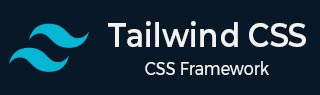
- Tailwind CSS 教程
- Tailwind CSS - 首页
- Tailwind CSS - 路线图
- Tailwind CSS - 简介
- Tailwind CSS - 安装
- Tailwind CSS - 编辑器设置
- Tailwind CSS - 核心概念
- Tailwind CSS - 实用优先基础
- Tailwind CSS - 悬停、焦点和其他状态
- Tailwind CSS - 响应式设计
- Tailwind CSS - 深色模式
- Tailwind CSS - 重用样式
- Tailwind CSS - 添加自定义样式
- Tailwind CSS - 函数和指令
- Tailwind CSS - 自定义
- Tailwind CSS - 配置
- Tailwind CSS - 内容配置
- Tailwind CSS - 主题配置
- Tailwind CSS - 自定义屏幕尺寸
- Tailwind CSS - 自定义颜色
- Tailwind CSS - 自定义间距
- Tailwind CSS - 插件
- Tailwind CSS - 预设
- Tailwind CSS - 基础样式
- Tailwind CSS - 预处理
- Tailwind CSS - 布局
- Tailwind CSS - 宽高比
- Tailwind CSS - 容器
- Tailwind CSS - 列
- Tailwind CSS - 断行后
- Tailwind CSS - 断行前
- Tailwind CSS - 断行内
- Tailwind CSS - 边框装饰断开
- Tailwind CSS - 盒子大小
- Tailwind CSS - 显示
- Tailwind CSS - 浮动
- Tailwind CSS - 清除浮动
- Tailwind CSS - 隔离
- Tailwind CSS - 对象适应
- Tailwind CSS - 对象位置
- Tailwind CSS - 溢出
- Tailwind CSS - 滚动行为
- Tailwind CSS - 位置
- Tailwind CSS - 上/右/下/左
- Tailwind CSS - 可见性
- Tailwind CSS - Z-index
- Tailwind CSS - Flexbox 和 Grid
- Tailwind CSS - Flex Basis
- Tailwind CSS - Flex Direction
- Tailwind CSS - Flex Wrap
- Tailwind CSS - Flex
- Tailwind CSS - Flex Grow
- Tailwind CSS - Flex Shrink
- Tailwind CSS - Order
- Tailwind CSS - Grid Template Columns
- Tailwind CSS - Grid Column Start / End
- Tailwind CSS - Grid Template Rows
- Tailwind CSS - Grid Row Start / End
- Tailwind CSS - Grid Auto Flow
- Tailwind CSS - Grid Auto Columns
- Tailwind CSS - Grid Auto Rows
- Tailwind CSS - Gap
- Tailwind CSS - Justify Content
- Tailwind CSS - Justify Items
- Tailwind CSS - Justify Self
- Tailwind CSS - Align Content
- Tailwind CSS - Align Items
- Tailwind CSS - Align Self
- Tailwind CSS - Place Content
- Tailwind CSS - Place Items
- Tailwind CSS - Place Self
- Tailwind CSS - 间距
- Tailwind CSS - 内边距
- Tailwind CSS - 外边距
- Tailwind CSS - 元素间距
- Tailwind CSS - 大小
- Tailwind CSS - 宽度
- Tailwind CSS - 最小宽度
- Tailwind CSS - 最大宽度
- Tailwind CSS - 高度
- Tailwind CSS - 最小高度
- Tailwind CSS - 最大高度
- Tailwind CSS - 尺寸
- Tailwind CSS - 排版
- Tailwind CSS - 字体系列
- Tailwind CSS - 字体大小
- Tailwind CSS - 字体平滑
- Tailwind CSS - 字体样式
- Tailwind CSS - 字体粗细
- Tailwind CSS - 数字字体变体
- Tailwind CSS - 字间距
- Tailwind CSS - 行数限制
- Tailwind CSS - 行高
- Tailwind CSS - 列表样式图像
- Tailwind CSS - 列表样式位置
- Tailwind CSS - 列表样式类型
- Tailwind CSS - 文本对齐
- Tailwind CSS - 文本颜色
- Tailwind CSS - 文本装饰
- Tailwind CSS - 文本装饰颜色
- Tailwind CSS - 文本装饰样式
- Tailwind CSS - 文本装饰粗细
- Tailwind CSS - 文本下划线偏移
- Tailwind CSS - 文本转换
- Tailwind CSS - 文本溢出
- Tailwind CSS - 文本换行
- Tailwind CSS - 文本缩进
- Tailwind CSS - 垂直对齐
- Tailwind CSS - 空格
- Tailwind CSS - 断字
- Tailwind CSS - 连字符
- Tailwind CSS - 内容
- Tailwind CSS - 背景
- Tailwind CSS - 背景附件
- Tailwind CSS - 背景裁剪
- Tailwind CSS - 背景颜色
- Tailwind CSS - 背景原点
- Tailwind CSS - 背景位置
- Tailwind CSS - 背景重复
- Tailwind CSS - 背景大小
- Tailwind CSS - 背景图片
- Tailwind CSS - 渐变颜色停止点
- Tailwind CSS - 边框
- Tailwind CSS - 边框半径
- Tailwind CSS - 边框宽度
- Tailwind CSS - 边框颜色
- Tailwind CSS - 边框样式
- Tailwind CSS - 分隔线宽度
- Tailwind CSS - 分隔线颜色
- Tailwind CSS - 分隔线样式
- Tailwind CSS - 轮廓宽度
- Tailwind CSS - 轮廓颜色
- Tailwind CSS - 轮廓样式
- Tailwind CSS - 轮廓偏移
- Tailwind CSS - 环宽度
- Tailwind CSS - 环颜色
- Tailwind CSS - 环偏移宽度
- Tailwind CSS - 环偏移颜色
- Tailwind CSS - 效果
- Tailwind CSS - 盒子阴影
- Tailwind CSS - 盒子阴影颜色
- Tailwind CSS - 不透明度
- Tailwind CSS - 混合模式
- Tailwind CSS - 背景混合模式
- Tailwind CSS - 滤镜
- Tailwind CSS - 模糊
- Tailwind CSS - 亮度
- Tailwind CSS - 对比度
- Tailwind CSS - 投影
- Tailwind CSS - 灰度
- Tailwind CSS - 色相旋转
- Tailwind CSS - 反转
- Tailwind CSS - 饱和度
- Tailwind CSS - 棕褐色
- Tailwind CSS - 背景模糊
- Tailwind CSS - 背景亮度
- Tailwind CSS - 背景对比度
- Tailwind CSS - 背景灰度
- Tailwind CSS - 背景色相旋转
- Tailwind CSS - 背景反转
- Tailwind CSS - 背景不透明度
- Tailwind CSS - 背景饱和度
- Tailwind CSS - 背景棕褐色
- Tailwind CSS - 表格
- Tailwind CSS - 边框塌陷
- Tailwind CSS - 边框间距
- Tailwind CSS - 表格布局
- Tailwind CSS - 标题位置
- Tailwind CSS - 过渡和动画
- Tailwind CSS - 过渡属性
- Tailwind CSS - 过渡时长
- Tailwind CSS - 过渡时序函数
- Tailwind CSS - 过渡延迟
- Tailwind CSS - 动画
- Tailwind CSS - 变换
- Tailwind CSS - 缩放
- Tailwind CSS - 旋转
- Tailwind CSS - 位移
- Tailwind CSS - 倾斜
- Tailwind CSS - 变换原点
- Tailwind CSS - 交互性
- Tailwind CSS - 强调色
- Tailwind CSS - 外观
- Tailwind CSS - 光标
- Tailwind CSS - 光标颜色
- Tailwind CSS - 指针事件
- Tailwind CSS - 调整大小
- Tailwind CSS - 滚动行为
- Tailwind CSS - 滚动边距
- Tailwind CSS - 滚动填充
- Tailwind CSS - 滚动捕捉对齐
- Tailwind CSS - 滚动捕捉停止
- Tailwind CSS - 滚动捕捉类型
- Tailwind CSS - 触摸操作
- Tailwind CSS - 用户选择
- Tailwind CSS - 将更改
- Tailwind CSS - SVG
- Tailwind CSS - 填充
- Tailwind CSS - 描边
- Tailwind CSS - 描边宽度
- Tailwind CSS - 可访问性
- Tailwind CSS - 屏幕阅读器
- Tailwind CSS - 强制颜色调整
- Tailwind CSS - 附加内容
- Tailwind CSS - 与预处理器一起使用
- Tailwind CSS - 生产环境优化
- Tailwind CSS - 参考
- Tailwind CSS - 核心概念
- Tailwind CSS - 自定义
- Tailwind CSS - 布局
- Tailwind CSS - Flexbox 和 Grid
- Tailwind CSS - 间距
- Tailwind CSS - 大小
- Tailwind CSS - 排版
- Tailwind CSS - 背景
- Tailwind CSS - 边框
- Tailwind CSS - 效果
- Tailwind CSS - 滤镜
- Tailwind CSS - 表格
- Tailwind CSS - 过渡和动画
- Tailwind CSS - 变换
- Tailwind CSS - 交互性
- Tailwind CSS - 资源
- Tailwind CSS - 讨论
- Tailwind CSS - 有用资源
Tailwind CSS - 主题配置
Tailwind CSS 主题配置指定项目的主题。
在 `tailwind.config.js` 中,“主题”部分允许您定义项目的调色板、类型比例、字体、断点、边框半径值等等。
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { screens: { sm: '480px', md: '768px', lg: '976px', xl: '1440px', }, colors: { 'blue': '#1fb6ff', 'purple': '#7e5bef', 'pink': '#ff49db', 'orange': '#ff7849', 'green': '#13ce66', 'yellow': '#ffc82c', 'gray-dark': '#273444', 'gray': '#8492a6', 'gray-light': '#d3dce6', }, fontFamily: { sans: ['Graphik', 'sans-serif'], serif: ['Merriweather', 'serif'], }, extend: { spacing: { '128': '32rem', '144': '36rem', }, borderRadius: { '4xl': '2rem', } } } }
主题结构
`tailwind.config.js` 的“主题”部分包含一系列键,例如 `screens`、`colors`、`spacing` 和 `corePlugins` 用于自定义。
屏幕尺寸
`screens` 键允许您设置项目中的响应式断点。
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { screens: { 'sm': '640px', 'md': '768px', 'lg': '1024px', 'xl': '1280px', '2xl': '1536px', } } }
颜色
`colors` 键允许您设置项目的全局调色板。
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { colors: { transparent: 'transparent', black: '#000', white: '#fff', gray: { 100: '#f7fafc', // ... 900: '#1a202c', }, // ... } } }
间距
`spacing` 键允许您设置项目的全局间距和大小比例。
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { spacing: { px: '1px', 0: '0', 0.5: '0.125rem', 1: '0.25rem', 1.5: '0.375rem', 2: '0.5rem', 2.5: '0.625rem', 3: '0.75rem', 3.5: '0.875rem', 4: '1rem', 5: '1.25rem', }, } }
核心插件
主题设置允许您为每个插件选择选项。例如,`borderRadius` 设置圆角样式。
module.exports = { theme: { borderRadius: { 'none': '0', 'sm': '.125rem', DEFAULT: '.25rem', 'lg': '.5rem', 'full': '9999px', }, } }
名称成为类名,值设置样式。这就是 Tailwind 对所有插件的工作方式。
.rounded-none { border-radius: 0 } .rounded-sm { border-radius: .125rem } .rounded { border-radius: .25rem } .rounded-lg { border-radius: .5rem } .rounded-full { border-radius: 9999px }
自定义默认主题
您的项目会自动使用默认主题设置。如果您想更改它们,您可以自定义主题以满足您的需求。
扩展默认主题
`theme.extend` 选项允许您向主题添加新值,同时保留默认值。这将结合新值和现有值,为您创建新的类。
例如,这里我们扩展 `fontFamily` 属性以添加 `font-display` 类,该类可以更改元素上使用的字体
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { extend: { fontFamily: { display: 'Oswald, ui-serif', // Adds a new `font-display` class } } } }
添加到您的主题后,您可以像使用任何其他字体系列实用程序一样使用它
<h1 class="font-display"> This uses the Oswald font </h1>
覆盖默认主题
您可以通过直接在 `tailwind.config.js` 中的主题部分添加覆盖来更改默认主题选项。
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { // Replaces all of the default `opacity` values opacity: { '0': '0', '20': '0.2', '40': '0.4', '60': '0.6', '80': '0.8', '100': '1', } } }
引用其他值
使用闭包来引用其他主题值。它为您提供了一个 `theme()` 函数,可以使用点表示法访问其他值。
例如,您可以通过在您的 `backgroundSize` 配置中引用 `theme('spacing')` 来为 `spacing` 比例中的每个值生成 `background-size` 实用程序。
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { spacing: { // ... }, backgroundSize: ({ theme }) => ({ auto: 'auto', cover: 'cover', contain: 'contain', ...theme('spacing') }) } }
**注意:**对顶级主题键使用函数。
/** @type {import('tailwindcss').Config} */ module.exports = { theme: { fill: ({ theme }) => ({ gray: theme('colors.gray') }) } }
引用默认主题
要使用默认主题中的值,请从 `tailwindcss/defaultTheme` 导入它。
const defaultTheme = require('tailwindcss/defaultTheme') module.exports = { theme: { extend: { fontFamily: { sans: [ 'Lato', ...defaultTheme.fontFamily.sans, ] } } } }
禁用整个核心插件
要禁用核心插件,请在 `corePlugins` 中将其设置为 `false`,而不是在主题配置中设置为空对象。
/** Don't Do */ /** @type {import('tailwindcss').Config} */ module.exports = { theme: { opacity: {}, } } /** Always Do */ /** @type {import('tailwindcss').Config} */ module.exports = { corePlugins: { opacity: false, } }
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
配置参考
大多数主题对象键与 Tailwind 的核心插件匹配,除了 `screens`、`colors` 和 `spacing`。一些插件没有主题键,因为它们只接受固定值。您还可以使用 `theme.extend` 键扩展默认主题。
所有这些键也可以在 `theme.extend` 键下使用,以启用扩展默认主题。
类名 | CSS 属性 |
---|---|
accentColor | `accent-color` 属性的值 |
animation | `animation` 属性的值 |
aria | `aria` 属性的值 |
aspectRatio | `aspect-ratio` 属性的值 |
backdropBlur | `backdropBlur` 插件的值 |
backdropBrightness | `backdropBrightness` 插件的值 |
backdropContrast | 背景对比度插件的值 |
backdropGrayscale | backdropGrayscale 插件的值 |
backdropHueRotate | backdropHueRotate 插件的值 |
backdropInvert | backdropInvert 插件的值 |
backdropOpacity | backdropOpacity 插件的值 |
backdropSaturate | backdropSaturate 插件的值 |
backdropSepia | backdropSepia 插件的值 |
backgroundColor | background-color 属性的值 |
backgroundImage | background-image 属性的值 |
backgroundOpacity | background-opacity 属性的值 |
backgroundPosition | background-position 属性的值 |
backgroundSize | background-size 属性的值 |
blur | blur 插件的值 |
borderColor | border-color 属性的值 |
borderOpacity | borderOpacity 插件的值 |
borderRadius | border-radius 属性的值 |
borderSpacing | border-spacing 属性的值 |
borderWidth | borderWidth 插件的值 |
boxShadow | box-shadow 属性的值 |
boxShadowColor | boxShadowColor 插件的值 |
brightness | brightness 插件的值 |
caretColor | caret-color 属性的值 |
颜色 | 您项目的调色板 |
列 | columns 属性的值 |
容器 | 容器插件的配置 |
内容 | content 属性的值 |
对比度 | contrast 插件的值 |
光标 | cursor 属性的值 |
分隔线颜色 | divideColor 插件的值 |
分隔线透明度 | divideOpacity 插件的值 |
分隔线宽度 | divideWidth 插件的值 |
投影 | dropShadow 插件的值 |
填充 | fill 插件的值 |
弹性盒 | flex 属性的值 |
flexBasis | flex-basis 属性的值 |
flexGrow | flex-grow 属性的值 |
flexShrink | flex-shrink 属性的值 |
fontFamily | font-family 属性的值 |
fontSize | font-size 属性的值 |
fontWeight | font-weight 属性的值 |
间隙 | gap 属性的值 |
渐变颜色停止点 | gradientColorStops 插件的值 |
渐变颜色停止点位置 | gradient-color-stop-positions 属性的值 |
灰度 | grayscale 插件的值 |
gridAutoColumns | grid-auto-columns 属性的值 |
gridAutoRows | grid-auto-rows 属性的值 |
gridColumn | grid-column 属性的值 |
gridColumnEnd | grid-column-end 属性的值 |
gridColumnStart | grid-column-start 属性的值 |
gridRow | grid-row 属性的值 |
gridRowEnd | grid-row-end 属性的值 |
gridRowStart | grid-row-start 属性的值 |
gridTemplateColumns | grid-template-columns 属性的值 |
gridTemplateRows | grid-template-rows 属性的值 |
高度 | height 属性的值 |
色相旋转 | hueRotate 插件的值 |
内边距 | top、right、bottom 和 left 属性的值 |
反转 | invert 插件的值 |
关键帧 | 动画插件中使用的关键帧值 |
字母间距 | letter-spacing 属性的值 |
行高 | line-height 属性的值 |
列表样式类型 | list-style-type 属性的值 |
列表样式图片 | list-style-image 属性的值 |
外边距 | margin 属性的值 |
行限制 | line-clamp 属性的值 |
最大高度 | max-height 属性的值 |
最大宽度 | max-width 属性的值 |
最小高度 | min-height 属性的值 |
最小宽度 | min-width 属性的值 |
对象位置 | object-position 属性的值 |
透明度 | opacity 属性的值 |
顺序 | order 属性的值 |
轮廓颜色 | outline-color 属性的值 |
轮廓偏移 | outline-offset 属性的值 |
轮廓宽度 | outline-width 属性的值 |
内填充 | padding 属性的值 |
占位符颜色 | placeholderColor 插件的值 |
占位符透明度 | placeholderOpacity 插件的值 |
环颜色 | ringColor 插件的值 |
环偏移颜色 | ringOffsetColor 插件的值 |
环偏移宽度 | ringOffsetWidth 插件的值 |
环透明度 | ringOpacity 插件的值 |
环宽度 | ringWidth 插件的值 |
旋转 | rotate 插件的值 |
饱和度 | saturate 插件的值 |
缩放 | scale 插件的值 |
屏幕 | 您项目的响应式断点 |
滚动边距 | scroll-margin 属性的值 |
滚动填充 | scroll-padding 属性的值 |
棕褐色 | sepia 插件的值 |
倾斜 | skew 插件的值 |
间距 | space 插件的值 |
间距 | 您项目的间距比例 |
描边 | stroke 属性的值 |
描边宽度 | stroke-width 属性的值 |
支持 | supports 属性的值 |
数据 | data 属性的值 |
文本颜色 | color 属性的值 |
文本装饰颜色 | text-decoration-color 属性的值 |
文本装饰粗细 | text-decoration-thickness 属性的值 |
文本缩进 | text-indent 属性的值 |
文本透明度 | textOpacity 插件的值 |
文本下划线偏移 | text-underline-offset 属性的值 |
变换原点 | transform-origin 属性的值 |
过渡延迟 | transition-delay 属性的值 |
过渡持续时间 | transition-duration 属性的值 |
过渡属性 | transition-property 属性的值 |
过渡时序函数 | transition-timing-function 属性的值 |
平移 | translate 插件的值 |
尺寸 | size 属性的值 |
宽度 | width 属性的值 |
将要更改 | will-change 属性的值 |
Z 轴索引 | z-index 属性的值 |