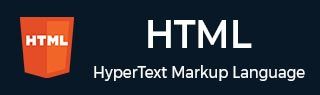
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头与标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮箱链接
- HTML 颜色名称与值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 多媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - QR 码
- HTML - Modernizr
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 outerHTML 属性
outerHTML 属性获取元素的完整 HTML 代码,包括其起始标签、属性、内容(包括子元素和文本内容)以及结束标签。
语法
设置 outerHTML 属性element.outerHTML = text;获取 outerHTML 属性
element.outerHTML;
属性值
值 | 描述 |
---|---|
文本 | 您要为元素设置的新 HTML 内容。 |
返回值
outerHTML 属性返回一个字符串,其中包含元素的 HTML 内容,包括其属性、起始标签和结束标签。
HTML DOM 元素 'outerHTML' 属性示例
以下是一些示例,演示了 'outerHTML' 属性的使用,以便更好地理解。
修改 div 元素内容
此示例演示了如何使用 outerHTML 属性来更新 HTML 元素的内容。最初,有一个包含 <div> 元素的 <p> 标签。单击按钮时,其函数会使用新的结构更新 <p> 标签的内容。
<!DOCTYPE html> <html> <body> <h1>HTML - DOM Element</h1> <h2>outerHTML Property</h2> <p>Click to change the div's content.</p> <div id="ex"> <p>Yes! I am a paragraph.</p> </div> <button onclick="changeOuterHTML()"> Change outerHTML </button> <script> function changeOuterHTML() { const divEle = document.getElementById('ex'); divEle.outerHTML= '<div id="newExample"> <p> Oops! You changed me. </p> </div>'; // Displaying the updated HTML content document.getElementById('result').innerHTML = "Updated outerHTML: " + divEle.outerHTML; } </script> <p id="result"></p> </body> </html>
使用 outerHTML 进行克隆
此示例演示如何使用 outerHTML 属性克隆元素。最初,单击按钮时,该函数返回 <div> 元素的 outerHTML。然后,它创建一个新的 <div> 元素,然后克隆原始 <div> 的整个结构。
<!DOCTYPE html> <html> <body> <h1>HTML - DOM Element</h1> <h2>outerHTML Property</h2> <p>This clones the div elements...</p> <div id="real"> <p>This is a paragraph in the source.</p> </div> <button onclick="cloneElement()"> Clone Element </button> <script> function cloneElement() { const sourceDiv =document.getElementById('real'); const clon = sourceDiv.outerHTML; // Creats new element same as HTML as the source const cloned = document.createElement('div'); cloned.innerHTML = clon; // Appending the cloned element to the body document.body.appendChild(cloned); } </script> </body> </html>
删除元素
此示例演示了如何使用 outerHTML 属性通过将 <div> 元素的 outerHTML 设置为空字符串 ('') 来从 HTML DOM 中删除特定的 <div> 元素。
<!DOCTYPE html> <html> <body> <h1>HTML - DOM Element</h1> <h2>outerHTML Property</h2> <p> Upon clicking the button it will remove the div element.... </p> <div id="ex"> <p>This paragraph will be removed.</p> </div> <button onclick="removeElement()"> Remove Element </button> <script> function removeElement() { const divEle = document.getElementById('ex'); divEle.outerHTML = ''; } </script> </body> </html>
根据用户输入呈现 HTML
此示例演示如何使用 outerHTML 属性根据用户输入呈现 HTML 内容。单击按钮时,该函数将 <div> 元素的 outerHTML 设置为用户提供的 HTML 内容。
<!DOCTYPE html> <html> <head> <title>outerHTML Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>outerHTML Property</h2> <p> This clones the div elements...</p> <p> <b>Note:</b> The attribute also get cloned. </p> <div id="real"> <p style="color: green;"> This is a paragraph in the source. </p> </div> <button onclick="cloneElement()"> Clone Element </button> <script> function cloneElement() { const sourceDiv =document.getElementById('real'); const clon = sourceDiv.outerHTML; // Creats new element same as HTML as the source const cloned = document.createElement('div'); cloned.innerHTML = clon; // Appending the cloned element to the body document.body.appendChild(cloned); } </script> </body> </html>
动态内容插入
此示例演示如何使用 outerHTML 属性将新内容插入 HTML 元素。单击按钮时,新 HTML 内容可以动态地插入到 DOM 结构中的特定位置。
<!DOCTYPE html> <html> <body> <h1>HTML - DOM Element</h1> <h2>outerHTML Property</h2> <p> Click the button to insert a new element before the existing content. </p> <div id="cont"> <p>This is the existing content.</p> </div> <button onclick="insertEle()"> Insert New Element </button> <script> function insertEle() { const contDiv = document.getElementById('cont'); const newEle= '<div id="newDiv"> <h3>New Element</h3> <p>This is a new paragraph.</p> </div>'; // Inserts new element before the existing content contDiv.insertAdjacentHTML('beforebegin', newEle); } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
outerHTML | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告