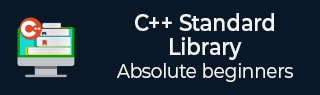
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ vector::operator<=() 函数
C++ vector::operator<=() 函数用于测试第一个向量是否小于或等于另一个向量,如果运算符左侧小于或等于向量右侧的向量,则返回 true,否则返回 false。运算符 <= 按顺序比较元素,并在第一次不匹配时停止比较。此成员函数永远不会抛出异常,并且 operator<=() 函数的时间复杂度为线性。
语法
以下是 C++ vector::operator<=() 函数的语法:
bool operator<=(const vector<Type, Allocator>& left, const vector<Type, Allocator>& right);
参数
- left - 表示运算符左侧的向量对象类型。
- right - 表示运算符右侧的向量对象类型。
示例 1
让我们考虑以下示例,我们将使用 opertor<=() 函数。
#include <iostream> #include <vector> using namespace std; int main (){ vector<int> myvector1 {123,234,345}; vector<int> myvector2 {123,234,345}; if (myvector1 <= myvector2) cout<<"myvector1 is less than or equal to myvector2.\n"; else cout<<"myvector1 is not less than or equal to myvector2.\n"; return 0; }
输出
当我们编译并运行上述程序时,将产生以下结果:
myvector1 is less than or equal to myvector2.
示例 2
考虑另一种情况,我们将获取字符串值并进行比较。
#include <iostream> #include <vector> using namespace std; int main (){ vector<string> myvector1 {"abc","bcd","cde"}; vector<string> myvector2 {"abc","bcd"}; if (myvector1 <= myvector2) cout<<"myvector1 is less than or equal to myvector2.\n"; else cout<<"myvector1 is not less than or equal to myvector2.\n"; return 0; }
输出
运行上述程序后,将产生以下结果:
myvector1 is not less than or equal to myvector2.
示例 3
在以下示例中,我们将使用 push_back() 函数插入值并应用 operator<=() 函数。
#include <vector> #include <iostream> int main( ){ using namespace std; vector <int> myvector1, myvector2; myvector1.push_back( 1 ); myvector1.push_back( 2 ); myvector1.push_back( 4 ); myvector2.push_back( 1 ); myvector2.push_back( 23 ); if ( myvector1 <= myvector2 ) cout << "myvector1 is less than or equal to vector myvector2." << endl; else cout << "myvector1 is greater than vector myvector2." << endl; }
输出
执行上述程序后,将产生以下结果:
myvector1 is less than or equal to vector myvector2.
广告