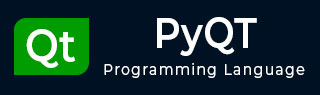
- PyQt 教程
- PyQt - 首页
- PyQt - 简介
- PyQt - 环境配置
- PyQt - Hello World
- PyQt - 主要类
- PyQt - 使用 Qt Designer
- PyQt - 元对象
- PyQt 信号与槽
- PyQt - 信号和槽
- PyQt - 支持和信号
- PyQt - 未绑定和已绑定信号
- PyQt - 使用 PyQtSignal 创建新的信号
- PyQt - 连接、断开和发射信号
- PyQt - 槽装饰器
- PyQt - 槽连接
- PyQt 布局
- PyQt - 布局管理
- PyQt - QBoxLayout
- PyQt - QGridLayout
- PyQt - QFormLayout
- PyQt - QHBoxLayout
- PyQt - QVBoxLayout
- PyQt - QStackedLayout
- PyQt - QGraphicsGridLayout
- PyQt - QGraphicsAnchorLayout
- PyQt - QGraphicsLayout
- PyQt - QGraphicsLinearLayout
- PyQt 基本组件
- PyQt - 基本组件
- PyQt - QLabel 组件
- PyQt - QLineEdit 组件
- PyQt - QPushButton 组件
- PyQt - QRadioButton 组件
- PyQt - QCheckBox 组件
- PyQt - QComboBox 组件
- PyQt - QSpinBox 组件
- PyQt - QMessageBox
- PyQt - QDialogButtonBox 组件
- PyQt - QFontComboBox 组件
- PyQt - QDoubleSpinBox 组件
- PyQt - QToolBox 组件
- PyQt - QDialog 类
- PyQt - QMessageBox
- PyQt - 多文档界面
- PyQt - 拖放
- PyQt 绘图 API
- PyQt - 绘图 API
- PyQt 数据库
- PyQt - 数据库处理
- PyQt 核心要点
- PyQt - BrushStyle 常量
- PyQt - QClipboard
- PyQt - QPixmap 类
- PyQt 有用资源
- PyQt - 快速指南
- PyQt - 有用资源
- PyQt - 讨论
PyQt - QDialogButtonBox 组件
QDialogButtonBox
工具简化了在对话框中添加按钮的过程。它简化了创建对话框按钮设置(如“确定”、“取消”、“应用”等)的过程。使用此功能,开发人员可以跨平台维护按钮位置和操作。
QDialogButtonBox 中使用的方法
以下是 QDialogButtonBox 组件的一些常用方法及其描述:
方法 | 描述 |
---|---|
addButton() | 向按钮框添加一个按钮。 |
button() | 返回与指定角色关联的按钮。 |
setStandardButtons() | 设置要添加到按钮框的标准按钮。 |
standardButton() | 返回被点击的标准按钮。 |
示例 1:创建简单的 QDialogButtonBox
在这个例子中,我们使用 QDialogButtonBox.StandardButton.Ok | QDialogButtonBox.StandardButton.Cancel 参数创建一个带有“确定”和“取消”按钮的 **QDialogButtonBox**。我们将按钮框的 accepted 和 rejected 信号分别连接到对话框的 accept 和 reject 槽。
import sys from PyQt6.QtWidgets import QApplication, QDialog, QDialogButtonBox, QVBoxLayout class Dialog(QDialog): def __init__(self): super().__init__() self.initUI() def initUI(self): layout = QVBoxLayout() buttonBox = QDialogButtonBox(QDialogButtonBox.StandardButton.Ok | QDialogButtonBox.StandardButton.Cancel) buttonBox.accepted.connect(self.accept) buttonBox.rejected.connect(self.reject) layout.addWidget(buttonBox) self.setLayout(layout) if __name__ == '__main__': app = QApplication(sys.argv) dialog = Dialog() dialog.exec() sys.exit(app.exec())
输出
以上代码产生以下输出:
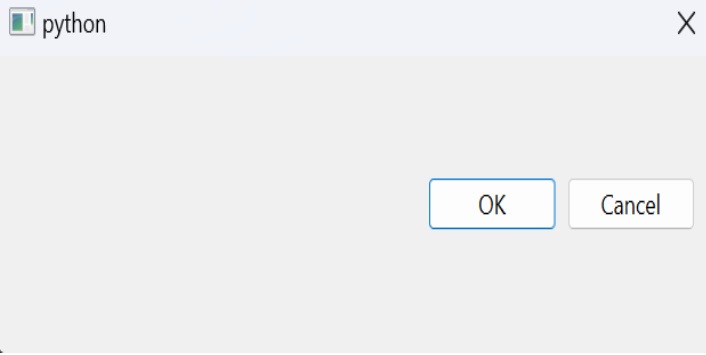
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 2:使用标准按钮
在这个例子中,我们创建一个 **QDialogButtonBox** 并使用 setStandardButtons() 方法设置标准按钮(保存、取消和放弃)。我们将按钮框的 clicked 信号连接到自定义槽 buttonClicked,该槽使用 **standardButton()** 识别被点击的按钮,并根据按钮的角色执行特定操作。
import sys from PyQt6.QtWidgets import QApplication, QDialog, QDialogButtonBox, QVBoxLayout class Dialog(QDialog): def __init__(self): super().__init__() self.initUI() def initUI(self): layout = QVBoxLayout() buttonBox = QDialogButtonBox() buttonBox.setStandardButtons(QDialogButtonBox.StandardButton.Save | QDialogButtonBox.StandardButton.Cancel | QDialogButtonBox.StandardButton.Discard) buttonBox.clicked.connect(self.buttonClicked) layout.addWidget(buttonBox) self.setLayout(layout) def buttonClicked(self, button): role = buttonBox.standardButton(button) if role == QDialogButtonBox.Save: print("Save clicked") elif role == QDialogButtonBox.Cancel: print("Cancel clicked") elif role == QDialogButtonBox.Discard: print("Discard clicked") if __name__ == '__main__': app = QApplication(sys.argv) dialog = Dialog() dialog.exec() sys.exit(app.exec())
输出
以上代码产生以下输出:
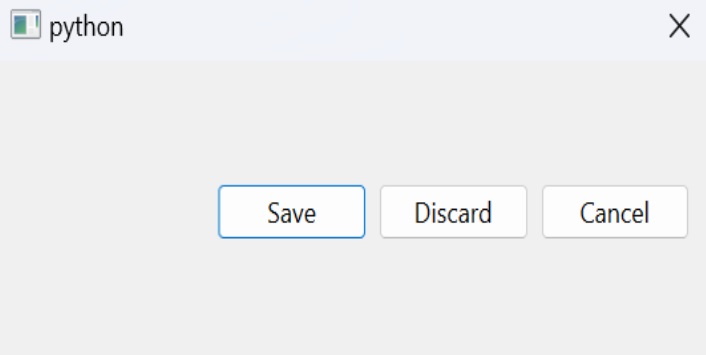
广告