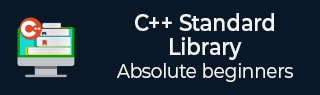
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ vector::swap() 函数
C++ vector::swap() 函数用于交换两个向量的元素。向量长度不同并不构成问题。两个向量只有在类型相同的情况下才能交换。swap() 函数的时间复杂度为常数。
vector 类的成员函数可以交换自身和另一个向量。算法库还包含各种名称不同的 swap 函数,用于其他目的。算法库 swap 函数与 vector swap() 函数之间的主要区别在于,vector 函数将自身向量与另一个向量交换,而算法库 swap 函数则分别交换两个独立的向量。
语法
以下是 C++ vector::swap() 函数的语法:
void swap (vector& x);
参数
x - 表示另一个相同类型的向量,其内容将被交换。
示例 1
让我们考虑以下示例,我们将使用 swap() 函数。
#include <iostream> #include <vector> using namespace std; int main(){ vector<int> tutorial1{11,22,33,44}; vector<int> tutorial2{111,222,333,444}; tutorial1.swap(tutorial2); cout << "vector1 Elements are : "; for (auto x = tutorial1.begin(); x < tutorial1.end(); ++x) cout << *x << " "; cout << endl<< "vector2 Elements are : "; for (auto x = tutorial2.begin(); x < tutorial2.end(); ++x) cout << *x << " "; return 0; }
输出
当我们编译并运行上述程序时,将产生以下结果:
vector1 Elements are : 111 222 333 444 vector2 Elements are : 11 22 33 44
示例 2
考虑另一种情况,我们将使用两个不同大小的向量并应用 swap() 函数。
#include <iostream> #include <vector> using namespace std; int main(){ vector<int> myvector1{12,23}; vector<int> myvector2{34,45,56,67}; myvector1.swap(myvector2); cout << "The vec1 elemets are:"; for (int i = 0; i < myvector1.size(); i++) cout << ' ' << myvector1[i]; cout << '\n'; cout << "The vec2 elemets are:"; for (int i = 0; i < myvector2.size(); i++) cout << ' ' << myvector2[i]; cout << '\n'; return 0; }
输出
运行上述程序后,将产生以下结果:
The vec1 elemets are: 34 45 56 67 The vec2 elemets are: 12 23
示例 3
在以下示例中,我们将使用 char 类型并在迭代器之间应用 swap() 函数。
#include <iostream> #include <algorithm> #include <vector> using namespace std; int main(){ vector<char> myvector1 = {'T', 'P'}; vector<char> myvector2 = {'W', 'E', 'L', 'C', 'O', 'M', 'E'}; vector<char>::iterator x = myvector1.begin(); vector<char>::iterator y = myvector2.begin(); swap(x, y); for (x = x; x != myvector2.end(); x++) { cout << *x << ' '; } cout << endl; for (y = y; y != myvector1.end(); y++) { cout << *y << ' '; } cout << endl; return 0; }
输出
执行上述程序后,将产生以下结果:
W E L C O M E T P
广告