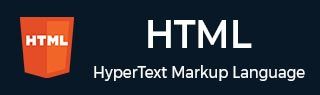
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内嵌框架 (Iframe)
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题与说明
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮箱链接
- HTML 颜色名称与值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM querySelectorAll() 方法
HTML DOM 的querySelectorAll()方法允许您选择和访问文档中与给定 CSS 选择器匹配的所有 HTML 元素。
CSS 选择器用于查找或选择文档中您想要设置样式或操作的元素。例如,您可以使用 ID、类名和各种其他选择器来定位特定元素。
语法
以下是 HTML DOM querySelectorAll() 函数的语法:
element.querySelectorAll(CSS_selectors);
参数
此方法接受如下所述的一个参数:
参数 | 描述 |
---|---|
CSS_selectors | 定义您想要选择的元素类型的字符串。它可以是 ID、类、元素类型(例如 <div> 或 <p>),甚至是特定的属性及其值。要定义多个选择器,请用逗号 (,) 分隔它们。 |
返回值
此方法返回一个 NodeList 对象,其中包含与给定 CSS 选择器匹配的元素集合。如果找不到匹配的元素,则返回一个空的“NodeList”。
示例 1:将样式应用于所有段落
以下是 HTML DOM querySelectorAll() 方法的基本示例。它将样式应用于文档中的所有段落 (<p>) 元素:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> <style> .highlight { background-color: lightblue; } </style> </head> <body> <p>Applying Style to All Paragraphs.</p> <p>Click the below button to add style</p> <p>Paragraph 1</p> <p>Paragraph 2</p> <p>Paragraph 3</p> <button onclick="applyHighlight()">Apply Highlight</button> <script> function applyHighlight() { const para=document.querySelectorAll('p'); para.forEach(para => { para.classList.add('highlight'); }); } </script> </body> </html>
示例 2:计数和显示列表项
此示例使用 querySelectorAll() 方法计算并显示无序列表中的列表项 (<li>) 的总数:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> </head> <body> <p>Click the button to see the count of List Items..</p> <ul id="myL"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <button onclick="countItems()">Count Items</button> <p id="totalItems"></p> <script> function countItems() { const i=document.querySelectorAll('#myL li'); document.getElementById('totalItems').textContent = `Total items: ${i.length}`; } </script> </body> </html>
示例 3:向所有元素添加事件监听器
此示例演示如何使用 querySelectorAll() 方法选择元素并动态地向所有元素添加事件监听器:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> </head> <body> <p>Adding Event Listener to all element<p> <button id="btn1">Button 1</button> <button id="btn2">Button 2</button> <button id="btn3">Button 3</button> <div id="output"></div> <script> const bn=document.querySelectorAll('button'); const opd = document.getElementById('output'); bn.forEach(button => { button.addEventListener('click', () => { const buttonId = button.id; const message = `Button ${buttonId} clicked`; // Display message in output const msgP=document.createElement('p'); msgP.textContent = message; opd.appendChild(msgP); }); }); </script> </body> </html>
示例 4:选择和修改选定的表格单元格
此示例演示如何使用 querySelectorAll() 方法选择和修改表格单元格。代码包含一个按钮,单击该按钮时,它会将突出显示类添加到所有表格单元格:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> <style> .highlight { background-color: violet; } </style> </head> <body> <p>Click button to apply style.</p> <table id="myT" border="1"> <tr> <td>Name</td> <td>Age</td> </tr> <tr> <td>John</td> <td>30</td> </tr> <tr> <td>Jane</td> <td>25</td> </tr> </table> <button onclick="highlightCells()">Highlight Cells</button> <script> function highlightCells() { const c=document.querySelectorAll('#myT td'); c.forEach(c => { c.classList.add('highlight'); }); } </script> </body> </html>
示例 5:筛选和设置特定元素的样式
此示例演示如何使用 querySelectorAll() 方法选择特定元素并根据其位置应用不同的样式:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> <style> .even { color: green; } .odd { color: blue; } </style> </head> <body> <p>Selects specific elements and applies style to each... </p> <ul id="MyL"> <li>1</li> <li>2</li> <li>3</li> <li>4</li> <li>5</li> </ul> <button onclick="styleNumbers()">Style Numbers</button> <script> function styleNumbers() { const i=document.querySelectorAll('#MyL li'); i.forEach((i, index) => { if ((index + 1) % 2 === 0) { i.classList.add('even'); } else { i.classList.add('odd'); } }); } </script> </body> </html>
示例 6:检查所有复选框的状态
此示例演示如何使用 querySelectorAll() 方法检查所有复选框的状态。代码包含附加到 <div> 元素的 <p> 元素,以显示每个复选框的状态:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> </head> <body> <p>Check the status of the following checkboxes</p> <p>Click the below button</p> <input type="checkbox" id="checkbox1"> <label for="checkbox1">Checkbox 1</label><br> <input type="checkbox" id="checkbox2"> <label for="checkbox2">Checkbox 2</label><br> <input type="checkbox" id="checkbox3"> <label for="checkbox3">Checkbox 3</label><br> <button onclick="checkStates()">Check States</button> <div id="checkboxStatus"></div> <script> function checkStates() { const checkboxes = document.querySelectorAll ('input[type="checkbox"]'); const checkboxStatusDiv=document.getElementById ('checkboxStatus'); checkboxStatusDiv.innerHTML = ''; checkboxes.forEach(checkbox => { const checkboxState = checkbox.checked ? 'checked' : 'not checked'; const checkboxStatus = document.createElement('p'); checkboxStatus.textContent = `${checkbox.id} is ${checkboxState}.`; checkboxStatusDiv.appendChild (checkboxStatus); }); } </script> </body> </html>
示例 7:选择和设置“ul”元素的样式
此示例演示如何使用 querySelectorAll() 方法选择和设置多个元素的样式。代码的目标是 <ul> 元素,并将突出显示类应用于其所有子 <li> 元素:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> <style> .highlight { background-color: violet; } </style> </head> <body> <p>Click button to highlight the <ul> elements... </p> <ul id="myL"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <button onclick="applyHighlight()">Apply Highlight</button> <script> function applyHighlight() { const i=document.querySelectorAll('#myL li'); i.forEach(i => { i.classList.add('highlight'); }); } </script> </body> </html>
示例 8:更新所有“li”项的文本
此示例演示如何使用 querySelectorAll() 方法更改与 CSS 选择器匹配的所有元素的文本内容:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM querySelectorAll() Method</title> </head> <body> <p>Change the text of all elements by clicking the below button.. </p> <ul id="mL"> <li>Chota</li> <li>Mighty</li> <li>Little</li> </ul> <button onclick="changeText()">Change Text</button> <div id="output"></div> <script> function changeText() { const i=document.querySelectorAll('#mL li'); const names = ['Chota Bheem','Mighty Raju','Little Krishna']; const opd = document.getElementById('output'); opd.innerHTML = ''; i.forEach((item, index) => { item.textContent = names[index]; // Display updated text in output const newip=document.createElement('p'); newip.textContent = `Changed text to: ${names[index]}`; opd.appendChild(newip); }); } </script> </body> </html>
支持的浏览器
方法 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
querySelectorAll() | 是 | 是 | 是 | 是 | 是 |