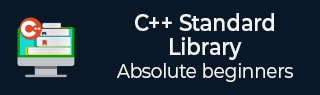
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ vector::reserve() 函数
C++ `vector::reserve()` 函数用于预留向量的容量。容量必须足够大,以便能够容纳 n 个元素。任何给定向量,只要其容量大于或等于我们在 `reserve()` 函数中指定的新的容量,其容量就可以通过 `reserve()` 函数来增加。
`reserve()` 函数只会预留向量的空间;它不会增加向量的大小。如果预留的向量大小大于当前大小,所有这些调整都将失效。`reserve()` 函数的时间复杂度最多为线性。
语法
以下是 C++ `vector::reserve()` 函数的语法:
void reserve (size_type n);
参数
n − 表示将存储在向量中的元素数量。
示例 1
让我们考虑以下示例,我们将使用 `reserve()` 函数。
#include <iostream> #include <vector> using namespace std; int main(void) { vector<int> v1; vector<int> v2; ssize_t size; size = v1.capacity(); for (int i = 0; i < 25; ++i) { v1.push_back(i); if (size != v1.capacity()) { size = v1.capacity(); cout << "Expanding vector v1 to hold " << size<< " elements" << endl; } } cout << endl << endl; v2.reserve(25); for (int i = 0; i < 25; ++i) { v2.push_back(i); if (size != v2.capacity()) { size = v2.capacity(); cout << "Expanding vector v2 to hold " << size<< " elements" << endl; } } return 0; }
输出
编译并运行上述程序后,将产生以下结果:
Expanding vector v1 to hold 1 elements Expanding vector v1 to hold 2 elements Expanding vector v1 to hold 4 elements Expanding vector v1 to hold 8 elements Expanding vector v1 to hold 16 elements Expanding vector v1 to hold 32 elements Expanding vector v2 to hold 25 elements
示例 2
考虑另一种情况,我们将分配和释放空间。
#include <cstddef> #include <iostream> #include <new> #include <vector> template<class Tp> struct tutorial{ typedef Tp value_type; tutorial() = default; template<class x> tutorial(const tutorial<x>&) {} Tp* allocate(std::size_t n){ n *= sizeof(Tp); Tp* p = static_cast<Tp*>(::operator new(n)); std::cout << "allocating " << n << " bytes @ " << p << '\n'; return p; } void deallocate(Tp* p, std::size_t n){ std::cout << "deallocating " << n * sizeof *p << " bytes @ " << p << "\n\n"; ::operator delete(p); } }; template<class x, class y> bool operator==(const tutorial<x>&, const tutorial<y>&) { return true; } template<class x, class y> bool operator!=(const tutorial<x>&, const tutorial<y>&) { return false; } int main(){ constexpr int max_elements = 10; std::cout << "using reserve() function : \n"; { std::vector<int, tutorial<int>> v1; v1.reserve(max_elements); for (int n = 0; n < max_elements; ++n) v1.push_back(n); } }
输出
运行上述程序后,将产生以下结果:
using reserve() function : allocating 40 bytes @ 0x55981828e2c0 deallocating 40 bytes @ 0x55981828e2c0
示例 3
在下面的示例中,我们将检查使用 `reserve()` 函数和不使用 `reserve()` 函数的情况。
#include <cstddef> #include <iostream> #include <new> #include <vector> template<class a> struct tutorial{ typedef a value_type; tutorial() = default; template<class x> tutorial(const tutorial<x>&) {} a* allocate(std::size_t n){ n *= sizeof(a); a* p = static_cast<a*>(::operator new(n)); std::cout << "allocating " << n << " bytes @ " << p << '\n'; return p; } void deallocate(a* p, std::size_t n){ std::cout << "deallocating " << n * sizeof *p << " bytes @ " << p << "\n\n"; ::operator delete(p); } }; template<class x, class y> bool operator==(const tutorial<x>&, const tutorial<y>&) { return true; } template<class x, class y> bool operator!=(const tutorial<x>&, const tutorial<y>&) { return false;} int main(){ constexpr int max_elements = 8; std::cout << "using reserve() function: \n"; { std::vector<int, tutorial<int>> v1; v1.reserve(max_elements); for (int n = 0; n < max_elements; ++n) v1.push_back(n); } std::cout << "without reserve() function : \n"; { std::vector<int, tutorial<int>> v1; for (int n = 0; n < max_elements; ++n){ if (v1.size() == v1.capacity())std::cout << "size() == capacity() == " << v1.size() << '\n'; v1.push_back(n); } } }
输出
执行上述程序后,将产生以下结果:
using reserve() function: allocating 32 bytes @ 0x55d7dd6ca2c0 deallocating 32 bytes @ 0x55d7dd6ca2c0 without reserve() function : size() == capacity() == 0 allocating 4 bytes @ 0x55d7dd6ca2f0 size() == capacity() == 1 allocating 8 bytes @ 0x55d7dd6ca310 deallocating 4 bytes @ 0x55d7dd6ca2f0 size() == capacity() == 2 allocating 16 bytes @ 0x55d7dd6ca2f0 deallocating 8 bytes @ 0x55d7dd6ca310 size() == capacity() == 4 allocating 32 bytes @ 0x55d7dd6ca2c0 deallocating 16 bytes @ 0x55d7dd6ca2f0 deallocating 32 bytes @ 0x55d7dd6ca2c0
广告