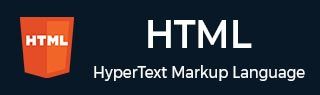
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与发展
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块级元素
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内嵌框架 (iframe)
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头与标题
- HTML - 表格样式
- HTML - 表格列组 (colgroup)
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮箱链接
- HTML 颜色名称与值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 多媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - head 元素
- HTML - 添加 Favicon
- HTML - JavaScript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML APIs
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - 网页消息传递
- HTML - Web CORS
- HTML - WebRTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizr
- HTML - 验证
- HTML - 颜色选择器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 childNodes 属性
在 HTML DOM 中,**childNodes** 属性检索元素的所有子节点,包括元素、文本节点和注释。这是一个只读属性,通常用于内容操作。
与 childElementCount 不同,它涵盖所有类型的子节点。
语法
element.childNodes;
返回值
此属性返回一个 NodeList,其中包含指定元素的所有子节点。
HTML DOM 元素 'childNodes' 属性示例
以下是一些显示 HTML DOM 中 childNodes 属性用法的示例。
计算并显示子节点数量
此示例显示了 childNodes 属性的基本用法,用于动态计算和显示子元素的数量。以下代码包含一个按钮,用于计算和显示'父' div 内的子元素('<p>' 和 '<div>')的数量。
<!DOCTYPE html> <html> <head> <title>Child Nodes Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>ChildNodes Property</h2> <div id="parent"> <p>This is a parent paragraph.</p> <div> <p>This is a nested paragraph.</p> <span>This is a nested span element.</span> </div> </div> <button onclick="countChildNodes()"> Count Child Nodes </button> <div id="output"></div> <script> function countChildNodes() { const count = document.getElementById ('parent').querySelectorAll('*').length; document.getElementById('output').textContent = `Number of child elements: ${count}`; } </script> </body> </html>
修改子节点
此示例显示如何使用 childNodes 属性来修改 HTML 元素内的子节点。以下代码包含一个按钮,用于移除最初的 <p> 段落,并动态地向 'parent' 添加一个新的 <h2> 标题。
<!DOCTYPE html> <html lang="en"> <head> <title>Modifying Child Nodes</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>childNodes property</h2> <p>Click the button to modify child nodes.</p> <div id="parent"> <p>This is a paragraph.</p> <ul> <li>Item 1</li> <li>Item 2</li> </ul> <span>Another child</span> </div> <button onclick="modifyChildNodes()"> Modify Child Nodes </button> <script> function modifyChildNodes() { const parentElement = document.getElementById('parent'); const firstChild = parentElement.childNodes[0]; parentElement.removeChild(firstChild); const newElement = document.createElement('h2'); newElement.textContent = 'New Heading'; parentElement.appendChild(newElement); } </script> </body> </html>
使用 'childNodes' 属性访问子节点
此示例显示如何使用 childNodes 属性访问和显示有关 id 为 "parent" 的 <div> 元素内子节点(<p> 元素和 <span> 元素)的信息。
<!DOCTYPE html> <html> <head> <title>ChildNodes Property Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>ChildNodes Property </h2> <p>It displays the parent node's child nodes</p> <p>Whitespace between elements are text nodes.</p> <div id="parent"> <p>This is the first paragraph.</p> <p>This is the second paragraph.</p> <span>This is a span element.</span> </div> <button onclick="displayChildNodes()"> Display Child Nodes </button> <div id="output"></div> <script> function displayChildNodes() { const parent = document.getElementById('parent'); const childNodes = parent.childNodes; let res = "<p><strong>Child Nodes:</strong></p>"; childNodes.forEach(node => { res+=`<p>${node.nodeName}-${node.nodeType}</p>`; }); document.getElementById('output').innerHTML =res; } </script> </body> </html>
动态添加和移除子节点
此示例帮助我们理解如何动态地使用 childNodes 属性来添加和移除子节点。以下代码通过点击“添加”按钮添加一个包含新内容的新 <p> 节点,并通过点击“移除”按钮移除最后一个子节点。
<!DOCTYPE html> <html> <head> <title>Manipulating Child Nodes</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>childNodes Property</h2> <h2>Manipulating Child Nodes</h2> <div id="container"> <p>This is the first paragraph.</p> <span>This is a span element.</span> </div> <button onclick="addNewNode()">Add New Node</button> <button onclick="removeLastNode()"> Remove Last Node </button> <div id="output"></div> <script> const container=document.getElementById('container'); function addNewNode() { container.innerHTML+= '<p>This is a new paragraph added dynamically.</p>'; updateOutput(); } function removeLastNode() { const childNodes = container.children; if (childNodes.length > 0) { container.removeChild(container.lastElementChild); updateOutput(); } } function updateOutput() { const childNodes = container.children; let res = "<p><strong>Current Child Nodes:</strong></p>"; Array.from(childNodes).forEach(node => { res+=`<p>${node.nodeName}:${node.textContent}</p>`; }); document.getElementById('output').innerHTML = res; } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
childNodes | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告