如何使用 Material UI 在 ReactJS 中创建暗黑模式?
我们将学习如何使用 Material UI 库在 ReactJS 中创建暗黑模式。Material UI 是一个外部 React 库,它提供设计好的 React 组件,我们可以通过从库中导入直接在我们的 React 项目中使用。
在全世界范围内,大多数用户喜欢暗黑模式,只有一部分人喜欢亮色模式。暗黑模式有助于减少访客的眼睛疲劳,并显得更加高档。因此,我们应该允许用户根据自己的偏好选择暗黑模式或亮色模式。
在原生 JavaScript 或 JQuery 中,我们可以通过添加和删除暗黑主题的类来创建暗黑和亮色模式。在 React 中,我们可以使用 Material-Ui 等外部库来在应用程序中实现暗黑模式。
在开始之前,用户需要在 React 项目中安装 Material-Ui 库。在终端中执行以下命令。
npm install @mui/material @emotion/react @emotion/styled
语法
用户可以按照以下语法使用 ReactJS 的 Material-Ui 库创建暗黑模式。
const darkTheme = createTheme({ palette: { mode: "dark", }, }); <ThemeProvider theme={darkTheme}> <CssBaseline /> </ThemeProvider>
在上面的语法中,我们使用了来自 Material-Ui 的 ThemeProvider 组件,并将 darkTheme 对象作为 ThemeProvider 组件的一个属性传递。此外,我们需要使用 CssBaseline 组件来应用暗黑主题的 CSS;否则,它将无法正常工作。
示例 1
在下面的示例中,我们导入了来自 Material Ui 库的 ThemeProvider 组件和 createTheme 构造函数。之后,我们使用了 createTheme() 构造函数并创建了暗黑主题。
我们需要将组件的内容绑定到 ThemeProvider 组件内部,并将主题对象作为 ThemeProvider 组件的属性传递。在输出中,用户可以看到暗黑主题。
import { ThemeProvider, createTheme } from "@mui/mater/styles; import CssBaseline from "@mui/material/CssBaseline"; const theme = createTheme({ palette: { mode: "dark", }, }); function App() { const divStyle = { display: "flex", flexDireciton: "row", textAlign: "center", border: "5px dotted red", width: "600px", height: "300px", marginLeft: "1rem", padding: "1rem", }; const ChildDivStyle = { height: "80%", width: "45%", margin: "1.5rem", backgroundColor: "White", }; return ( <ThemeProvider theme={theme}> <CssBaseline /> <div> <h2> {" "} Using the <i> Material UI </i> library to create dark mode for the React web application. {" "} </h2> <div style = {divStyle}> <div style = {ChildDivStyle}> </div> <div style = {ChildDivStyle}> </div> </div> </div> </ThemeProvider> ); } export default App;
输出
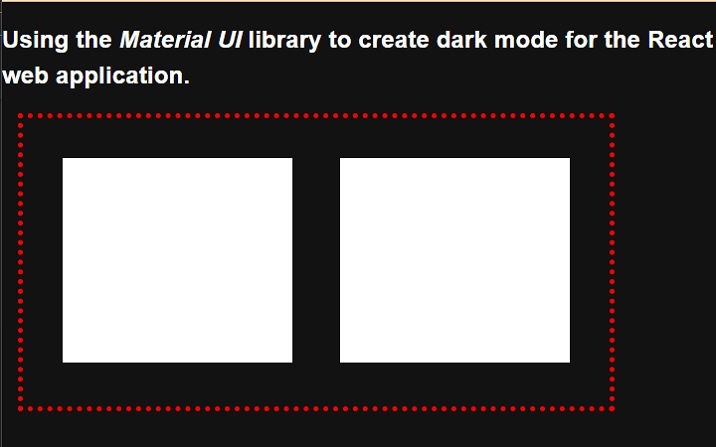
示例 2 的步骤
用户可以按照以下步骤创建示例 2。
步骤 1 − 从 Material-Ui 库导入 ThemeProvider、CreateTheme 和 CSSBaseline。
步骤 2 − 现在,使用 ThemeProvider 组件并将其他组件绑定以应用主题。
步骤 3 − 接下来,创建一个按钮来切换主题。当用户单击按钮时,它应该调用 handleThemeChange() 函数。
步骤 4 − 现在,设置主题的变量。我们将根据 ‘themeLight’ 变量的布尔值选择亮色或暗黑主题。
步骤 5 − 使用 createTheme() 构造函数根据 themeLight 变量的值创建主题。对于条件主题,我们可以使用三元运算符。
步骤 6 − 接下来,还要实现 handleThemeChange() 函数。在 handleThemeChange() 函数中,更改 themeLight 变量的值以在亮色和暗黑主题之间切换。
示例 2
在下面的示例中,我们使用了 Material-Ui 库将暗黑和亮色主题应用于 React 应用程序。当用户单击按钮时,它会从亮色主题更改为暗黑主题,或从暗黑主题更改为亮色主题。
import { ThemeProvider, createTheme } from "@mui/material/styles"; import CssBaseline from "@mui/material/CssBaseline"; import React from "react"; import { useState } from "react"; function App() { const [themeLight, setThemeType] = useState(true); const theme = createTheme({ palette: { mode: themeLight ? "light" : "dark", }, }); function handleThemeChange() { setThemeType(!themeLight); } const divStyle = { textAlign: "center", border: "5px solid green", width: "500px", height: "400px", marginLeft: "1rem", padding: "1rem", fontSize: "1.2rem", color: "blue,", }; const buttonStyle = { width: "15rem", height: "2rem", borderRadius: "10px", border: "1px solid green", backgroundColor: "darkGrey", fontSize: "1.5rem", padding: "2px", marginLeft: "1rem", }; return ( <ThemeProvider theme={theme}> <CssBaseline /> <div> <h2> {" "} Using the <i> Material UI </i> library to create dark mode for the React web application. {" "} </h2> <h3> Use the button to toggle the theme. </h3> <div style = {divStyle}> <p>This is the content of the Div! </p> <p>This is another content of the Div! </p> <p>This is the test text of the Div! </p> <p>This is the last line of the Div! </p> </div> </div> <br></br> <button style = {buttonStyle} onClick = {handleThemeChange}> Change Theme </button> </ThemeProvider> ); } export default App;
输出
用户学习了如何使用 Material Ui 库在暗黑和亮色模式之间切换。用户可以在示例输出中观察到,当我们从亮色模式切换到暗黑模式时,Material UI 会更改字体颜色和背景。这意味着它会更改我们没有定义样式的 HTML 元素的 CSS,如果我们已对任何 HTML 元素应用 CSS,则它保持不变。