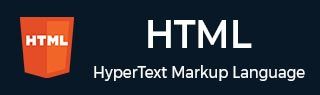
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内联框架
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题和说明
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - 头元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM querySelector() 方法
HTML DOM 的querySelector() 方法允许您选择并访问文档中与给定 CSS 选择器匹配的第一个 HTML 元素。
CSS 选择器用于查找或选择您想要设置样式或操作的文档中的元素。例如,您可以使用 ID、类名和各种其他选择器来定位特定元素。
语法
以下是 HTML DOM querySelector() 方法的语法:
element.querySelector(CSS_selectors);
参数
此方法接受如下所述的一个参数:
参数 | 描述 |
---|---|
CSS_selectors | 一个字符串,定义您要选择的元素类型。它可以是 ID、类、元素类型(如 <div> 或 <p>),甚至特定的属性及其值。 |
返回值
此方法返回与给定 CSS 选择器匹配的第一个 HTML 元素。如果未找到匹配的元素,则返回“null”。
示例 1:将 Highlight 类应用于选定元素
以下是 HTML DOM querySelector() 方法的基本示例。当单击按钮时,它会将“highlight”类添加到匹配的段落 (<p>) 元素:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelector() Method</title> <style> .highlight { background-color: lightblue; } button{ margin: 5px 0px; padding: 8px; } </style> </head> <body> <p>Selects the first element that matches the given CSS selector</p> <h4>This is a heading</h4> <p>This is a paragraph.</p> <p class="hi">This is another paragraph with class="hi".</p> <div>This is a div element.</div> <button onclick="applyHighlight()">Apply Highlight</button> <script> function applyHighlight() { // Selecting the element with class "hi" const hele = document.querySelector('.hi'); // Applying bold font weight and highlight hele.style.fontWeight = 'bold'; hele.classList.add('highlight'); // Creating a new paragraph to display the action const resp = document.createElement('p'); resp.textContent = 'Applied bold font weight to element with ' + 'class "highlight".'+ '.highlight'; document.body.appendChild(resp); } </script> </body> </html>
示例 2:选择并显示元素文本
此示例演示了querySelector() 方法的使用,方法是选择文档中的第一个 <p> 元素;然后它将该段落的文本内容显示为输出:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelector() Method</title> <style> .highlight { background-color: yellow; } button{ margin: 5px 0px; padding: 8px; } </style> </head> <body> <h1>This is a heading</h1> <p>This is a paragraph.</p> <p class="highlight">This is another paragraph with class="highlight"</p> <div>This is a div element.</div> <button onclick="showOutput()">Show Output</button> <script> function showOutput() { // Selecting the first <p> element const firstP = document.querySelector('p'); // Creating a new paragraph to display the result const resultP=document.createElement('p'); resultP.textContent = "Selected paragraph text: "+ "It selects the first element that matches " + "the specified CSS selector "; // Displaying the result in a clean way document.body.appendChild(resultP); } </script> </body> </html>
示例 3:选择和更改文本内容
在下面的示例中,querySelector() 方法选择一个 <div> 元素,并在单击按钮时使用新的文本内容对其进行修改:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelector() Method</title> <style> button{ margin: 5px 0px; padding: 8px; } </style> </head> <body> <p>Selecting and Changing Text Content</p> <div id="con">Original text content.</div> <button onclick="changeContent()">Change Content</button> <script> function changeContent() { const cele = document.querySelector('#con'); // Changing text content of selected element cele.textContent = 'Updated text content!'; // Creating a paragraph for displaying action const resp = document.createElement('p'); resp.textContent = 'Changed text content of element with ID "con".'; // Displaying the result in a clean way document.body.appendChild(resp); } </script> </body> </html>
示例 4:在选定元素上添加事件监听器
以下示例显示了如何使用querySelector() 方法选择一个元素并动态添加事件监听器:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelector() Method</title> <style> button{ margin: 5px 0px; padding: 8px; } </style> </head> <body> <p>Adding Event Listener on selected element...</p> <button id="myButton">Click Me!</button> <div id="output"></div> <script> document.addEventListener('DOMContentLoaded', function() { const b=document.querySelector('#myButton'); const op = document.querySelector('#output'); b.addEventListener('click', function() { output.innerHTML = ''; const msg = document.createElement('p'); msg.textContent = 'Button clicked!'; op.appendChild(msg); }); }); </script> </body> </html>
示例 5:选择和修改表单元素
此示例显示了querySelector() 方法如何选择表单元素并在输出面板中显示其值:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelector() Method</title> <style> button{ margin: 5px 0px; padding: 8px; } </style> </head> <body> <p>Selects the form elements and modifies their values...</p> <form id="myForm"> <label for="username">Username:</label> <input type="text" id="username" name="username"> <br><br> <label for="password">Password:</label> <input type="password" id="password" name="password"> <br><br> <button type="button"onclick="submitForm()">Submit Form</button> </form> <div id="output"></div> <script> function submitForm() { const form=document.querySelector('#myForm'); const uin = form.querySelector('#username'); const pin = form.querySelector('#password'); // Displaying form data in the output panel const op = document.querySelector('#output'); op.innerHTML = `<p>Username: ${uin.value}</p><p>Password: ${pin.value}</p>`; } </script> </body> </html>
示例 6:选择和修改选定的表格行
在以下示例中,使用 querySelector() 方法修改表格行。它将“highlight”类添加到由年龄最小的人选择的行:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelector() Method</title> <style> button{ margin: 5px 0px; padding: 8px; } .highlight { background-color: yellow; } </style> </head> <body> <p>Selects and adds highlight class on selected table row..</p> <table id="myTable" border="1"> <thead> <tr> <th>Name</th> <th>Age</th> </tr> </thead> <tbody> <tr> <td>John</td> <td>25</td> </tr> <tr> <td>Jane</td> <td>30</td> </tr> </tbody> </table> <button onclick="highlightYoungest()">Highlight Youngest</button> <script> function highlightYoungest() { const t=document.querySelector('#myTable'); const r = t.querySelectorAll('tbody tr'); // Finding the youngest person let youngestAge = Infinity; let youngestRow = null; r.forEach(row => { const age = parseInt(row.querySelector ('td:nth-child(2)').textContent); if (age < youngestAge) { youngestAge = age; youngestRow = row; } }); youngestRow.classList.add('highlight'); //creates new paragraph to display the result const rp = document.createElement('p'); rp.textContent="Highlighted the youngest " + "person (Age: ${youngestAge}) in the table."; // Displaying the in a clean way document.body.appendChild(rp); } </script> </body> </html>
示例 7:控制 Div 元素的可见性
下面的示例使用 querySelector() 方法控制元素的可见性。当单击按钮时,<div> 元素变为可见:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelector() Method</title> <style> .hidden { display: none; } button{ margin: 5px 0px; padding: 8px; } </style> </head> <body> <p>Controls the visibility of a <div> element...</p> <button onclick="toggleVisibility()">Control Visibility</button> <div id="myDiv"> <p>Click the button to hide me!</p> </div> <script> function toggleVisibility() { const d=document.querySelector('#myDiv'); d.classList.toggle('hidden'); } </script> </body> </html>
支持的浏览器
方法 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
querySelector() | 是 4.0 | 是 8.0 | 是 3.5 | 是 3.2 | 是 10.0 |
html_dom_element_reference.htm
广告