another unordrer_set myUset = move(uSet); cout<<"Elements of myUset: "; for(auto it: myUset){ cout<<it<<" "; } cout<<endl; return 0; }
输出
以下是上述代码的输出:
Elements of myUset: 5 4 3 2 1
示例 3
让我们看看以下示例,我们将替换当前 unordered_set 中 ilist 的内容。
#include <iostream> #include <unordered_set> using namespace std; int main(void) { unordered_set<char> uSet = { 'a', 'b', 'c', 'd', 'e'}; auto ilist = {'A', 'B', 'C'}; cout<<"uSet content before the assignment operator= operation: "<<endl; for(auto&it : uSet){ cout<<it<<endl; } cout<<"The ilist content before the assignment operator = operation: "<<endl; for(auto & i : ilist){ cout<<i<<endl; } //using operator = function uSet = ilist; cout<<"uSet content after the assignment operator = operation: "<<endl; for(auto& it : uSet){ cout<<it<<endl; } return 0; }
输出
上述代码的输出如下:
uSet content before the assignment operator= operation: e d c b a The ilist content before the assignment operator = operation: A B C uSet content after the assignment operator = operation: C B A
广告