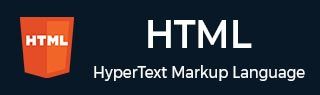
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内联框架 (iframe)
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格列组 (colgroup)
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 多媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - head 元素
- HTML - 添加 Favicon
- HTML - JavaScript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - WebRTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizr
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM style 属性
HTML DOM 的style 属性用于获取直接为特定元素设置的 CSS 样式。
它可以访问该特定元素的内联 CSS 属性,不包括 <head> 部分或任何外部样式表中的样式。
语法
以下是 HTML DOM 的style(设置样式)属性的语法:
element.style.propertyName = value;
其中,“value”是您想要应用于特定元素 CSS 属性的新值。
要获取已使用的样式属性,请使用以下语法:
element.style.propertyName;
参数
此属性不接受任何参数。
返回值
style 属性返回一个对象,该对象包含应用于特定元素的内联 CSS 样式。
示例 1:将内联样式应用于段落元素
下面的程序演示了如何使用 HTML DOM 的style 属性将样式应用于段落 (<p>) 元素:
<!DOCTYPE html> <html lang="en"> <head> <style> .highlighted { background-color: lightblue; padding: 10px; border: 1px solid blue; } button{ padding: 8px 10px; } </style> </head> <body> <p id="myPara">Click the below button to change my style!</p> <button onclick="changeStyle()">Change Style</button> <script> function changeStyle() { // Get the paragraph element let para = document.getElementById('myPara'); // Apply new styles using the style property para.style.backgroundColor ='green'; para.style.color = 'white'; para.style.fontWeight = 'bold'; para.style.padding = '15px'; para.innerHTML = 'Style Changed!'; } </script> </body> </html>
上面的程序在单击按钮时将样式添加到段落 (“p”) 元素。
示例 2:每次单击时更改段落的颜色
以下是 HTML DOM style 属性的另一个示例。我们使用此属性在单击按钮时更新段落 (“p”) 元素的文本颜色:<!DOCTYPE html> <html lang="en"> <head> <style> #colorfulText { font-size: 24px; font-weight: bold; margin-top: 20px; } button{ padding: 8px 10px; } </style> </head> <body> <p>Click the below to update the style..</p> <p id="colorfulText">Click the button to see colorful text!</p> <button onclick="changeColor()">Generate Colorful Text</button> <script> function changeColor() { let t=document.getElementById('colorfulText'); let col= ['red','green','blue','orange','purple']; let randomColor = col[Math.floor(Math.random() * col.length)]; t.style.color = randomColor; t.innerHTML = `Colorful Text in ${randomColor.toUpperCase()}`; } </script> </body> </html>
上面的程序每次单击按钮时都会向段落添加新的颜色。
示例 3:将高亮效果应用于按钮元素
在下面的示例中,DOM style 属性用于通过在单击时将高亮效果应用于按钮来更改元素的外观:
<!DOCTYPE html> <html lang="en"> <head> <style> button{ padding: 8px 10px; cursor: pointer; } </style> </head> <body> <p>Click the below button to add a highlight effect to it.<p> <button id="myButton">Click to Highlight</button> <script> let bn = document.getElementById('myButton'); //Add event listener to the button bn.addEventListener('click', function() { // Change the background color using style property bn.style.backgroundColor = 'green'; bn.style.color = 'white'; bn.style.border = 'none'; bn.innerHTML = 'Highlighted!'; }); </script> </body> </html>
执行上述程序后,将显示一个按钮,单击该按钮时,将向该按钮添加样式。
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
style | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告