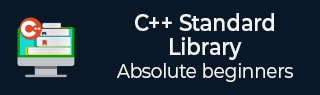
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_set::hash_function() 函数
C++ 的std::unordered_set::hash_function()用于获取已分配元素的哈希函数对象,该对象由 unordered_set 容器使用的哈希函数对象计算得出。
哈希函数对象是类的实例,具有为给定元素生成唯一哈希值的功能。它是一个一元函数,接受 key_type 对象作为参数,并根据它返回类型为 size_t 的唯一值。
语法
以下是 std::unordered_set::hash_function() 的语法。
hasher hash_function() const;
参数
此函数不接受任何参数。
返回值
此函数返回哈希函数。
示例 1
考虑以下示例,我们将演示 std::unordered_set::hash_function() 函数的使用。
#include <iostream> #include <string> #include <unordered_set> typedef std::unordered_set<std::string> stringset; int main () { stringset uSet; stringset::hasher fn = uSet.hash_function(); std::cout << "This contains: " << fn ("This") << std::endl; std::cout << "That contains: " << fn ("That") << std::endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
This contains: 16508604031426688195 That contains: 12586652871572997328
示例 2
让我们看看以下示例,我们将计算 unordered_set 中每个元素的哈希值。
#include <iostream> #include <unordered_set> using namespace std; int main(void) { unordered_set <string> uSet = {"Aman", "Vivek", "Rahul"}; auto fun = uSet.hash_function(); for(auto it = uSet.begin(); it!=uSet.end(); ++it){ cout << "Hash value of "<<*it<<" "<<fun(*it) << endl; } return 0; }
输出
以下是以上代码的输出:
Hash value of Rahul 3776999528719996023 Hash value of Vivek 13786444838311805924 Hash value of Aman 17071648282880668303
示例 3
在以下示例中,我们将计算类型为 char 的 unordered_set 中每个元素的哈希值。
#include <iostream> #include <unordered_set> using namespace std; int main(void) { unordered_set <char> uSet = {'a', 'b', 'c'}; auto fun = uSet.hash_function(); for(auto it = uSet.begin(); it!=uSet.end(); ++it){ cout << "Hash value of "<<*it<<" "<<fun(*it) << endl; } return 0; }
输出
以上代码的输出如下:
Hash value of c 99 Hash value of b 98 Hash value of a 97
广告