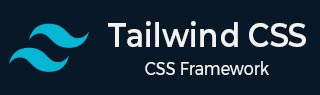
- Tailwind CSS 教程
- Tailwind CSS - 首页
- Tailwind CSS - 路线图
- Tailwind CSS - 简介
- Tailwind CSS - 安装
- Tailwind CSS - 编辑器设置
- Tailwind CSS - 核心概念
- Tailwind CSS - 实用优先基础
- Tailwind CSS - 悬停、焦点和其他状态
- Tailwind CSS - 响应式设计
- Tailwind CSS - 深色模式
- Tailwind CSS - 重用样式
- Tailwind CSS - 添加自定义样式
- Tailwind CSS - 函数与指令
- Tailwind CSS - 自定义
- Tailwind CSS - 配置
- Tailwind CSS - 内容配置
- Tailwind CSS - 主题配置
- Tailwind CSS - 自定义屏幕尺寸
- Tailwind CSS - 自定义颜色
- Tailwind CSS - 自定义间距
- Tailwind CSS - 插件
- Tailwind CSS - 预设
- Tailwind CSS - 基础样式
- Tailwind CSS - 预处理
- Tailwind CSS - 布局
- Tailwind CSS - 宽高比
- Tailwind CSS - 容器
- Tailwind CSS - 列
- Tailwind CSS - 分栏后
- Tailwind CSS - 分栏前
- Tailwind CSS - 分栏内
- Tailwind CSS - 边框装饰断开
- Tailwind CSS - 盒子尺寸
- Tailwind CSS - 显示
- Tailwind CSS - 浮动
- Tailwind CSS - 清除浮动
- Tailwind CSS - 隔离
- Tailwind CSS - 对象适应
- Tailwind CSS - 对象位置
- Tailwind CSS - 溢出
- Tailwind CSS - 滚动溢出行为
- Tailwind CSS - 定位
- Tailwind CSS - 上/右/下/左
- Tailwind CSS - 可见性
- Tailwind CSS - Z轴索引
- Tailwind CSS - Flexbox 和 Grid
- Tailwind CSS - Flex 基准
- Tailwind CSS - Flex 方向
- Tailwind CSS - Flex 换行
- Tailwind CSS - Flex
- Tailwind CSS - Flex 伸长
- Tailwind CSS - Flex 缩短
- Tailwind CSS - 顺序
- Tailwind CSS - 网格模板列
- Tailwind CSS - 网格列开始/结束
- Tailwind CSS - 网格模板行
- Tailwind CSS - 网格行开始/结束
- Tailwind CSS - 网格自动填充
- Tailwind CSS - 网格自动列
- Tailwind CSS - 网格自动行
- Tailwind CSS - 间隙
- Tailwind CSS - 内容对齐
- Tailwind CSS - 项目对齐
- Tailwind CSS - 自身对齐
- Tailwind CSS - 内容排列
- Tailwind CSS - 项目排列
- Tailwind CSS - 自身排列
- Tailwind CSS - 内容放置
- Tailwind CSS - 项目放置
- Tailwind CSS - 自身放置
- Tailwind CSS - 间距
- Tailwind CSS - 内边距
- Tailwind CSS - 外边距
- Tailwind CSS - 元素间距
- Tailwind CSS - 尺寸
- Tailwind CSS - 宽度
- Tailwind CSS - 最小宽度
- Tailwind CSS - 最大宽度
- Tailwind CSS - 高度
- Tailwind CSS - 最小高度
- Tailwind CSS - 最大高度
- Tailwind CSS - 尺寸
- Tailwind CSS - 排版
- Tailwind CSS - 字体系列
- Tailwind CSS - 字体大小
- Tailwind CSS - 字体平滑
- Tailwind CSS - 字体样式
- Tailwind CSS - 字体粗细
- Tailwind CSS - 字体变体数字
- Tailwind CSS - 字间距
- Tailwind CSS - 行数限制
- Tailwind CSS - 行高
- Tailwind CSS - 列表样式图片
- Tailwind CSS - 列表样式位置
- Tailwind CSS - 列表样式类型
- Tailwind CSS - 文本对齐
- Tailwind CSS - 文本颜色
- Tailwind CSS - 文本装饰
- Tailwind CSS - 文本装饰颜色
- Tailwind CSS - 文本装饰样式
- Tailwind CSS - 文本装饰粗细
- Tailwind CSS - 文本下划线偏移
- Tailwind CSS - 文本转换
- Tailwind CSS - 文本溢出
- Tailwind CSS - 文本换行
- Tailwind CSS - 文本缩进
- Tailwind CSS - 垂直对齐
- Tailwind CSS - 空格
- Tailwind CSS - 断词
- Tailwind CSS - 连字符
- Tailwind CSS - 内容
- Tailwind CSS - 背景
- Tailwind CSS - 背景附件
- Tailwind CSS - 背景裁剪
- Tailwind CSS - 背景颜色
- Tailwind CSS - 背景原点
- Tailwind CSS - 背景位置
- Tailwind CSS - 背景重复
- Tailwind CSS - 背景尺寸
- Tailwind CSS - 背景图片
- Tailwind CSS - 渐变颜色停止
- Tailwind CSS - 边框
- Tailwind CSS - 圆角
- Tailwind CSS - 边框宽度
- Tailwind CSS - 边框颜色
- Tailwind CSS - 边框样式
- Tailwind CSS - 分隔线宽度
- Tailwind CSS - 分隔线颜色
- Tailwind CSS - 分隔线样式
- Tailwind CSS - 轮廓宽度
- Tailwind CSS - 轮廓颜色
- Tailwind CSS - 轮廓样式
- Tailwind CSS - 轮廓偏移
- Tailwind CSS - 环宽度
- Tailwind CSS - 环颜色
- Tailwind CSS - 环偏移宽度
- Tailwind CSS - 环偏移颜色
- Tailwind CSS - 视觉效果
- Tailwind CSS - 盒子阴影
- Tailwind CSS - 盒子阴影颜色
- Tailwind CSS - 不透明度
- Tailwind CSS - 混合模式
- Tailwind CSS - 背景混合模式
- Tailwind CSS - 滤镜
- Tailwind CSS - 模糊
- Tailwind CSS - 亮度
- Tailwind CSS - 对比度
- Tailwind CSS - 投影
- Tailwind CSS - 灰度
- Tailwind CSS - 色相旋转
- Tailwind CSS - 反转
- Tailwind CSS - 饱和度
- Tailwind CSS - 棕褐色
- Tailwind CSS - 背景模糊
- Tailwind CSS - 背景亮度
- Tailwind CSS - 背景对比度
- Tailwind CSS - 背景灰度
- Tailwind CSS - 背景色相旋转
- Tailwind CSS - 背景反转
- Tailwind CSS - 背景不透明度
- Tailwind CSS - 背景饱和度
- Tailwind CSS - 背景棕褐色
- Tailwind CSS - 表格
- Tailwind CSS - 边框折叠
- Tailwind CSS - 边框间距
- Tailwind CSS - 表格布局
- Tailwind CSS - 标题位置
- Tailwind CSS - 过渡与动画
- Tailwind CSS - 过渡属性
- Tailwind CSS - 过渡时长
- Tailwind CSS - 过渡时间函数
- Tailwind CSS - 过渡延迟
- Tailwind CSS - 动画
- Tailwind CSS - 变换
- Tailwind CSS - 缩放
- Tailwind CSS - 旋转
- Tailwind CSS - 位移
- Tailwind CSS - 倾斜
- Tailwind CSS - 变换原点
- Tailwind CSS - 交互性
- Tailwind CSS - 重音颜色
- Tailwind CSS - 外观
- Tailwind CSS - 光标
- Tailwind CSS - 插入符号颜色
- Tailwind CSS - 指针事件
- Tailwind CSS - 调整大小
- Tailwind CSS - 滚动行为
- Tailwind CSS - 滚动边距
- Tailwind CSS - 滚动填充
- Tailwind CSS - 滚动捕捉对齐
- Tailwind CSS - 滚动捕捉停止
- Tailwind CSS - 滚动捕捉类型
- Tailwind CSS - 触摸操作
- Tailwind CSS - 用户选择
- Tailwind CSS - 将要更改
- Tailwind CSS - SVG
- Tailwind CSS - 填充
- Tailwind CSS - 描边
- Tailwind CSS - 描边宽度
- Tailwind CSS - 可访问性
- Tailwind CSS - 屏幕阅读器
- Tailwind CSS - 强制颜色调整
- Tailwind CSS - 附加
- Tailwind CSS - 与预处理器一起使用
- Tailwind CSS - 生产优化
- Tailwind CSS - 参考
- Tailwind CSS - 核心概念
- Tailwind CSS - 自定义
- Tailwind CSS - 布局
- Tailwind CSS - Flexbox 和 Grid
- Tailwind CSS - 间距
- Tailwind CSS - 尺寸
- Tailwind CSS - 排版
- Tailwind CSS - 背景
- Tailwind CSS - 边框
- Tailwind CSS - 视觉效果
- Tailwind CSS - 滤镜
- Tailwind CSS - 表格
- Tailwind CSS - 过渡与动画
- Tailwind CSS - 变换
- Tailwind CSS - 交互性
- Tailwind CSS - 资源
- Tailwind CSS - 讨论
- Tailwind CSS - 有用资源
Tailwind CSS - 函数与指令
指令在 Tailwind CSS 中是用于在 CSS 中应用或自定义样式的命令。
指令是您在 CSS 中使用的Tailwind CSS 命令,用于访问特殊功能并控制 Tailwind CSS 应用的样式。
@tailwind
@tailwind 指令允许您将 Tailwind 的基础样式、组件、实用程序和变体直接包含到您的 CSS 中。
示例
/** * Inserts Tailwind's foundational styles and any additional base styles from plugins. */ @tailwind base; /** * Includes Tailwind's component styles and any extra component styles from plugins. */ @tailwind components; /** * Adds Tailwind's utility classes and any additional utility classes from plugins. */ @tailwind utilities; /** * Controls the placement of variant styles (like hover, focus, and responsive) in your CSS. * If not specified, these variants are added at the end of your stylesheet by default. */ @tailwind variants;
@layer
@layer 指令允许您指定自定义样式在 Tailwind 中属于哪个类别(基础、组件或实用程序)。
示例
/** * Import Tailwind's base styles, component styles, and utility classes into your CSS. */ @tailwind base; @tailwind components; @tailwind utilities; /** * Define custom base styles for HTML elements using the base layer. */ @layer base { h1 { @apply font-bold text-3xl; /* Applies a bold font and large size to h1 elements */ } h2 { @apply font-semibold text-2xl; /* Applies a semi-bold font and medium size to h2 elements */ } } /** * Create custom component styles using the components layer. */ @layer components { .card { @apply bg-gray-100 border border-gray-300 p-4 rounded-lg shadow-md; /* Styles for a card component */ } } /** * Add custom utility classes using the utilities layer. */ @layer utilities { .no-opacity { opacity: 1; /* Sets the element's opacity to fully opaque */ } .blurred { filter: blur(5px); /* Applies a blur effect to elements */ } }
Tailwind 会自动在@layer 指令中组织 CSS 以匹配@tailwind规则的顺序,因此您无需担心顺序以避免特异性问题。
这些层中的自定义 CSS 仅在 HTML 中使用时才会包含在最终构建中,就像默认的 Tailwind 类一样。
此外,使用@layer 允许您将修饰符(如悬停、焦点)和响应式断点(如md: 和lg:)应用到您的自定义样式。
@apply
@apply 允许您将现有的实用程序类直接包含到您的自定义 CSS 中。
如果您希望在编写自己的 CSS 时使用 Tailwind 的样式,这将非常有用,从而更容易自定义或覆盖来自其他来源的样式。
示例
.custom-card { @apply rounded-lg shadow-lg; } .custom-input { @apply border border-gray-400 rounded-md; } .custom-header { @apply text-xl font-semibold text-gray-800; }
当您使用@apply 时,默认情况下会删除任何!important声明以防止与其他 CSS 规则冲突。以下是它的工作原理。
示例
/* Define a class with !important */ .text-red { color: red !important; } /* Apply the .text-red class to another class */ .alert { @apply text-red; } /* The .text-red class retains the !important declaration */ .text-red { color: red !important; } /* The .alert class does not include !important */ .alert { color: red; }
如果您想使用@apply包含来自现有类的样式并确保它们是!important,则需要在自定义 CSS 中每个属性的末尾显式添加!important。
/* The .card class without !important */ .card { padding: 1rem; background-color: #edf2f7; border: 1px solid #cbd5e0; border-radius: .375rem; } /* The .card-important class with !important */ .card-important { padding: 1rem !important; background-color: #edf2f7 !important; border: 1px solid #cbd5e0 !important; border-radius: .375rem !important; }
要使用@apply应用!important,请在每个属性中添加!important。Sass/SCSS,请使用这种方法包含!important
/* Apply !important using Sass variable */ .card-important { @apply p-4 bg-gray-200 border border-gray-400 rounded; @apply #{$important}; /* Applies !important */ }
在每个组件的 CSS 中使用 @apply
在Vue 和Svelte 等框架中,您可以将样式直接包含在每个组件文件内的<style>块中。
尝试在Vue 或Svelte 等框架中的<style>块内使用来自全局 CSS 的自定义类会导致错误,因为它找不到该类。
示例 :main.css
@tailwind base; @tailwind components; @tailwind utilities; @layer components { .button { background-color: theme('colors.blue.500'); border-radius: theme('borderRadius.md'); padding: theme('spacing.4'); color: theme('colors.white'); } }
示例 :Card.svelte
<button> <slot></slot> </button> <style> button { /* This won't work because Button.svelte and main.css are processed separately */ @apply button; } </style>
在此示例中,.button 在 main.css 中定义,但Button.svelte 中的@apply不起作用,因为每个文件都是单独处理的。Vue 和Svelte独立处理它们的<style>块,因此它们无法直接使用来自全局 CSS 的样式。
当你有多个组件,每个组件都有自己的<style>块时,Tailwind 会分别处理每个文件。例如,如果你在main.css中定义了一个.button类,但尝试在Button.svelte中使用@apply button,它将无法工作。这是因为 Tailwind 会独立处理Button.svelte和main.css,所以在Button.svelte中使用.button类时,它无法识别该类。
不要尝试跨文件使用@apply,而是在Tailwind 的配置中直接定义你的样式。以下是方法。
示例
const plugin = require('tailwindcss/plugin') module.exports = { // ... plugins: [ plugin(function ({ addComponents, theme }) { addComponents({ '.button': { backgroundColor: theme('colors.blue.500'), borderRadius: theme('borderRadius.md'), padding: theme('spacing.4'), boxShadow: theme('boxShadow.md'), color: theme('colors.white'), } }) }) ] }
通过这样做,任何 Tailwind CSS 文件都可以访问.button类。
为了获得更流畅的体验,最好直接在 HTML 中使用Tailwind 的实用程序类,而不是依赖于跨文件的@apply。这种方法简化了你的设置并避免了复杂情况。
@config
@config 指令告诉 Tailwind CSS 在处理特定 CSS 文件时使用哪个配置文件。如果你项目的不同部分使用了不同的配置文件,这将非常有用。
在site.css中,你可能会使用默认配置。
示例
@config "./tailwind.site.config.js"; @tailwind base; @tailwind components; @tailwind utilities;
在admin.css中,你可以使用以下方式指定不同的配置文件:
示例
@config "./tailwind.admin.config.js"; @tailwind base; @tailwind components; @tailwind utilities;
你在@config指令中指定的路径相对于 CSS 文件本身,并将覆盖你在 PostCSS 设置或 Tailwind CLI 中设置的任何配置。
当使用 Tailwind CSS 与postcss-import时,请确保所有@import语句都位于 CSS 文件中@config指令之前。
为什么?postcss-import需要先处理@import语句,遵循 CSS 规则,这些规则要求导入出现在任何其他规则之前。如果你将@config放在@import语句之前,会导致问题,你的样式可能无法正确处理。
示例:错误顺序
/* Incorrect order - will cause problems */ @config "./tailwind.admin.config.js"; @import "tailwindcss/base"; @import "./custom-base.css"; @import "tailwindcss/components"; @import "./custom-components.css"; @import "tailwindcss/utilities";
示例:正确顺序
/* Always put @import first */ @import "tailwindcss/base"; @import "./custom-base.css"; @import "tailwindcss/components"; @import "./custom-components.css"; @import "tailwindcss/utilities"; @config "./tailwind.admin.config.js";
函数
使用 Tailwind,你可以在 CSS 中使用自定义函数来访问Tailwind 特定的值。这些函数在构建时计算,并在最终 CSS 中转换为静态值。
@theme
要访问Tailwind 配置中的值,只需使用带点表示法的theme()函数即可。
示例
.button { border-width: theme(borderWidth.md); }
如果需要使用带点的值(如边框宽度比例中的 3.5),请使用方括号
示例
.button { border-width: theme(borderWidth[3.5]); }
要访问Tailwind 默认调色板中的嵌套颜色,请使用点表示法以确保正确引用颜色值。
避免对嵌套颜色值使用连字符。
示例
/* Incorrect */ .button { background-color: theme(colors.green-500); }
使用点来访问嵌套颜色值。
示例
/* Correct */ .button { background-color: theme(colors.green.500); }
要更改主题颜色的不透明度,请添加一个斜杠,后跟不透明度百分比
示例
.card-bg { background-color: theme(colors.green.300 / 50%); }
@screens
Tailwind CSS 中的screen()函数允许你使用命名断点创建媒体查询,避免在 CSS 中重复值。
示例
@media screen(md) { /* Styles for medium screens and up */ }
在构建 CSS 时,这将转换为指定断点的标准媒体查询。
示例
@media (min-width: 768px) { /* Styles for screens that are at least 768px wide */ }
@screens
Tailwind CSS 中的screen()函数可帮助你使用命名断点创建媒体查询,而不是在 CSS 中重复其值。
示例
@media screen(md) { /* Styles for medium screens and up */ }
在构建 CSS 时,这将转换为指定断点的标准媒体查询。
示例
@media (min-width: 768px) { /* Styles for screens that are at least 768px wide */ }