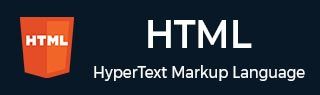
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引号
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内嵌框架
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - 头元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 insertAdjacentElement() 方法
**insertAdjacentElement()** 方法允许您在网页上将新的 HTML 元素精确地插入到另一个元素的相对位置。
语法
element.insertAdjacentElement(position, elementToInsert);
参数
参数 | 描述 |
---|---|
position | 指定相对于元素插入 HTML 内容的位置 beforebegin afterbegin beforeend afterend |
elementToInsert | 要插入结构中的新元素。 |
位置选项
- **beforebegin:** 放置在指定元素之前。
- **afterbegin:** 插入到指定元素内容的开头之后。
- **beforeend:** 插入到指定元素内容的结尾之前。
- **afterend:** 添加到指定元素之后。
返回值
此方法直接在指定位置插入,不返回值。
HTML DOM 元素“insertAdjacentElement()”方法示例
以下是一些关于 insertAdjacentElement() 方法如何指定不同元素插入位置的示例。
在 beforebegin 处插入新内容
此示例演示了在 HTML DOM 中使用 insertAdjacentElement 方法。它在现有的具有 id 为 container 的 **<div>** 元素之前插入一个新的 **<p>** 元素,其中包含文本“New content-beforebegin”。
<!DOCTYPE html> <html lang="en"> <head> <style> .container { border: 1px solid #ccc; padding: 10px; margin: 10px; } </style> </head> <body> <h1>HTML DOM Element</h1> <h2>insertAdjacentElement() Method</h2> <p>The container starts the existing content.</p> <p>Adds content before the existing element....</p> <div id="container" class="container"> <h2 id="sectionLabel">Before:</h2> <p>This is the existing content.</p> </div> <button onclick="addNewElement()"> Add New Element Before Beginning </button> <script> function addNewElement() { let newElement = document.createElement('p'); newElement.textContent ='New content - beforebegin'; newElement.style.backgroundColor = 'lightgreen'; document.getElementById ('container').insertAdjacentElement ('beforebegin', newElement); document.getElementById('sectionLabel').textContent= 'After:'; } </script> </body> </html>
在 afterbegin 处插入新内容
此示例演示了在 HTML DOM 中使用 insertAdjacentElement 方法。它在现有的具有 id 为 container 的 <div> 元素的开头之后插入一个新的 <p> 元素,其中包含文本“New content-afterbegin”。
<!DOCTYPE html> <html lang="en"> <head> <style> .container { border: 1px solid #ccc; padding: 10px; margin: 10px; } </style> </head> <body> <h1>HTML DOM Element</h1> <h2>insertAdjacentElement() Method</h2> <p>The container(box) starts the existing content.</p> <p>Adds content after the existing element....</p> <div id="container" class="container"> <h3 id="sectionLabel">Before:</h3> <p>This is the existing content.</p> </div> <button onclick="addNewElement()"> Add New Element After Beginning </button> <script> function addNewElement() { let newElement = document.createElement('p'); newElement.textContent = 'New content - afterbegin'; newElement.style.backgroundColor = 'lightblue'; document.getElementById('container').insertBefore (newElement,document.querySelector ('#container > h3')); document.getElementById('sectionLabel').textContent= 'After:'; } </script> </body> </html>
在 beforeend 处插入新内容
此示例演示了在 HTML DOM 中使用 insertAdjacentElement 方法。它在现有的具有 id 为 myContainer 的 <div> 元素的结尾之前插入一个新的 <p> 元素,其中包含文本“New content-beforeend”。
<!DOCTYPE html> <html lang="en"> <head> <style> .container { border: 1px solid #ccc; padding: 10px; margin: 10px; } </style> </head> <body> <h1>HTML DOM Element</h1> <h2>insertAdjacentElement() Method</h2> <p>Container marks the content's end..</p> <p>Adds content before the existing element....</p> <div class="container" id="myContainer"> <h3>Before:</h3> <p>This is the existing content.</p> </div> <button onclick="addNewElement()"> Add New Element Before End </button> <script> function addNewElement() { let newElement = document.createElement('p'); newElement.textContent = 'New content beforeend'; newElement.style.backgroundColor = 'lightblue'; // Insert the new element before the existing one let container = document.getElementById ('myContainer'); container.insertAdjacentElement ('beforeend', newElement); } </script> </body> </html>
在 afterend 处插入新内容
此示例演示了在 HTML DOM 中使用 insertAdjacentElement() 方法。它在现有的具有 id 为 container 的 <div> 元素的结尾之后插入一个新的 <p> 元素,其中包含文本“New content-afterend”。
<!DOCTYPE html> <html lang="en"> <head> <style> .container { border: 1px solid #ccc; padding: 10px; margin: 10px; } </style> </head> <body> <h1>HTML DOM Element</h1> <h2>insertAdjacentElement() Method</h2> <p>Container marks the content's end..</p> <p>Adds new content after the existing one...</p> <div id="container" class="container"> <h3 id="sectionLabel">Before:</h3> <p>This is the existing content.</p> </div> <button onclick="addNewElement()"> Add New Element After End </button> <script> function addNewElement() { let newElement = document.createElement('p'); newElement.textContent = 'New content - afterend'; newElement.style.backgroundColor = 'lightcoral'; // Insert new element after the existing one let existingElement = document.getElementById('container'); existingElement.insertAdjacentElement ('afterend', newElement); let sectionLabel = document.getElementById('sectionLabel'); sectionLabel.textContent = 'After:'; } </script> </body> </html>
支持的浏览器
方法 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
insertAdjacentElement() | 是 5.0 | 是 12.0 | 是 8.0 | 是 5.0 | 是 11.50 |
html_dom_element_reference.htm
广告