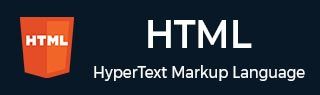
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内联框架 (iframe)
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 多媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity 绘图
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 nodeType 属性
nodeType 属性返回一个数字,告诉我们文档中节点的类型。元素节点返回 1,文本节点返回 3,注释节点返回 8,还有其他一些可能的值。
语法
node.nodeType
返回值
nodeType 属性返回一个整数,表示节点的类型。
节点类型及其属性。
整数值 | 节点类型 | 节点名称 | 节点值 |
---|---|---|---|
1 | 元素 | 元素名称(大写) | null |
2 | 属性 | 属性名称 | 属性值 |
3 | 文本 | #text | 文本节点的内容 |
4 | CDATA 节 | #cdata-section | CDATA 节的内容 |
5 | 实体引用 | 实体引用名称 | null |
6 | 实体 | 实体名称 | null |
7 | 处理指令 | 处理指令的目标 | 处理指令的内容 |
8 | 注释 | #comment | 注释文本 |
9 | 文档 | #document | null |
10 | 文档类型 | 文档类型名称 | null |
11 | 文档片段 | #document fragment | null |
12 | 符号 | 符号名称 | null |
HTML DOM 元素 'nodeType' 属性示例
以下是一些示例,用于更好地理解 'nodeType' 属性的用法。
获取 h1 元素的节点类型。
此示例演示如何使用 nodeType 属性来识别和处理 DOM 中不同类型的节点,尤其关注<h1> 元素。代码选择 <h1> 元素并返回此元素的节点类型。
<!DOCTYPE html> <html lang="en"> <head> <title>nodeType Property Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>nodeType Property</h2> <p>Displays the node type of a particular node:</p> <script> window.onload = function() { var header = document.querySelector('h1'); var nodeType = header.nodeType; // Display nodeType value in the HTML var resultDiv=document.getElementById('res'); resultDiv.textContent='Node Type:'+nodeType; // Additional check for demonstration if (nodeType === Node.ELEMENT_NODE) { resultDiv.textContent += ' (This is an element node)'; } else { resultDiv.textContent += ' (This is not an element node)'; } }; </script> <div id="res"></div> </body> </html>
获取段落的节点类型
此示例帮助我们使用 nodeType 属性识别和显示段落 (<p>) 元素中文本节点的信息。结果显示在 ID 为 "result" 的<div> 元素中。
<!DOCTYPE html> <html lang="en"> <head> <title>nodeType Property Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>nodeType Property</h2> <p>This paragraph contains <strong>some text</strong> and <em>emphasis</em>. </p> <script> window.onload = function() { var paragraph = document.querySelector('p'); var nodeType = paragraph.firstChild.nodeType; // Display nodeType value in the HTML var resultDiv=document.getElementById('res'); resultDiv.textContent = 'Node Type: ' + nodeType + (nodeType === Node.TEXT_NODE ?'(This is a Text node)':'(Not text node)'); }; </script> <div id="res"></div> </body> </html>
显示 ul 元素的节点类型
此示例演示如何使用 nodeType 属性迭代<ul> 元素中子节点的类型,包括<li> 元素、注释和文本节点。代码显示每个子节点的返回类型和整数值。
<!DOCTYPE html> <html lang="en"> <head> <title>nodeType Property Example</title> </head> <script> window.onload = function() { var ulElement = document.querySelector('ul'); var childNodes = ulElement.childNodes; // Display nodeType of each child node in the HTML var resultDiv = document.getElementById('result'); // Introductory paragraph var introParagraph = document.createElement('p'); introParagraph.textContent= 'Displays nodeType of each child node:'; resultDiv.appendChild(introParagraph); for (var i = 0; i < childNodes.length; i++) { var nodeType = childNodes[i].nodeType; var nodeTypeText = nodeType === Node.ELEMENT_NODE ? 'Element' : nodeType === Node.TEXT_NODE ? 'Text' : nodeType===Node.COMMENT_NODE?'Comment': nodeType === Node.DOCUMENT_NODE ? 'Document' : 'Other'; resultDiv.innerHTML+= '<p>Node Type of Child Node ' +(i+1)+':'+nodeTypeText + '</p>'; } }; </script> </head> <body> <h1>HTML - DOM Element</h1> <h2>nodeType Property</h2> <h4>Below are some Child Nodes :</h4> <ul> <li>Item 1</li> <!-- Comment --> <li>Item 2</li> Text Node between items <li>Item 3</li> </ul> <div id="result"></div> </body> </html>
显示有关第一个子节点的信息
此示例演示如何使用 HTML DOM 元素中的 'nodeType' 属性访问和显示元素的第一个子节点的信息。
<!DOCTYPE html> <html lang="en"> <head> <title>Node Information Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>nodeType Property</h2> <p>Displays the information about the first Child Node: </p> <div id="my"> <!-- First child node --> <p>Content of the first child node.</p> </div> <script> window.onload = function() { var divElement=document.getElementById('my'); var firstChild = divElement.firstChild; var nodeName = firstChild.nodeName; var nodeValue =firstChild.nodeValue||'N/A'; var nodeType = firstChild.nodeType; var resultDiv=document.getElementById('res'); resultDiv.innerHTML = '<p>Node Name: ' + nodeName + '</p>' + '<p>Node Value: ' + nodeValue + "Content of the first child node"+'</p>'+ '<p>Node Type: ' + nodeType + '</p>'; }; </script> <div id="res"></div> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
nodeType | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告