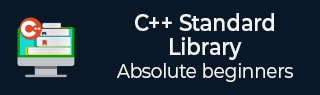
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ vector::operator!=() 函数
C++ vector::operator!=() 函数用于测试两个向量是否不相等,如果给定的两个向量不相等,则返回 true,否则返回 false。Operator !=() 函数首先检查两个容器的大小,如果大小相同,则按顺序比较元素,并在第一次不匹配时停止比较。此成员函数永远不会抛出异常,并且 operator!=() 函数的时间复杂度为线性。
语法
以下是 C++ vector::operator!=() 函数的语法:
template <class T, class Alloc> bool operator!= (const vector<T,Alloc>& lhs, const vector<T,Alloc>& rhs);
参数
- lhs - 表示第一个向量
- rhs - 表示第二个向量
示例 1
让我们考虑以下示例,我们将使用 opertor!=() 函数。
#include <iostream> #include <vector> using namespace std; int main(void) { vector<int> v1; vector<int> v2; v1.resize(10, 100); if (v1 != v2) cout << "v1 and v2 are not equal" << endl; v1 = v2; if (!(v1 != v2)) cout << "v1 and v2 are equal" << endl; return 0; }
输出
当我们编译并运行以上程序时,将产生以下结果:
v1 and v2 are not equal v1 and v2 are equal
示例 2
考虑另一种情况,我们将采用字符串值并应用 operator!=() 函数。
#include <iostream> #include <vector> using namespace std; int main (){ vector<string> myvector1 {"RS7","AUDI","MAYBACH GLS"}; vector<string> myvector2 {"RS7","AUDI","MAYBACH GLS"}; if (myvector1 != myvector2) cout<<"The Two Vectors are not equal.\n"; else cout<<"The Two Vectors are equal.\n"; return 0; }
输出
运行以上程序后,将产生以下结果:
The Two Vectors are equal.
示例 3
在以下示例中,我们将使用 push_back() 函数插入值,并检查向量是否相等。
#include <vector> #include <iostream> int main( ){ using namespace std; vector <int> myvector1, myvector2; myvector1.push_back( 11 ); myvector1.push_back( 113 ); myvector2.push_back( 222 ); myvector2.push_back( 113 ); if ( myvector1 != myvector2 ) cout << "Vectors are not equal." << endl; else cout << "Vectors are equal." << endl; }
输出
当我们执行以上程序时,将产生以下结果:
Vectors are not equal.
广告