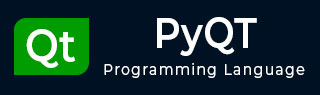
- PyQt 教程
- PyQt - 首页
- PyQt - 简介
- PyQt - 环境配置
- PyQt - Hello World
- PyQt - 主要类
- PyQt - 使用 Qt Designer
- PyQt - 元对象
- PyQt 信号与槽
- PyQt - 信号和槽
- PyQt - 支持和信号
- PyQt - 未绑定和已绑定信号
- PyQt - 使用 PyQtSignal 创建新信号
- PyQt - 连接、断开和发射信号
- PyQt - 槽装饰器
- PyQt - 槽连接
- PyQt 布局
- PyQt - 布局管理
- PyQt - QBoxLayout
- PyQt - QGridLayout
- PyQt - QFormLayout
- PyQt - QHBoxLayout
- PyQt - QVBoxLayout
- PyQt - QStackedLayout
- PyQt - QGraphicsGridLayout
- PyQt - QGraphicsAnchorLayout
- PyQt - QGraphicsLayout
- PyQt - QGraphicsLinearLayout
- PyQt 基本控件
- PyQt - 基本控件
- PyQt - QLabel 控件
- PyQt - QLineEdit 控件
- PyQt - QPushButton 控件
- PyQt - QRadioButton 控件
- PyQt - QCheckBox 控件
- PyQt - QComboBox 控件
- PyQt - QSpinBox 控件
- PyQt - QMessageBox
- PyQt - QDialogButtonBox 控件
- PyQt - QFontComboBox 控件
- PyQt - QDoubleSpinBox 控件
- PyQt - QToolBox 控件
- PyQt - QDialog 类
- PyQt - QMessageBox
- PyQt - 多文档界面
- PyQt - 拖放操作
- PyQt 绘图 API
- PyQt - 绘图 API
- PyQt 数据库
- PyQt - 数据库处理
- PyQt 基础知识
- PyQt - BrushStyle 常量
- PyQt - QClipboard
- PyQt - QPixmap 类
- PyQt 有用资源
- PyQt - 快速指南
- PyQt - 有用资源
- PyQt - 讨论
PyQt - QFontComboBox 控件
QFontComboBox 控件是一个组合框,用于在 PyQt 应用程序中选择字体系列。它允许用户从按字母顺序排列的字体系列名称列表中进行选择。一些字体系列名称包括 Arial、Helvetica 和 Times New Roman 等。
继承关系
QFontComboBox 类继承其核心功能自 QComboBox 类,而 QComboBox 类又继承自 QWidgets 类。

QFontComboBox 的主要特性
- 字体系列选择 - 使用字体系列选择,用户可以轻松地从组合框中提供的按字母顺序排列的列表中选择字体系列。
- 视觉表示 - 尽可能使用字体的实际样式来显示字体,这有助于用户直观地识别他们喜欢的字体。
- 自定义 - 我们可以根据某些标准(如可伸缩性和书写系统)过滤字体。
- 集成 - 它通常与其他与字体相关的控件(如字体大小选择器和样式切换)一起集成到工具栏中。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 1:基本的 QFontComboBox 实现
在这个示例中,创建了一个简单的 PyQt 应用程序,并在主窗口中添加了一个 QFontComboBox 控件。运行应用程序后,用户可以交互式地从组合框中选择字体系列。
这里,initUI 方法通过设置 QVBoxLayout 来初始化用户界面,以垂直排列控件,将 QFontComboBox 添加到布局中,并将窗口标题设置为 'Font Selector'。最后,使用 QApplication 执行应用程序,显示窗口并在应用程序退出之前处理事件。
import sys from PyQt6.QtWidgets import QApplication, QWidget, QVBoxLayout, QFontComboBox class FontSelector(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): layout = QVBoxLayout() font_combo = QFontComboBox() layout.addWidget(font_combo) self.setLayout(layout) self.setWindowTitle('Font Selector') if __name__ == '__main__': app = QApplication(sys.argv) window = FontSelector() window.show() sys.exit(app.exec())
输出结果
以上代码产生以下结果:

示例 2:使用 QFontComboBox 过滤字体
在下面的示例中,我们将创建一个字体选择器,它只显示可缩放的字体。当我们从组合框中选择一个字体时,下面的标签将更新以显示所选的字体系列。
在一个 FontSelector QWidget 中,一个 QVBoxLayout 布局排列控件。一个 QFontComboBox,font_combo,由于 setFontFilters() 而只显示可缩放的字体。一个 QLabel,font_label,当字体选择发生变化时,通过 update_label() 更新以显示所选的字体系列。
import sys from PyQt6.QtWidgets import QApplication, QWidget, QVBoxLayout, QFontComboBox, QLabel class FontSelector(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): layout = QVBoxLayout() # Create QFontComboBox font_combo = QFontComboBox() # Set font filters to show only scalable fonts font_combo.setFontFilters(QFontComboBox.FontFilter.ScalableFonts) # Create a label to display the selected font self.font_label = QLabel("Selected Font") # Connect fontChanged signal to update_label slot font_combo.currentFontChanged.connect(self.update_label) layout.addWidget(font_combo) layout.addWidget(self.font_label) self.setLayout(layout) self.setWindowTitle('Font Selector') def update_label(self, font): # Update the label text with the selected font family self.font_label.setText(f"Selected Font: {font.family()}") if __name__ == '__main__': app = QApplication(sys.argv) window = FontSelector() window.show() sys.exit(app.exec())
输出结果
以上代码产生以下结果:
