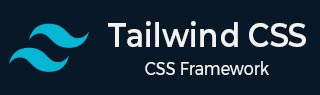
- Tailwind CSS 教程
- Tailwind CSS - 首页
- Tailwind CSS - 路线图
- Tailwind CSS - 简介
- Tailwind CSS - 安装
- Tailwind CSS - 编辑器设置
- Tailwind CSS - 核心概念
- Tailwind CSS - 实用优先基础
- Tailwind CSS - 悬停、焦点和其他状态
- Tailwind CSS - 响应式设计
- Tailwind CSS - 暗黑模式
- Tailwind CSS - 重用样式
- Tailwind CSS - 添加自定义样式
- Tailwind CSS - 函数和指令
- Tailwind CSS - 自定义
- Tailwind CSS - 配置
- Tailwind CSS - 内容配置
- Tailwind CSS - 主题配置
- Tailwind CSS - 自定义屏幕尺寸
- Tailwind CSS - 自定义颜色
- Tailwind CSS - 自定义间距
- Tailwind CSS - 插件
- Tailwind CSS - 预设
- Tailwind CSS - 基础样式
- Tailwind CSS - 预处理
- Tailwind CSS - 布局
- Tailwind CSS - 高宽比
- Tailwind CSS - 容器
- Tailwind CSS - 列
- Tailwind CSS - 分栏后
- Tailwind CSS - 分栏前
- Tailwind CSS - 分栏内
- Tailwind CSS - 边框装饰断开
- Tailwind CSS - 盒子尺寸
- Tailwind CSS - 显示
- Tailwind CSS - 浮动
- Tailwind CSS - 清除浮动
- Tailwind CSS - 隔离
- Tailwind CSS - 对象适应
- Tailwind CSS - 对象位置
- Tailwind CSS - 溢出
- Tailwind CSS - 滚动行为
- Tailwind CSS - 定位
- Tailwind CSS - 上/右/下/左
- Tailwind CSS - 可见性
- Tailwind CSS - Z 轴索引
- Tailwind CSS - Flexbox 和 Grid
- Tailwind CSS - Flex 基准
- Tailwind CSS - Flex 方向
- Tailwind CSS - Flex 换行
- Tailwind CSS - Flex
- Tailwind CSS - Flex 伸展
- Tailwind CSS - Flex 压缩
- Tailwind CSS - 顺序
- Tailwind CSS - 网格模板列
- Tailwind CSS - 网格列开始/结束
- Tailwind CSS - 网格模板行
- Tailwind CSS - 网格行开始/结束
- Tailwind CSS - 网格自动填充
- Tailwind CSS - 网格自动列
- Tailwind CSS - 网格自动行
- Tailwind CSS - 间隙
- Tailwind CSS - 内容对齐
- Tailwind CSS - 项目对齐
- Tailwind CSS - 自身对齐
- Tailwind CSS - 内容排列
- Tailwind CSS - 项目排列
- Tailwind CSS - 自身排列
- Tailwind CSS - 内容放置
- Tailwind CSS - 项目放置
- Tailwind CSS - 自身放置
- Tailwind CSS - 间距
- Tailwind CSS - 内边距
- Tailwind CSS - 外边距
- Tailwind CSS - 元素间距
- Tailwind CSS - 尺寸
- Tailwind CSS - 宽度
- Tailwind CSS - 最小宽度
- Tailwind CSS - 最大宽度
- Tailwind CSS - 高度
- Tailwind CSS - 最小高度
- Tailwind CSS - 最大高度
- Tailwind CSS - 尺寸
- Tailwind CSS - 排版
- Tailwind CSS - 字体系列
- Tailwind CSS - 字体大小
- Tailwind CSS - 字体平滑
- Tailwind CSS - 字体样式
- Tailwind CSS - 字体粗细
- Tailwind CSS - 数字字体变体
- Tailwind CSS - 字间距
- Tailwind CSS - 行限制
- Tailwind CSS - 行高
- Tailwind CSS - 列表样式图像
- Tailwind CSS - 列表样式位置
- Tailwind CSS - 列表样式类型
- Tailwind CSS - 文本对齐
- Tailwind CSS - 文本颜色
- Tailwind CSS - 文本装饰
- Tailwind CSS - 文本装饰颜色
- Tailwind CSS - 文本装饰样式
- Tailwind CSS - 文本装饰粗细
- Tailwind CSS - 文本下划线偏移
- Tailwind CSS - 文本转换
- Tailwind CSS - 文本溢出
- Tailwind CSS - 文本换行
- Tailwind CSS - 文本缩进
- Tailwind CSS - 垂直对齐
- Tailwind CSS - 空格
- Tailwind CSS - 断词
- Tailwind CSS - 连字符
- Tailwind CSS - 内容
- Tailwind CSS - 背景
- Tailwind CSS - 背景附件
- Tailwind CSS - 背景裁剪
- Tailwind CSS - 背景颜色
- Tailwind CSS - 背景原点
- Tailwind CSS - 背景位置
- Tailwind CSS - 背景重复
- Tailwind CSS - 背景尺寸
- Tailwind CSS - 背景图像
- Tailwind CSS - 渐变颜色停止
- Tailwind CSS - 边框
- Tailwind CSS - 圆角
- Tailwind CSS - 边框宽度
- Tailwind CSS - 边框颜色
- Tailwind CSS - 边框样式
- Tailwind CSS - 分割线宽度
- Tailwind CSS - 分割线颜色
- Tailwind CSS - 分割线样式
- Tailwind CSS - 轮廓宽度
- Tailwind CSS - 轮廓颜色
- Tailwind CSS - 轮廓样式
- Tailwind CSS - 轮廓偏移
- Tailwind CSS - 环宽度
- Tailwind CSS - 环颜色
- Tailwind CSS - 环偏移宽度
- Tailwind CSS - 环偏移颜色
- Tailwind CSS - 效果
- Tailwind CSS - 盒子阴影
- Tailwind CSS - 盒子阴影颜色
- Tailwind CSS - 不透明度
- Tailwind CSS - 混合模式
- Tailwind CSS - 背景混合模式
- Tailwind CSS - 过滤器
- Tailwind CSS - 模糊
- Tailwind CSS - 亮度
- Tailwind CSS - 对比度
- Tailwind CSS - 投影
- Tailwind CSS - 灰度
- Tailwind CSS - 色相旋转
- Tailwind CSS - 反色
- Tailwind CSS - 饱和度
- Tailwind CSS - 棕褐色
- Tailwind CSS - 背景模糊
- Tailwind CSS - 背景亮度
- Tailwind CSS - 背景对比度
- Tailwind CSS - 背景灰度
- Tailwind CSS - 背景色相旋转
- Tailwind CSS - 背景反色
- Tailwind CSS - 背景不透明度
- Tailwind CSS - 背景饱和度
- Tailwind CSS - 背景棕褐色
- Tailwind CSS - 表格
- Tailwind CSS - 边框折叠
- Tailwind CSS - 边框间距
- Tailwind CSS - 表格布局
- Tailwind CSS - 标题位置
- Tailwind CSS - 过渡和动画
- Tailwind CSS - 过渡属性
- Tailwind CSS - 过渡时长
- Tailwind CSS - 过渡时间函数
- Tailwind CSS - 过渡延迟
- Tailwind CSS - 动画
- Tailwind CSS - 变换
- Tailwind CSS - 缩放
- Tailwind CSS - 旋转
- Tailwind CSS - 平移
- Tailwind CSS - 倾斜
- Tailwind CSS - 变换原点
- Tailwind CSS - 交互性
- Tailwind CSS - 口音颜色
- Tailwind CSS - 外观
- Tailwind CSS - 光标
- Tailwind CSS - 插入符号颜色
- Tailwind CSS - 指针事件
- Tailwind CSS - 调整大小
- Tailwind CSS - 滚动行为
- Tailwind CSS - 滚动边距
- Tailwind CSS - 滚动填充
- Tailwind CSS - 滚动捕捉对齐
- Tailwind CSS - 滚动捕捉停止
- Tailwind CSS - 滚动捕捉类型
- Tailwind CSS - 触摸操作
- Tailwind CSS - 用户选择
- Tailwind CSS - 将更改
- Tailwind CSS - SVG
- Tailwind CSS - 填充
- Tailwind CSS - 描边
- Tailwind CSS - 描边宽度
- Tailwind CSS - 可访问性
- Tailwind CSS - 屏幕阅读器
- Tailwind CSS - 强制颜色调整
- Tailwind CSS - 附加
- Tailwind CSS - 与预处理器一起使用
- Tailwind CSS - 生产优化
- Tailwind CSS - 参考
- Tailwind CSS - 核心概念
- Tailwind CSS - 自定义
- Tailwind CSS - 布局
- Tailwind CSS - Flexbox 和 Grid
- Tailwind CSS - 间距
- Tailwind CSS - 尺寸
- Tailwind CSS - 排版
- Tailwind CSS - 背景
- Tailwind CSS - 边框
- Tailwind CSS - 效果
- Tailwind CSS - 过滤器
- Tailwind CSS - 表格
- Tailwind CSS - 过渡和动画
- Tailwind CSS - 转换
- Tailwind CSS - 交互性
- Tailwind CSS - 资源
- Tailwind CSS - 讨论
- Tailwind CSS - 有用资源
Tailwind CSS - 内容配置
Tailwind CSS 内容配置指定了项目的源文件。'tailwind.config.js' 文件的 Content 部分指定了所有 HTML 模板、Javascript 组件以及包含 Tailwind 类名的任何其他源文件。
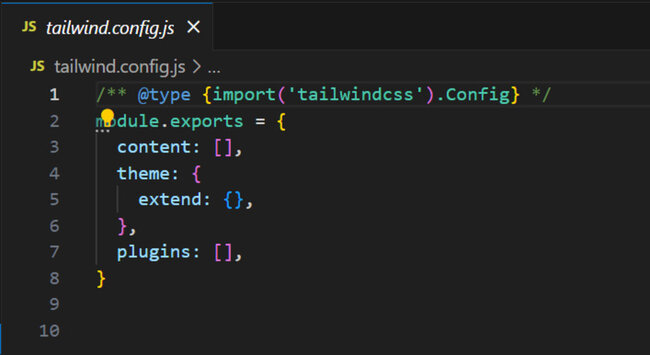
内容源路径配置
'tailwind.config.js' 文件的 content 部分中的源路径配置有助于 Tailwind CSS 扫描所有 HTML、Javascript 组件以及任何包含类名的文件,以生成这些样式的相应 CSS。
/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './pages/**/*.{html,js}', './components/**/*.{html,js}' ], // ... }
关键点
在 Tailwind CSS 中配置内容时需要记住的关键点。
- 使用通配符模式(**/*)递归匹配文件。
- 使用{}和逗号分隔的值来匹配选项列表,例如 {html,js}。
- 保持路径相对于项目根目录。
内容配置模式技巧
为了有效地配置内容,请遵循以下技巧
- 精确:排除捕获不必要文件或目录(例如 node_modules)的广泛模式。
content: [ './components/**/*.{html,js}', './pages/**/*.{html,js}', './index.html', // Include specific files if needed ],
content: [ './public/index.html', './src/**/*.{html,js}', ],
content: [ './src/**/*.js', ],
content: [ './src/**/*.css', ],
深度类识别
Tailwind 使用正则表达式从源代码中提取潜在的类名,而无需解析或执行它。
<div class="md:flex"> <div class="md:flex-shrink-0"> <img class="rounded-lg md:w-56" src="/img/shopping.jpg" alt="Woman paying for a purchase"> </div> <div class="mt-4 md:mt-0 md:ml-6"> <div class="uppercase tracking-wide text-sm text-indigo-600 font-bold"> Marketing </div> <a href="/get-started" class="block mt-1 text-lg leading-tight font-semibold text-gray-900 hover:underline"> Finding customers for your new business </a> <p class="mt-2 text-gray-600"> Getting a new business off the ground is a lot of hard work. Here are five ideas you can use to find your first customers. </p> </div> </div>
注意:Tailwind 可以与任何语言(如 JSX)一起使用,通过在任何地方搜索类,而不仅仅是在 HTML 中。
动态类名
Tailwind 仅在您的代码中查找完整的类名。如果您使用字符串或部分构建类名。Tailwind 无法识别它们,也不会生成相应的 CSS。
创建类名时应遵循的措施。
- 始终使用完整的类名,而不是动态构建类名。
<div class="{{ error ? 'text-red-600' : 'text-green-600' }}"></div>
function Button({ color, children }) { const colorVariants = { blue: "bg-blue-600 hover:bg-blue-500", red: "bg-red-600 hover:bg-red-500", }; return <button className={`${colorVariants[color]} ...`}>{children}</button>; }
使用外部库
当使用第三方库并使用您自己的自定义 CSS 为其设置样式时,请尝试不要在不使用Tailwind 的 @layer功能的情况下编写这些样式。这将使 Tailwind 更容易扫描第三方库的源代码。
@tailwind base; @tailwind components; .select2-dropdown { @apply rounded-b-lg shadow-md; } .select2-search { @apply border border-gray-300 rounded; } .select2-results__group { @apply text-lg font-bold text-gray-900; } /* ... */ @tailwind utilities;
如果您在多个项目中使用 Tailwind 样式的组件,请确保已将 Tailwind 配置为扫描这些组件以查找类名。
module.exports = { content: [ './components/**/*.{html,js}', './pages/**/*.{html,js}', './node_modules/@my-company/tailwind-components/**/*.js', ], // ... }
如果您使用的是带有工作区的 monorepo,则可能需要使用require.resolve,以便 Tailwind 可以找到您的内容文件。
const path = require('path'); module.exports = { content: [ './components/**/*.{html,js}', './pages/**/*.{html,js}', path.join(path.dirname(require.resolve('@my-company/tailwind-components')), '**/*.js'), ], // ... }
使用相对路径
Tailwind 默认情况下使用当前目录作为路径。为了避免问题,将 'relative' 属性设置为 'true' 以将路径绑定到 'tailwind.config.js' 文件。
module.exports = { content: { relative: true, files: ["./pages/**/*.{html,js}", "./components/**/*.{html,js}"], }, // ... };
设置原始内容
要在 Tailwind 中扫描原始内容而不是文件的内容,请使用具有 "raw" 键的对象而不是文件路径。这允许您配置 Tailwind 以扫描自定义内容。
/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './pages/**/*.{html,js}', './components/**/*.{html,js}', { raw: '<div class="font-bold">', extension: 'html' }, ], // ... }
安全列表类
如果您希望 Tailwind 生成内容文件中不存在的某些类名,请使用 safelist 选项。
/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './pages/**/*.{html,js}', './components/**/*.{html,js}', ], safelist: [ 'bg-red-500', 'text-3xl', 'lg:text-4xl', ] // ... }
使用正则表达式
Tailwind 支持基于模式的安全列表,用于您需要安全列表许多类的情况。
/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './pages/**/*.{html,js}', './components/**/*.{html,js}', ], safelist: [ 'text-2xl', 'text-3xl', { pattern: /bg-(red|green|blue)-(100|200|300)/, }, ], // ... }
您可以强制 Tailwind 为某些类创建额外的样式,方法是将它们添加到 'variants' 选项中。
/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './pages/**/*.{html,js}', './components/**/*.{html,js}', ], safelist: [ 'text-2xl', 'text-3xl', { pattern: /bg-(red|green|blue)-(100|200|300)/, variants: ['lg', 'hover', 'focus', 'lg:hover'], }, ], // ... }
消除类
Tailwind 可能会创建不必要的类,例如即使不使用也会生成 'container' 类,如下所示。
<div class="text-lg leading-8 text-gray-600"> Every custom pool we design starts as a used shipping container, and is retrofitted with state of the art technology and finishes to turn it into a beautiful and functional way to entertain your guests all summer long. </div>
为了避免与现有 CSS 冲突,而无需为所有 Tailwind 类添加前缀,请使用blocklist选项忽略特定类。
/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './pages/**/*.{html,js}', './components/**/*.{html,js}', ], blocklist: [ 'container', 'collapse', ], // ... }
源文件转换
如果您编写的內容會轉換成 HTML(例如 Markdown),請先將內容轉換成 HTML,然后再提取类。使用 'content.transform' 转换文件并使用 'content.files' 指定源路径。
const remark = require('remark') module.exports = { content: { files: ['./src/**/*.{html,md}'], transform: { md: (content) => { return remark().process(content) } } }, // ... }
修改提取逻辑
使用 'extract' 自定义特定文件类型的类名检测。
注意:这是一个高级功能,您需要以不同的方式指定源路径。
/** @type {import('tailwindcss').Config} */ module.exports = { content: { files: ['./src/**/*.{html,wtf}'], extract: { wtf: (content) => { return content.match(/[^<>"'`\s]*/g) } } }, // ... }
常见问题排查
以下是配置 Tailwind CSS 内容时的一些常见问题,以及避免这些问题的措施。
- 缺少类:如果 Tailwind 没有生成类,请仔细检查您的内容配置和文件扩展名(例如,React 组件使用 'jsx' 而不是 'js'),以确保它匹配所有源文件。
module.exports = { content: [ './src/**/*.{html,js}', './src/**/*.{html,js,jsx}' ], // ... }
<!-- Incorrect --> <div class="text-{{ error ? 'red' : 'green' }}-600"></div> <!-- Correct --> <div class="{{ error ? 'text-red-600' : 'text-green-600' }}"></div>
content: [ './src/**/*.{html,js}', './src/pages/**/*.{html,js}', './src/components/**/*.{html,js}', './src/index.html', ],
// package.json { // ... "scripts": { "start": "concurrently \"npm run start:css\" \"react-scripts start\"", "start:css": "tailwindcss -o src/tailwind.css --watch", "build": "npm run build:css && react-scripts build", "build:css": "NODE_ENV=production tailwindcss -o src/tailwind.css -m", }, }