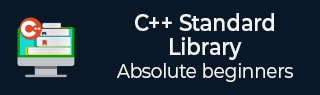
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ forward_list::cbegin() 函数
C++ 的 std::forward_list::cbegin() 函数用于获取指向 forward_list 容器第一个元素的常量迭代器。
如果当前 forward_list 为空,则 cbegin() 函数返回的常量迭代器等于 end() 函数的结果。在 C++ 中,迭代器是一个可以遍历 STL(标准模板库)中元素并访问单个元素的对象。
语法
以下是 C++ std::forward_list::cbegin() 函数的语法:
const_iterator cbegin() const;
参数
- 它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回指向第一个元素的常量迭代器。
示例 1
如果 forward_list 非空且为 int 类型,则 cbegin() 函数返回指向第一个元素的常量迭代器。
在下面的示例中,我们使用 C++ std::forward_list::cbegin() 函数来获取当前 forward_list {10, 20, 30, 40, 50} 的第一个元素的常量迭代器。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<int> num_list = {10, 20, 30, 40, 50}; cout<<"The num_list contents are: "<<endl; for(int n : num_list) { cout<<n<<endl; } //using the cbegin() function auto it = num_list.cbegin(); cout<<"The constant iterator to the first element: "<<*it; }
输出
以下是上述程序的输出:
The num_list contents are: 10 20 30 40 50 The constant iterator to the first element: 10
示例 2
如果 forward_list 为 char 类型,则此函数返回指向此 forward_list 的第一个 char 元素的常量迭代器。
以下是 C++ d::forward_list::cbegin() 函数的另一个示例。在这里,我们创建一个名为 char_list 的 forward_list(类型为 char),其内容为 {'A', 'B', 'C', 'D', 'E'}。然后,使用 cbegin() 函数,我们尝试获取此 forward_list 的第一个元素的常量迭代器。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<char> char_list = {'A', 'B', 'C', 'D', 'E'}; cout<<"The char_list contents are: "<<endl; for(char c : char_list) { cout<<c<<endl; } //using the cbegin() function auto it = char_list.cbegin(); cout<<"The constant iterator to the first element: "<<*it; }
输出
这将生成以下输出:
The char_list contents are: A B C D E The constant iterator to the first element: A
示例 3
使用 for 循环以及 cbegin() 函数遍历容器并获取从第一个元素开始的所有元素的常量迭代器。
在下面的程序中,我们创建一个名为 colors 的 forward_list(类型为 string),其内容为 {"Red", "Green", "Blue", "Black"}。然后,使用 for 循环以及 cbegin() 函数遍历此 forward_list 并获取从第一个元素开始的所有元素的常量迭代器。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<string> colors = {"Red", "Green", "Blue", "Black"}; cout<<"The iterator of all the elements are: "<<endl; for(auto it = colors.cbegin(); it != colors.end(); it++){ cout<<*it<<endl; } }
输出
上述程序产生以下输出:
The iterator of all the elements are: Red Green Blue Black
示例 4
如果 forward_list 为空,则返回的常量迭代器等于 end() 函数的结果。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<string> fruits = {}; cout<<"The size of this forward_list is: "<<endl; cout<<distance(fruits.begin(), fruits.end())<<endl; cout<<"The iterator of all the elements are: "<<endl; //using the cbegin() function auto it = fruits.cbegin(); cout<<*it<<endl; }
输出
让我们编译并运行上述程序,这将产生以下结果:
The size of this forward_list is: 0 The iterator of all the elements are: Segmentation fault