如何在 React 中使用 Material UI 添加样式组件?
Styled Components 是 React 生态系统中一个备受赞誉的库,它使开发者能够使用 CSS-in-JS,从而带来封装性、可重用性和简化样式维护等优势。另一方面,Material-UI 是一个广泛使用的 React UI 组件库,它严格遵循 Material Design 的原则。它提供了大量可自定义的预构建组件。虽然 Material-UI 自带了一个名为 makeStyles 的样式解决方案,但它可以与 Styled Components 无缝集成,使开发者能够利用这两个库的组合功能。
我们的探索围绕安装过程展开,重点介绍了 @mui/styled-engine 和 @mui/styled-engine-sc 包。这些包使开发者能够在为 Material UI 组件设置样式时选择 Emotion 或 Styled Components。本指南通过清晰的代码示例和全面的说明,使开发者能够掌握使用样式组件的知识,从而在 Material UI 框架内创建视觉效果出众且易于维护的用户界面。
在本篇综合指南中,我们将深入探讨如何利用 React 的强大功能,将样式组件无缝集成到 Material UI 中。
样式组件到底是什么?
Styled Components 是 React 生态系统中一个著名的库,它使开发者能够编写 CSS-in-JS,从而能够直接在 JavaScript 代码中定义和应用样式。这种创新方法提供了许多优势,包括封装性、可重用性和简化样式维护。
另一方面,Material-UI 是一个广泛使用的 React UI 组件库,它严格遵循 Material Design 的指南。它为开发者提供了一个广泛的预构建组件集合,所有这些组件都是可自定义的,从而有助于创建视觉效果出众的用户界面。尽管 Material-UI 有自己名为 makeStyles 的样式解决方案,但它与 Styled Components 完全兼容,允许开发者利用这两个库的组合潜力。
Emotion 库是用于为 Material UI 组件生成 CSS 样式的默认样式解决方案。每个 Material UI 组件都依赖于 styled() API 来将 CSS 无缝注入页面。此 API 受各种流行的样式库支持,从而提供了在 Material UI 框架内轻松切换它们的功能。
安装过程
要开始将 Styled Components 集成到 Material-UI 中,必须安装一系列必需的包。如果您的项目中已经存在 Styled Components,则可以只使用它们。Material-UI 提供了两个不同的包可供选择:@mui/styled-engine 和 @mui/styled-engine-sc。
前者是默认包,包含 Emotion 的 styled() API 和补充实用程序。后者则是一个类似的包装器,专门为 Styled Components 设计。根据您的喜好和需求,您可以轻松配置您的项目以使用任一包。
通过 npm 安装
$ npm install @mui/material @mui/styled-engine-sc styled-components
配置
配置在 React 项目的 webpack.config.js 文件中完成。
module.exports = { //... + resolve: { + alias: { + '@mui/styled-engine': '@mui/styled-engine-sc' + }, + }, };
通过 yarn 安装
$ yarn add @mui/material @mui/styled-engine-sc styled-components
配置
配置在 React 项目的 package.json 文件中完成。
{ "dependencies": { - "@mui/styled-engine": "latest" + "@mui/styled-engine": "npm:@mui/styled-engine-sc@latest" }, + "resolutions": { + "@mui/styled-engine": "npm:@mui/styled-engine-sc@latest" + }, }
在 Next.js 中安装
$ npx [email protected] $ cd my-next-app $ yarn add @mui/material @mui/styled-engine-sc styled-components
在 Material-UI 中实现 Styled Components
要将 Styled Components 集成到 Material UI 中,您可以轻松地为每个 Material UI 组件应用自定义样式。通过使用 Styled Components 提供的 styled 函数,您可以为组件定义样式。
您创建的 CSS 规则将仅限于其组件,这确保了样式不会相互冲突。此外,您可以选择通过扩展 Material UI 组件并向其应用样式来创建样式组件。这确保了整个应用程序的视觉效果。
示例 1
在给定示例中使用 styled engine sc 包时,开发者可以使用 @mui/system 包中的 styled 函数。此函数允许通过将 CSS-in-JS 对象作为输入来创建样式组件。
//import react and styled component from styled-engine-sc import * as React from "react"; import { styled } from "@mui/system"; //create a styled div const StyledDiv = styled("div")` display: flex; flex-direction: column; width: 40em; gap: 2em; `; //create a styled text area input const StyledTextArea = styled("textarea")` padding: 10px; border: 1px solid green; border-radius: 4px; height: 10em; `; //create a styled button const StyledButton = styled("button")` background-color: #189e02; color: #fff; box-shadow: 0 4px 6px green, 0 1px 3px black; padding: 7px 14px; border-radius: 5px; &:hover { background-color: #18ac00; } `; //main function App component function App() { return ( <StyledDiv> <h1>Styling Material UI using Styled Components</h1> <StyledTextArea /> <StyledButton>Add</StyledButton> </StyledDiv> ); } export default App;
输出
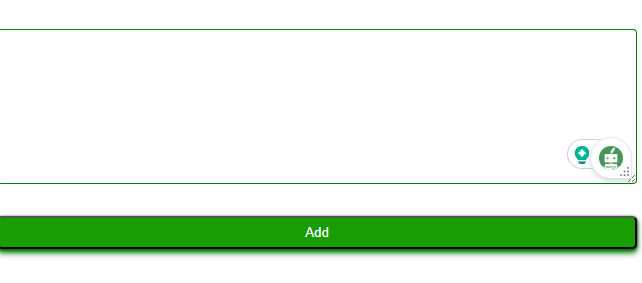
示例 2
当您在给定示例中使用 styled engine 包作为开发者时,您可以访问创建样式组件的深入 API。此包还使您能够使用 @mui/styled engine 中的 createStyled 函数创建自定义样式引擎。通过使用 styled engine 包,开发者可以灵活地控制样式引擎,从而能够根据自己的需求和偏好自定义它。
//import react and styled component from styled-engine import * as React from "react"; import { styled } from "@mui/material/styles"; //create a styled div const StyledDiv = styled("div")` display: flex; flex-direction: column; width: 40em; gap: 2em; `; //create a styled input for email const StyledInputEmail = styled("input")` padding: 10px; border: 1px solid #ccc; border-radius: 4px; outline: none; `; //create a styled input for password const StyledInputPass = styled("input")` padding: 10px; border: 1px solid #ccc; border-radius: 4px; outline: none; `; //create a styled button const StyledButton = styled("button")` background-color: #189e02; color: #fff; box-shadow: 0 4px 6px green, 0 1px 3px black; padding: 7px 14px; border-radius: 5px; &:hover { background-color: #18ac00; } `; // function App() { return ( <StyledDiv> <h1>Styling Material UI using Styled Components</h1> <StyledInputEmail type="email" placeholder="Enter your email" /> <StyledInputPass type="password" placeholder="Enter your password" /> <StyledButton>Submit</StyledButton> </StyledDiv> ); } export default App;
输出
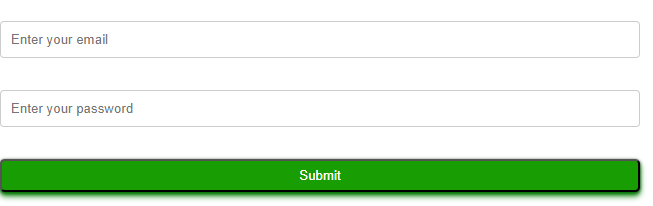
结论
本文提供了有关使用 React 将样式组件集成到 Material UI 中的见解。凭借其 CSS-in-JS 方法,样式组件为开发者提供了封装性、可重用性和简化样式维护等优势。Material UI 是一个遵循 Material Design 原则的 UI 组件库,它提供了一系列可轻松自定义的预构建组件。
为了利用这两个库的组合潜力,开发者可以。配置 @mui/styled engine sc 或 @mui/styled engine 包。这些包允许在 Material UI 框架内使用样式组件作为样式解决方案。通过提供代码示例和详细说明,本指南使开发者能够创建视觉效果吸引人且易于管理的用户界面。