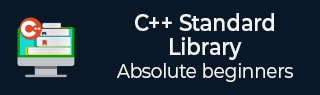
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ forward_list::before_begin() 函数
C++ 的 std::forward_list::before_begin() 函数用于获取指向 forward_list 容器第一个元素之前元素的迭代器。
此 forward_list 的元素充当占位符。此函数返回的迭代器可与 emplace_after()、erase_after()、insert_after() 和 splice_after() 函数一起使用,以在返回的迭代器位置插入、删除和替换元素。before_begin() 函数类似于 C++ std::forward_list 中的 cbefore_begin() 函数。
语法
以下是 C++ std::forward_list::before_begin() 函数的语法:
iterator before_begin();
参数
- 它不接受任何参数。
返回值
此函数返回指向第一个元素之前元素的迭代器。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 1
如果 forward_list 为 int 类型,则 before_begin() 函数返回指向第一个元素之前元素的迭代器。
在下面的程序中,我们使用 C++ std::forward_list::before_begin() 函数来检索指向此 forward_list {10, 20, 30, 40, 50} 的第一个元素之前元素的迭代器。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<int> num_list = {10, 20, 30, 40, 50}; cout<<"The num_list contents are: "<<endl; for(int n : num_list){ cout<<n<<endl; } //using the before_begin() function auto it = num_list.before_begin(); cout<<"An iterator which points to the position before the first element is: "<<*it; }
输出
让我们编译并运行上述程序,这将产生以下结果:
The num_list contents are: 10 20 30 40 50 An iterator which points to the position before the first element is: 0
示例 2
如果 forward_list 为 char 类型,则此函数返回指向第一个元素之前元素的迭代器。
以下是 C++ std::forward_list::before_begin() 函数的另一个示例。在这里,我们创建一个名为 char_list 的 forward_list(char 类型),其内容为 {'b', 'c', 'd', 'e'}。然后,我们使用 before_begin() 函数来检索指向此 forward_list 的第一个元素之前元素的迭代器,并使用 emplace_after() 函数在返回的迭代器位置插入一个新元素 'a'。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<char> char_list = {'b', 'c', 'd', 'e'}; cout<<"The char_list contents are: "<<endl; for(char c : char_list){ cout<<c<<endl; } //using the before_begin() function auto it = char_list.before_begin(); cout<<"An iterator is: "<<*it<<endl; //using emplace_after() function char_list.emplace_after(it, 'a'); cout<<"The char_list contents after inserting new element: "<<endl; for(char c: char_list){ cout<<c<<endl; } }
输出
这将生成以下输出:
The char_list contents are: b c d e An iterator is: 0 The char_list contents after inserting new element: a b c d e
示例 3
除了 int 类型和 char 类型的 forward_list 之外,我们还可以检索 string 类型 forward_list 元素的迭代器。
在这个例子中,我们创建了一个名为 names 的 forward_list(string 类型),其内容为 {"Raju", "Aman", "Rahul", "Ganesh"}。然后,使用 before_begin() 函数,我们尝试检索指向第一个元素之前元素的迭代器,并使用 insert_after() 函数在返回的迭代器位置插入一个新元素 "Ramesh"。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<string> names = {"Raju", "Aman", "Rahul", "Ganesh"}; cout<<"The names forward_list contents are: "<<endl; for(string n : names){ cout<<n<<endl; } //using the before_begin() function auto it = names.before_begin(); cout<<"An iterator is: "<<*it<<endl; //using the insert_after()function names.insert_after(it, "Ramesh"); cout<<"The names forward_list contents after inserting new element: "<<endl; for(string s : names){ cout<<s<<endl; } }
输出
以下是上述程序的输出:
The names forward_list contents are: Raju Aman Rahul Ganesh An iterator is: The names forward_list contents after inserting new element: Ramesh Raju Aman Rahul Ganesh