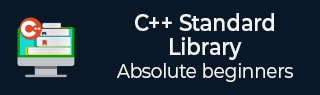
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_set::rehash() 函数
C++ 的std::unordered_set::rehash() 函数用于将 unordered_set 容器中的桶数设置为 n 或更多。重新哈希是哈希表的重建;所有元素都根据其新的哈希值重新排列到新的桶集中。
- 如果 n 大于容器中当前的桶数,则会发生重新哈希。
- 如果 n 小于容器中当前的桶数,则该函数可能对桶计数没有影响,并且可能不会强制重新哈希。
语法
以下是 std::unordered_set::rehash() 函数的语法。
void rehash ( size_type n );
参数
- n - 它表示桶的最小数量。
返回值
此函数不返回任何内容。
示例 1
让我们看一下下面的示例,我们将演示 unordered_set::rehash() 函数的使用。
#include <iostream> #include <string> #include <unordered_set> int main () { std::unordered_set<std::string> myset; myset.rehash(12); myset.insert("android"); myset.insert("java"); myset.insert("html"); myset.insert("css"); myset.insert("javascript"); std::cout << "current bucket_count: " << myset.bucket_count() << std::endl; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
current bucket_count: 13
示例 2
考虑以下示例,我们将考虑为空的 unordered_set,该集合为空,并在使用 unordered_set::rehash() 函数后显示其初始桶计数和当前桶计数。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<string> uSet; cout<<"Initial bucket count: "<<uSet.bucket_count()<<endl; uSet.rehash(10); cout << "current bucket_count: " << uSet.bucket_count() << endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Initial bucket count: 1 current bucket_count: 11
示例 3
让我们看一下 unordered_set::rehash() 函数用法的另一个示例,并显示使用函数前后计数。
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<int> uSet = {1, 2, 3, 4, 5}; cout<<"Initial bucket count: "<<uSet.bucket_count()<<endl; uSet.rehash(15); cout << "current bucket_count: " << uSet.bucket_count() << endl; return 0; }
输出
以下是上述代码的输出:
Initial bucket count: 13 current bucket_count: 17
示例 4
以下是一个示例,我们将使用 unordered_set::rehash() 函数设置桶数,并显示桶数。
#include <iostream> #include <unordered_set> using namespace std; int main() { unordered_set<int> uSet; uSet = { 2, 4, 6, 8 }; cout << "Size of unorder_set container : " << uSet.size() << endl; cout << "Initial bucket count : " << uSet.bucket_count() << endl; uSet.rehash(12); cout << "Size of unorder_set container : " << uSet.size() << endl; cout << "current bucket count is : " << uSet.bucket_count() << endl; for(unsigned int i = 0; i < uSet.bucket_count(); i++){ cout<<"The bucket #"<<i <<endl; } return 0; }
输出
上述代码的输出如下:
Size of unorder_set container : 4 Initial bucket count : 5 Size of unorder_set container : 4 current bucket count is : 13 The bucket #0 The bucket #1 The bucket #2 The bucket #3 The bucket #4 The bucket #5 The bucket #6 The bucket #7 The bucket #8 The bucket #9 The bucket #10 The bucket #11 The bucket #12
广告