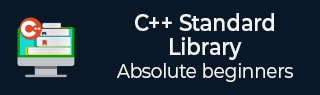
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_set::swap() 函数
C++ 的std::unordered_set::swap()函数用于交换unordered_set的内容与其他unordered_set的内容。如果两个unordered_set的内容相同,则什么也不做;否则,将一个unordered_set的内容与另一个unordered_set的内容交换。此函数的返回类型为void,这意味着它不返回值。
语法
以下是std::unordered_set::swap()函数的语法:
void swap ( unordered_set& other);
参数
- other − 另一个容器,用于交换内容。
返回值
此函数不返回值。
示例1
让我们来看下面的例子,我们将交换num_set1的内容与num_set2的内容。
#include<iostream> #include<string> #include<unordered_set> using namespace std; int main () { //create unordered_sets unordered_set<int> num_set1 = {1, 2, 3, 4, 5}; unordered_set<int> num_set2 = {6, 7, 8, 9, 10}; cout<<"Contents of the num_set1 before swap operation: "<<endl; for(int n1 : num_set1){ cout<<n1<<endl; } cout<<"Contents of the num_set2 before swap operation: "<<endl; for(int n2 : num_set2){ cout<<n2<<endl; } //using the swap() function num_set1.swap(num_set2); cout<<"Contents of the num_set1 after swap operation: "<<endl; for(int n1 : num_set1){ cout<<n1<<endl; } cout<<"Contents of the num_set2 after swap operation: "<<endl; for(int n2 : num_set2){ cout<<n2<<endl; } }
输出
如果我们运行上面的代码,它将生成以下输出:
Contents of the num_set1 before swap operation: 5 4 3 2 1 Contents of the num_set2 before swap operation: 10 9 8 7 6 Contents of the num_set1 after swap operation: 10 9 8 7 6 Contents of the num_set2 after swap operation: 5 4 3 2 1
示例2
考虑另一种情况,我们将考虑字符类型的unordered_set并应用swap()函数。
#include<iostream> #include<string> #include<unordered_set> using namespace std; int main () { //create unordered_sets unordered_set<char> char_set1 = {}; unordered_set<char> char_set2 = {'a', 'b', 'c', 'd'}; cout<<"Size of the char_set1 before swap operation: "<<char_set1.size()<<endl; cout<<"Contents of the char_set2 before swap operation: "<<endl; for(char c2 : char_set2){ cout<<c2<<endl; } //using the swap() function char_set1.swap(char_set2); cout<<"Contents of the char_set1 after swap operation: "<<endl; for(char c1 : char_set1){ cout<<c1<<endl; } cout<<"Size of the char_set2 after the swap operation: "<<char_set2.size(); }
输出
以下是上述代码的输出:
Size of the char_set1 before swap operation: 0 Contents of the char_set2 before swap operation: d c b a Contents of the char_set1 after swap operation: d c b a Size of the char_set2 after the swap operation: 0
示例3
在下面的例子中,我们将考虑字符串类型的unordered_set,并应用swap()函数来交换unordered_set的内容。
#include<iostream> #include<string> #include<unordered_set> using namespace std; int main () { //create unordered_sets unordered_set<string> str_set1 = {"Red", "Blue", "Yellow", "White"}; unordered_set<string> str_set2 = {"Apple", "Banana", "Mango", "Orange"}; cout<<"Contents of the str_set1 before swap operation: "<<endl; for(string s1 : str_set1){ cout<<s1<<endl; } cout<<"Content of the str_set2 before swap operation: "<<endl; for(string s2 : str_set2){ cout<<s2<<endl; } //using swap() operation str_set1.swap(str_set2); cout<<"Contents of the str_set1 after swap operation: "<<endl; for(string s1 : str_set1){ cout<<s1<<endl; } cout<<"Content of the str_set2 after swap operation: "<<endl; for(string s2 : str_set2){ cout<<s2<<endl; } }
输出
上述代码的输出如下:
Contents of the str_set1 before swap operation: White Yellow Blue Red Content of the str_set2 before swap operation: Mango Banana Orange Apple Contents of the str_set1 after swap operation: Mango Banana Orange Apple Content of the str_set2 after swap operation: White Yellow Blue Red
广告