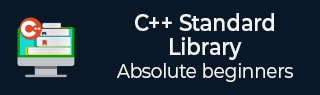
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_set::max_bucket_count() 函数
C++ 的unordered_set::max_bucket_count()函数用于返回unordered_set容器由于系统和库实现限制而持有的最大桶数。桶是容器内部哈希表中的一个槽,元素根据其键的哈希值分配到该槽中。它的编号范围从0到bucket_count - 1。
语法
以下是std::unordered_set::max_bucket_count()函数的语法。
size_type bucket_count() const noexcept;
参数
此函数不接受任何参数。
返回值
此函数返回unordered_set中最大桶数。
示例 1
让我们来看下面的例子,我们将演示unordered_set::max_bucket_count()函数的使用。
#include <iostream> #include <unordered_set> using namespace std; int main(void){ unordered_set<char> uSet = {'a', 'b', 'c', 'd'}; cout << " Maximum Number of buckets = " << uSet.max_bucket_count() << endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Maximum Number of buckets = 576460752303423487
示例 2
考虑下面的例子,我们将获取最大桶数并显示总桶数。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<string> uSet = {"Aman","Garav", "Sunil", "Roja", "Revathi"}; unsigned max = uSet.max_bucket_count(); cout << "uSet has maximum: " << max << " buckets. \n"; unsigned n = uSet.bucket_count(); cout << "uSet has total number of bucket: "<< n <<" buckets. \n"; return 0; }
输出
以下是上述代码的输出:
uSet has maximum:2863311530 buckets. uSet has total number of bucket: 13 buckets.
示例 3
在下面的例子中,我们将考虑两个unordered_set,一个是空的,另一个包含元素,并应用unordered_set::max_bucket_count()和bucket_count()函数。
#include <iostream> #include <unordered_set> using namespace std; int main() { unordered_set<char> uSet; uSet.insert({'a', 'b', 'c', 'd'}); unordered_set<char> myUSet; unsigned max1 = uSet.max_bucket_count(); int n1 = uSet.bucket_count(); cout << "uSet has maximum: " << max1 << " buckets.\n"; cout << "uSet has: " << n1 << " buckets. \n"; unsigned max2 = myUSet.max_bucket_count(); int n2 = myUSet.bucket_count(); cout << "myUSet has maximum: " << max2 << " buckets.\n"; cout << "myUSet has: " << n2 << " buckets. \n"; return 0; }
输出
上述代码的输出如下:
uSet has maximum: 4294967295 buckets. uSet has: 13 buckets. myUSet has maximum: 4294967295 buckets. myUSet has: 1 buckets.
广告