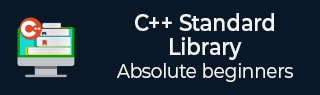
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ vector::crend() 函数
C++ vector::crend() 用于获取从反向结尾开始的向量的第一个元素。它返回一个指向向量第一个元素之前元素(即反向结尾)的常量反向迭代器。crend() 函数的时间复杂度为常数。
返回的 const_reverse_iterator 是一个指向常量内容(向量)的迭代器,它可以像迭代器一样增加或减少。但是,它不能用于更新或修改它指向的向量内容。
语法
以下是 C++ vector::crend() 函数的语法:
const_reverse_iterator crend() const noexcept;
参数
它不包含任何参数。
示例 1
让我们考虑以下示例,我们将使用 crend() 函数。
#include <iostream> #include <vector> using namespace std; int main(void){ vector<int> v = {1, 2, 3, 4, 5}; for (auto it = v.crend() - 1; it >= v.crbegin(); --it) cout << *it << endl; return 0; }
输出
编译并运行上述程序时,将产生以下结果:
1 2 3 4 5
示例 2
在下面的示例中,我们将使用 crend() 函数并访问向量中的第一个元素。
#include <iostream> #include<vector> using namespace std; int main(){ vector<string>car{"RX100","BMW","AUDI","YAMAHA"}; vector<string>::const_reverse_iterator x=car.crend()-1; std::cout<< *x; return 0; }
输出
运行上述程序后,将产生以下结果:
RX100
示例 3
考虑以下示例,我们将使用 push_back() 插入元素并使用 crend() 函数获取第一个元素。
#include <iostream> #include <vector> using namespace std; int main(){ vector<int> num; num.push_back(2); num.push_back(4); num.push_back(6); vector<int>::const_reverse_iterator x; x = num.crend()-1; cout << "The first element is: " << *x << endl; return 0; }
输出
运行上述程序后,将产生以下结果:
The first element is: 2
示例 4
查看以下示例,当我们尝试使用 crend() 函数修改值时,它会抛出错误。
#include <iostream> #include<vector> using namespace std; int main(){ vector<int> myvector{111,222,333,444}; vector<int>::const_reverse_iterator x=myvector.crend()-1; *x=8; cout<<*x; return 0; }
输出
运行上述程序后,将产生以下结果:
Main.cpp:8:5: error
广告