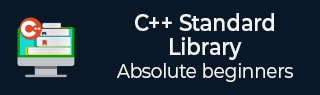
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ forward_list::begin() 函数
C++ 的std::forward_list::begin()函数用于检索指向forward_list容器第一个元素的迭代器。
如果当前forward_list为空,则begin()函数返回的迭代器等于end()函数的结果。在C++中,迭代器是一个可以迭代STL(标准模板库)中元素并提供对单个元素访问的对象。
语法
以下是C++ std::forward_list::begin()函数的语法:
iterator begin();
参数
- 它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回指向第一个元素的迭代器。
示例 1
如果forward_list非空且为int类型,则begin()函数返回指向第一个元素的迭代器。
在以下示例中,我们使用C++ std::forward_list::begin()函数来检索当前forward_list {1, 2, 3, 4, 5}的第一个元素的迭代器。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<int> num_list = {1, 2, 3, 4, 5}; cout<<"The num_list contents are: "<<endl; for(int n : num_list) { cout<<n<<endl; } //using the begin() function auto it = num_list.begin(); cout<<"The iterator to the first element: "<<*it; }
输出
以下是上述程序的输出:
The num_list contents are: 1 2 3 4 5 The iterator to the first element: 1
示例 2
如果forward_list为char类型,则此函数返回指向此forward_list的第一个char元素的迭代器。
以下是C++ d::forward_list::begin()函数的另一个示例。在这里,我们创建了一个名为char_list的forward_list(char类型),其内容为{'a', 'b', 'c', 'd', 'e'}。然后,使用begin()函数,我们尝试检索此forward_list第一个元素的迭代器。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<char> char_list = {'a', 'b', 'c', 'd', 'e'}; cout<<"The char_list contents are: "<<endl; for(char c : char_list) { cout<<c<<endl; } //using the begin() function auto it = char_list.begin(); cout<<"The iterator to the first element: "<<*it; }
输出
这将生成以下输出:
The char_list contents are: a b c d e The iterator to the first element: a
示例 3
使用for循环以及begin()函数遍历容器并检索从第一个元素开始的所有元素的迭代器。
在以下程序中,我们创建了一个名为fruits的forward_list(string类型),其内容为{"Apple", "Banana", "Orange", "Grapes"}。然后,使用for循环以及begin()函数遍历此forward_list并检索从第一个元素开始的所有元素的迭代器。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<string> fruits = {"Apple", "Banana", "Orange", "Grapes"}; cout<<"The iterator of all the elements are: "<<endl; for(auto it = fruits.begin(); it != fruits.end(); it++){ cout<<*it<<endl; } }
输出
上述程序产生以下输出:
The iterator of all the elements are: Apple Banana Orange Grapes
示例 4
如果forward_list为空,则返回的迭代器等于end()函数的结果。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<string> fruits = {}; cout<<"The size of this forward_list is: "<<endl; cout<<distance(fruits.begin(), fruits.end())<<endl; cout<<"The iterator of all the elements are: "<<endl; //using the begin() function auto it = fruits.begin(); cout<<*it<<endl; }
输出
执行上述程序后,它将产生以下输出:
The size of this forward_list is: 0 The iterator of all the elements are: Segmentation fault (core dumped)