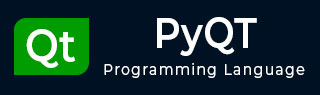
- PyQt 教程
- PyQt - 首页
- PyQt - 简介
- PyQt - 环境搭建
- PyQt - Hello World
- PyQt - 主要类
- PyQt - 使用 Qt Designer
- PyQt - 元对象
- PyQt 信号与槽
- PyQt - 信号和槽
- PyQt - 支持和信号
- PyQt - 未绑定和已绑定信号
- PyQt - 使用 PyQtSignal 创建新的信号
- PyQt - 连接、断开和发射信号
- PyQt - 槽装饰器
- PyQt - 槽连接
- PyQt 布局
- PyQt - 布局管理
- PyQt - QBoxLayout
- PyQt - QGridLayout
- PyQt - QFormLayout
- PyQt - QHBoxLayout
- PyQt - QVBoxLayout
- PyQt - QStackedLayout
- PyQt - QGraphicsGridLayout
- PyQt - QGraphicsAnchorLayout
- PyQt - QGraphicsLayout
- PyQt - QGraphicsLinearLayout
- PyQt 基本组件
- PyQt - 基本部件
- PyQt - QLabel 组件
- PyQt - QLineEdit 组件
- PyQt - QPushButton 组件
- PyQt - QRadioButton 组件
- PyQt - QCheckBox 组件
- PyQt - QComboBox 组件
- PyQt - QSpinBox 组件
- PyQt - QMessageBox
- PyQt - QDialogButtonBox 组件
- PyQt - QFontComboBox 组件
- PyQt - QDoubleSpinBox 组件
- PyQt - QToolBox 组件
- PyQt - QDialog 类
- PyQt - QMessageBox
- PyQt - 多文档界面
- PyQt - 拖放
- PyQt 绘图 API
- PyQt - 绘图 API
- PyQt 数据库
- PyQt - 数据库处理
- PyQt 核心知识
- PyQt - BrushStyle 常量
- PyQt - QClipboard
- PyQt - QPixmap 类
- PyQt 有用资源
- PyQt - 快速指南
- PyQt - 有用资源
- PyQt - 讨论
PyQt - QDoubleSpinBox 组件
PyQt 中的 QDoubleSpinBox 组件用于输入指定范围内的浮点数。它继承自 QAbstractSpinBox,后者又继承自 Qwidgets。它支持各种功能,包括键盘和鼠标交互、验证和舍入行为。
QDoubleSpinBox 的主要特性
- 精度控制 − 开发者可以指定要显示的小数位数。这提供了对浮点数精度的控制。
- 范围指定 − 可以设置允许的最小值和最大值,定义用户输入的理想范围。
- 键盘和鼠标交互 − 用户可以使用键盘箭头键或鼠标滚轮与 QDoubleSpinBox 交互,以增加或减少值。
- 信号和槽 − PyQt6 提供了一个信号 (valueChanged),可以将其连接到一个槽,以便在值更改时执行操作。
- 本地化数字显示 − QDoubleSpinBox 使用区域设置,确保根据用户的区域设置显示小数分隔符和分组字符。
设置最大值
要设置 QDoubleSpinBox 中的最大值,可以使用 setMaximum() 方法。此方法接受单个参数 – 您希望允许用户输入的最大值。通过设置最大值,您可以限制用户输入或选择超过指定限制的值。
语法
语法如下:
your_double_spin_box.setMaximum(max_value)
这里,your_double_spin_box 应替换为您实际的 QDoubleSpinBox 对象名称,而 max_value 应为所需的最大值。
示例 1:QDoubleSpinBox 的基本实现
在下面的示例中,我们创建了 PyQt6 应用程序的基本实现,其中包含 QDoubleSpinBox 组件和 QLabel。QDoubleSpinBox 允许用户在 0 到 100 范围内选择浮点数值,并且所选值将显示在 QLabel 中。
import sys from PyQt6.QtWidgets import QApplication, QMainWindow, QDoubleSpinBox, QLabel, QVBoxLayout, QWidget class MainWindow(QMainWindow): def __init__(self): super().__init__() self.init_ui() def init_ui(self): central_widget = QWidget(self) self.setCentralWidget(central_widget) layout = QVBoxLayout() self.double_spin_box = QDoubleSpinBox(self) self.double_spin_box.setMinimum(0) self.double_spin_box.setMaximum(100) # Setting the maximum value to 100 self.label = QLabel(self) self.label.setText("Selected value: ") layout.addWidget(self.double_spin_box) layout.addWidget(self.label) central_widget.setLayout(layout) self.double_spin_box.valueChanged.connect(self.update_label) def update_label(self, value): self.label.setText(f"Selected value: {value}") if __name__ == "__main__": app = QApplication(sys.argv) window = MainWindow() window.show() sys.exit(app.exec())
输出
以上代码产生以下结果:
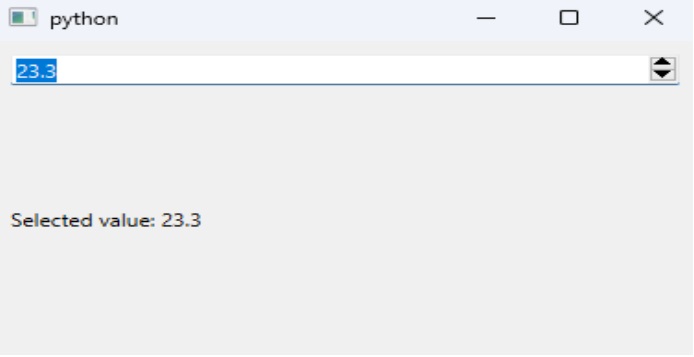
示例 2:自定义最大值
在下面的示例中,QDoubleSpinBox 的最大值自定义为 50,允许用户在 -50 到 50 范围内选择值。与示例 1 类似,所选值显示在 QLabel 中。
import sys from PyQt6.QtWidgets import QApplication, QMainWindow, QDoubleSpinBox, QLabel, QVBoxLayout, QWidget class MainWindow(QMainWindow): def __init__(self): super().__init__() self.init_ui() def init_ui(self): central_widget = QWidget(self) self.setCentralWidget(central_widget) layout = QVBoxLayout() self.double_spin_box = QDoubleSpinBox(self) self.double_spin_box.setMinimum(-50) self.double_spin_box.setMaximum(50) # Setting the maximum value to 50 self.label = QLabel(self) self.label.setText("Selected value: ") layout.addWidget(self.double_spin_box) layout.addWidget(self.label) central_widget.setLayout(layout) self.double_spin_box.valueChanged.connect(self.update_label) def update_label(self, value): self.label.setText(f"Selected value: {value}") if __name__ == "__main__": app = QApplication(sys.argv) window = MainWindow() window.show() sys.exit(app.exec())
输出
执行以上代码后,我们将得到以下结果:
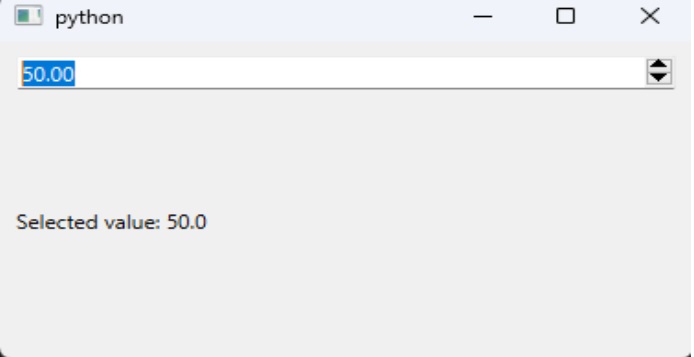
示例 3:处理大范围
在下面的示例中,我们展示了 QDoubleSpinBox 通过将最大值设置为 1000 来处理更大范围的能力。用户可以在 0 到 1000 范围内选择值,并且所选值会在 QLabel 中实时更新。
import sys from PyQt6.QtWidgets import QApplication, QMainWindow, QDoubleSpinBox, QLabel, QVBoxLayout, QWidget class MainWindow(QMainWindow): def __init__(self): super().__init__() self.init_ui() def init_ui(self): central_widget = QWidget(self) self.setCentralWidget(central_widget) layout = QVBoxLayout() self.double_spin_box = QDoubleSpinBox(self) self.double_spin_box.setMinimum(0) self.double_spin_box.setMaximum(1000) # Setting the maximum value to 1000 self.label = QLabel(self) self.label.setText("Selected value: ") layout.addWidget(self.double_spin_box) layout.addWidget(self.label) central_widget.setLayout(layout) self.double_spin_box.valueChanged.connect(self.update_label) def update_label(self, value): self.label.setText(f"Selected value: {value}") if __name__ == "__main__": app = QApplication(sys.argv) window = MainWindow() window.show() sys.exit(app.exec())
输出
执行以上代码后,我们将得到以下结果:
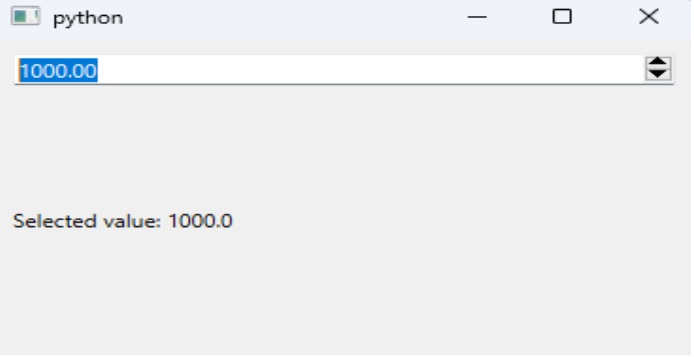
广告