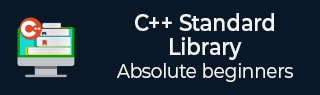
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_set::erase() 函数
C++ 的std::unordered_set::erase()函数用于从 unordered_set 容器中删除指定的元素,可以是单个元素或一系列元素。
此函数有 3 个多态变体:使用基于位置/迭代器的删除、基于范围的删除和基于键的删除(您可以在下面找到所有变体的语法)。
语法
以下是 std::unordered_set::erase() 函数的语法。
iterator erase ( const_iterator position ); or size_type erase ( const key_type& k ); or iterator erase ( const_iterator first, const_iterator last );
参数
- position - 指示指向要删除的单个元素的迭代器。
- k - 指示要删除的元素的值。
- (first, last) - 指定 unordered_set 中的一个范围。
返回值
该函数返回成员类型迭代器,它是一种前向迭代器类型。
示例 1
考虑以下示例,我们将演示 unordered_set::erase() 函数的使用。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<string> uSet = {"USA","Canada","France","UK","Japan","Germany","Italy"}; uSet.erase ( uSet.begin() ); uSet.erase ( "France" ); uSet.erase ( uSet.find("Japan"), uSet.end() ); cout << "uSet contains:"; for ( const string& x: uSet ) cout << " " << x; cout << endl; return 0; }
输出
让我们编译并运行以上程序,这将产生以下结果 -
uSet contains: Germany
示例 2
让我们看看下面的例子,我们将使用 erase() 函数从容器中删除奇数元素。
#include <unordered_set> #include <iostream> using namespace std; int main() { unordered_set<int> uSet = {10, 11, 12, 15, 17, 18}; cout << "After erasing all odd numbers: "; for (auto it = uSet.begin(); it != uSet.end();) { if (*it % 2 != 0) it = uSet.erase(it); else ++it; } cout<<"{ "; for(auto elem: uSet){ cout<<elem<<" "; } cout<<"}"; return 0; }
输出
如果我们运行以上代码,它将生成以下输出 -
After erasing all odd numbers: { 18 12 10 }
示例 3
在下面的例子中,我们将使用 erase() 函数删除指定的元素。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<int> uSet = {10, 20, 30, 40, 50}; uSet.erase ( 30 ); uSet.erase ( 40 ); cout << "uSet contains:"; for ( auto it: uSet ) cout << " " << it; cout << endl; return 0; }
输出
以下是以上代码的输出 -
uSet contains: 50 20 10
示例 4
以下是示例,我们将删除范围内的元素。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<char> uSet = {'a', 'b', 'c', 'd', 'e'}; cout <<"uSet contains:"; for ( auto it: uSet ) cout <<" " <<it; cout <<endl; uSet.erase ( uSet.begin(), uSet.find('c') ); cout <<"after erased uSet contains:"; for ( auto it: uSet ) cout <<" " <<it; cout <<endl; return 0; }
输出
以上代码的输出如下 -
uSet contains: e d c b a after erased uSet contains: c b a
广告