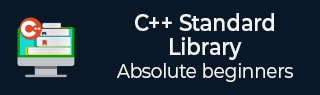
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ vector::end() 函数
C++ vector::end() 函数用于获取指向向量最后一个元素之后的迭代器。<vector> 头文件包含 end() 函数。end() 函数的时间复杂度为常数。
如果尝试解除对返回的向量的引用,则它将抛出一个垃圾值。要获取最后一个元素,我们必须从返回的迭代器中减去 1,即向后移动一个位置。如果向量为空,则无法解除对迭代器的引用。
语法
以下是 C++ vector::end() 函数的语法:
iterator end() noexcept; const_iterator end() const noexcept;
参数
它不接受任何类型的参数。
示例 1
让我们考虑以下示例,我们将使用 end() 函数。
#include <iostream> #include <vector> using namespace std; int main() { vector<int> myvector = {11,22,33,44}; vector<int> empty_vec = {}; auto x = myvector.end(); cout << *(x - 1); return 0; }
输出
当我们编译并运行上述程序时,将产生以下结果:
44
示例 2
考虑另一种情况,我们将使用 push_back() 函数插入元素并应用 end() 函数。
#include <iostream> #include <vector> using namespace std; int main(){ vector<int> myvector; myvector.push_back(12); myvector.push_back(23); vector<int>::iterator x; x = myvector.end()-1; cout << "Result : " << *x << endl; return 0; }
输出
运行上述程序后,将产生以下结果:
Result : 23
示例 3
在以下示例中,我们将使用字符串值并应用 end() 函数。
#include <iostream> #include <vector> using namespace std; int main (){ vector<string> tutorial{"JIM","JAM","JUM"}; vector<string>::iterator x; x = tutorial.end(); x--; cout<<*x<<" "; x--; cout<<*x<<" "; x--; cout<<*x<<" "; return 0; }
输出
当我们执行上述程序时,将产生以下结果:
JUM JAM JIM
广告